mirror of
https://github.com/facebook/lexical.git
synced 2025-05-17 23:26:16 +08:00
Add more keyboard navigation tests (#307)
* Add more keyboard navigation tests * Update offsets given changes on main Co-authored-by: Dominic Gannaway <dg@domgan.com>
This commit is contained in:
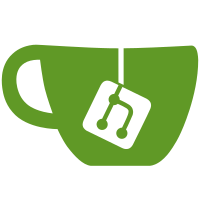
committed by
acywatson
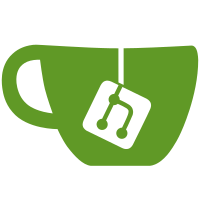
parent
af4f9f6ad7
commit
0a79385c23
@ -5,6 +5,10 @@
|
||||
* LICENSE file in the root directory of this source tree.
|
||||
*
|
||||
*/
|
||||
import {
|
||||
keyDownCtrlOrMeta,
|
||||
keyUpCtrlOrMeta,
|
||||
} from '../utils';
|
||||
|
||||
import {
|
||||
moveToEditorBeginning,
|
||||
@ -150,5 +154,314 @@ describe('Keyboard Navigation', () => {
|
||||
focusOffset: [65, 74],
|
||||
});
|
||||
});
|
||||
|
||||
it('can navigate through the plain text word by word', async () => {
|
||||
const {page} = e2e;
|
||||
await page.focus('div.editor');
|
||||
// type sample text
|
||||
await page.keyboard.type(' 123 abc 456 def ');
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 0, 0],
|
||||
anchorOffset: 20,
|
||||
focusPath: [0, 0, 0],
|
||||
focusOffset: 20,
|
||||
});
|
||||
// navigate through the text
|
||||
// 1 left
|
||||
await moveToPrevWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 0, 0],
|
||||
anchorOffset: 15,
|
||||
focusPath: [0, 0, 0],
|
||||
focusOffset: 15,
|
||||
});
|
||||
// 2 left
|
||||
await moveToPrevWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 0, 0],
|
||||
anchorOffset: 10,
|
||||
focusPath: [0, 0, 0],
|
||||
focusOffset: 10,
|
||||
});
|
||||
// 3 left
|
||||
await moveToPrevWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 0, 0],
|
||||
anchorOffset: 6,
|
||||
focusPath: [0, 0, 0],
|
||||
focusOffset: 6,
|
||||
});
|
||||
// 4 left
|
||||
await moveToPrevWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 0, 0],
|
||||
anchorOffset: 2,
|
||||
focusPath: [0, 0, 0],
|
||||
focusOffset: 2,
|
||||
});
|
||||
// 5 left
|
||||
await moveToPrevWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 0, 0],
|
||||
anchorOffset: 0,
|
||||
focusPath: [0, 0, 0],
|
||||
focusOffset: 0,
|
||||
});
|
||||
// 1 right
|
||||
await moveToNextWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 0, 0],
|
||||
anchorOffset: 5,
|
||||
focusPath: [0, 0, 0],
|
||||
focusOffset: 5,
|
||||
});
|
||||
// 2 right
|
||||
await moveToNextWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 0, 0],
|
||||
anchorOffset: 9,
|
||||
focusPath: [0, 0, 0],
|
||||
focusOffset: 9,
|
||||
});
|
||||
// 3 right
|
||||
await moveToNextWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 0, 0],
|
||||
anchorOffset: 13,
|
||||
focusPath: [0, 0, 0],
|
||||
focusOffset: 13,
|
||||
});
|
||||
// 4 right
|
||||
await moveToNextWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 0, 0],
|
||||
anchorOffset: 18,
|
||||
focusPath: [0, 0, 0],
|
||||
focusOffset: 18,
|
||||
});
|
||||
// 5 right
|
||||
await moveToNextWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 0, 0],
|
||||
anchorOffset: 20,
|
||||
focusPath: [0, 0, 0],
|
||||
focusOffset: 20,
|
||||
});
|
||||
});
|
||||
|
||||
it('can navigate through the formatted text word by word', async () => {
|
||||
const {page} = e2e;
|
||||
await page.focus('div.editor');
|
||||
// type sample text
|
||||
await page.keyboard.type(' 123 abc 456 def ');
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 0, 0],
|
||||
anchorOffset: 20,
|
||||
focusPath: [0, 0, 0],
|
||||
focusOffset: 20,
|
||||
});
|
||||
// select "de" and make it bold
|
||||
await moveToPrevWord(page);
|
||||
await page.keyboard.down('Shift');
|
||||
await page.keyboard.press('ArrowRight');
|
||||
await page.keyboard.press('ArrowRight');
|
||||
await page.keyboard.up('Shift');
|
||||
await keyDownCtrlOrMeta(page);
|
||||
await page.keyboard.press('b');
|
||||
await keyUpCtrlOrMeta(page);
|
||||
// select "ab" and make it bold
|
||||
await moveToPrevWord(page);
|
||||
await moveToPrevWord(page);
|
||||
await moveToPrevWord(page);
|
||||
await page.keyboard.down('Shift');
|
||||
await page.keyboard.press('ArrowRight');
|
||||
await page.keyboard.press('ArrowRight');
|
||||
await page.keyboard.up('Shift');
|
||||
await keyDownCtrlOrMeta(page);
|
||||
await page.keyboard.press('b');
|
||||
await keyUpCtrlOrMeta(page);
|
||||
await moveToLineEnd(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 4, 0],
|
||||
anchorOffset: 3,
|
||||
focusPath: [0, 4, 0],
|
||||
focusOffset: 3,
|
||||
});
|
||||
// navigate through the text
|
||||
// 1 left
|
||||
await moveToPrevWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 2, 0],
|
||||
anchorOffset: 7,
|
||||
focusPath: [0, 2, 0],
|
||||
focusOffset: 7,
|
||||
});
|
||||
// 2 left
|
||||
await moveToPrevWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 2, 0],
|
||||
anchorOffset: 2,
|
||||
focusPath: [0, 2, 0],
|
||||
focusOffset: 2,
|
||||
});
|
||||
// 3 left
|
||||
await moveToPrevWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 0, 0],
|
||||
anchorOffset: 6,
|
||||
focusPath: [0, 0, 0],
|
||||
focusOffset: 6,
|
||||
});
|
||||
// 4 left
|
||||
await moveToPrevWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 0, 0],
|
||||
anchorOffset: 2,
|
||||
focusPath: [0, 0, 0],
|
||||
focusOffset: 2,
|
||||
});
|
||||
// 5 left
|
||||
await moveToPrevWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 0, 0],
|
||||
anchorOffset: 0,
|
||||
focusPath: [0, 0, 0],
|
||||
focusOffset: 0,
|
||||
});
|
||||
// 1 right
|
||||
await moveToNextWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 0, 0],
|
||||
anchorOffset: 5,
|
||||
focusPath: [0, 0, 0],
|
||||
focusOffset: 5,
|
||||
});
|
||||
// 2 right
|
||||
await moveToNextWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 2, 0],
|
||||
anchorOffset: 1,
|
||||
focusPath: [0, 2, 0],
|
||||
focusOffset: 1,
|
||||
});
|
||||
// 3 right
|
||||
await moveToNextWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 2, 0],
|
||||
anchorOffset: 5,
|
||||
focusPath: [0, 2, 0],
|
||||
focusOffset: 5,
|
||||
});
|
||||
// 4 right
|
||||
await moveToNextWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 4, 0],
|
||||
anchorOffset: 1,
|
||||
focusPath: [0, 4, 0],
|
||||
focusOffset: 1,
|
||||
});
|
||||
// 5 right
|
||||
await moveToNextWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 4, 0],
|
||||
anchorOffset: 3,
|
||||
focusPath: [0, 4, 0],
|
||||
focusOffset: 3,
|
||||
});
|
||||
});
|
||||
|
||||
it('can navigate through the text with emoji word by word', async () => {
|
||||
const {page} = e2e;
|
||||
await page.focus('div.editor');
|
||||
// type sample text
|
||||
await page.keyboard.type('123:)456 abc:):)de fg');
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 6, 0],
|
||||
anchorOffset: 5,
|
||||
focusPath: [0, 6, 0],
|
||||
focusOffset: 5,
|
||||
});
|
||||
// navigate through the text
|
||||
// 1 left
|
||||
await moveToPrevWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 6, 0],
|
||||
anchorOffset: 3,
|
||||
focusPath: [0, 6, 0],
|
||||
focusOffset: 3,
|
||||
});
|
||||
// 2 left
|
||||
await moveToPrevWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 6, 0],
|
||||
anchorOffset: 0,
|
||||
focusPath: [0, 6, 0],
|
||||
focusOffset: 0,
|
||||
});
|
||||
// 3 left
|
||||
await moveToPrevWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 2, 0],
|
||||
anchorOffset: 4,
|
||||
focusPath: [0, 2, 0],
|
||||
focusOffset: 4,
|
||||
});
|
||||
// 4 left
|
||||
await moveToPrevWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 2, 0],
|
||||
anchorOffset: 0,
|
||||
focusPath: [0, 2, 0],
|
||||
focusOffset: 0,
|
||||
});
|
||||
// 5 left
|
||||
await moveToPrevWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 0, 0],
|
||||
anchorOffset: 0,
|
||||
focusPath: [0, 0, 0],
|
||||
focusOffset: 0,
|
||||
});
|
||||
// 1 right
|
||||
await moveToNextWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 0, 0],
|
||||
anchorOffset: 3,
|
||||
focusPath: [0, 0, 0],
|
||||
focusOffset: 3,
|
||||
});
|
||||
// 2 right
|
||||
await moveToNextWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 2, 0],
|
||||
anchorOffset: 3,
|
||||
focusPath: [0, 2, 0],
|
||||
focusOffset: 3,
|
||||
});
|
||||
// 3 right
|
||||
await moveToNextWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 2, 0],
|
||||
anchorOffset: 7,
|
||||
focusPath: [0, 2, 0],
|
||||
focusOffset: 7,
|
||||
});
|
||||
// 4 right
|
||||
await moveToNextWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 6, 0],
|
||||
anchorOffset: 2,
|
||||
focusPath: [0, 6, 0],
|
||||
focusOffset: 2,
|
||||
});
|
||||
// 5 right
|
||||
await moveToNextWord(page);
|
||||
await assertSelection(page, {
|
||||
anchorPath: [0, 6, 0],
|
||||
anchorOffset: 5,
|
||||
focusPath: [0, 6, 0],
|
||||
focusOffset: 5,
|
||||
});
|
||||
});
|
||||
});
|
||||
});
|
||||
|
Reference in New Issue
Block a user