mirror of
https://github.com/vmiklos/plees-tracker.git
synced 2025-05-17 19:16:07 +08:00
Extract SleepActivityUITest from MainActivityUITest
Also add a UITestBase base class to avoid duplication.
This commit is contained in:
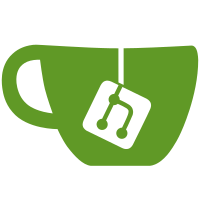
committed by
Miklos Vajna
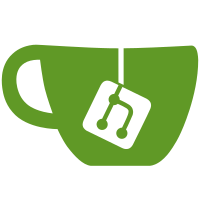
parent
969bd27572
commit
051ffdb3f4
@ -8,13 +8,7 @@ package hu.vmiklos.plees_tracker
|
||||
|
||||
import androidx.test.ext.junit.rules.ActivityScenarioRule
|
||||
import androidx.test.ext.junit.runners.AndroidJUnit4
|
||||
import androidx.test.platform.app.InstrumentationRegistry
|
||||
import androidx.test.uiautomator.By
|
||||
import androidx.test.uiautomator.Direction
|
||||
import androidx.test.uiautomator.UiDevice
|
||||
import androidx.test.uiautomator.UiObject2
|
||||
import androidx.test.uiautomator.Until
|
||||
import org.junit.Assert.assertEquals
|
||||
import org.junit.Rule
|
||||
import org.junit.Test
|
||||
import org.junit.runner.RunWith
|
||||
@ -23,55 +17,10 @@ import org.junit.runner.RunWith
|
||||
* UI tests for MainActivity.
|
||||
*/
|
||||
@RunWith(AndroidJUnit4::class)
|
||||
class MainActivityUITest {
|
||||
class MainActivityUITest : UITestBase() {
|
||||
@JvmField
|
||||
@Rule
|
||||
var activityScenarioRule = ActivityScenarioRule(MainActivity::class.java)
|
||||
private val timeout: Long = 5000
|
||||
val pkg = InstrumentationRegistry.getInstrumentation().processName
|
||||
|
||||
private fun resetDatabase() {
|
||||
val instrumentation = InstrumentationRegistry.getInstrumentation()
|
||||
val device = UiDevice.getInstance(instrumentation)
|
||||
device.pressMenu()
|
||||
val deleteAllSleep = device.wait(Until.findObject(By.text("Delete All Sleep")), timeout)
|
||||
deleteAllSleep.click()
|
||||
val yesButton = device.wait(Until.findObject(By.text("YES")), timeout)
|
||||
yesButton.click()
|
||||
}
|
||||
|
||||
private fun getDevice(): UiDevice {
|
||||
return UiDevice.getInstance(InstrumentationRegistry.getInstrumentation())
|
||||
}
|
||||
|
||||
private fun createSleep() {
|
||||
val device = getDevice()
|
||||
val startStop = device.wait(Until.findObject(By.res(pkg, "start_stop")), timeout)
|
||||
startStop.click()
|
||||
startStop.click()
|
||||
}
|
||||
|
||||
private fun assertResText(resourceId: String, textValue: String) {
|
||||
val device = getDevice()
|
||||
device.wait(Until.findObject(By.res(pkg, resourceId).text(textValue)), timeout)
|
||||
val obj = device.findObject(By.res(pkg, resourceId))
|
||||
assertEquals(textValue, obj.text)
|
||||
}
|
||||
|
||||
private fun findObjectByRes(resourceId: String): UiObject2 {
|
||||
val device = getDevice()
|
||||
return device.wait(Until.findObject(By.res(pkg, resourceId)), timeout)
|
||||
}
|
||||
|
||||
private fun findObjectByText(text: String): UiObject2 {
|
||||
val device = getDevice()
|
||||
return device.wait(Until.findObject(By.text(text)), timeout)
|
||||
}
|
||||
|
||||
private fun findObjectByDesc(desc: String): UiObject2 {
|
||||
val device = getDevice()
|
||||
return device.wait(Until.findObject(By.desc(desc)), timeout)
|
||||
}
|
||||
|
||||
@Test
|
||||
fun testCreateAndRead() {
|
||||
@ -98,27 +47,6 @@ class MainActivityUITest {
|
||||
// Then make sure we have no sleeps:
|
||||
assertResText("fragment_stats_sleeps", "0")
|
||||
}
|
||||
|
||||
@Test
|
||||
fun testUpdate() {
|
||||
resetDatabase()
|
||||
createSleep()
|
||||
|
||||
findObjectByRes("sleep_swipeable").click()
|
||||
findObjectByRes("sleep_start_time").click()
|
||||
findObjectByText("AM").click()
|
||||
findObjectByDesc("10").click()
|
||||
findObjectByDesc("0").click()
|
||||
findObjectByText("OK").click()
|
||||
findObjectByRes("sleep_stop_time").click()
|
||||
findObjectByText("PM").click()
|
||||
findObjectByDesc("10").click()
|
||||
findObjectByDesc("0").click()
|
||||
findObjectByText("OK").click()
|
||||
|
||||
assertResText("sleep_start_time", "10:00")
|
||||
assertResText("sleep_stop_time", "22:00")
|
||||
}
|
||||
}
|
||||
|
||||
/* vim:set shiftwidth=4 softtabstop=4 expandtab: */
|
||||
|
@ -0,0 +1,46 @@
|
||||
/*
|
||||
* Copyright 2024 Miklos Vajna
|
||||
*
|
||||
* SPDX-License-Identifier: MIT
|
||||
*/
|
||||
|
||||
package hu.vmiklos.plees_tracker
|
||||
|
||||
import androidx.test.ext.junit.rules.ActivityScenarioRule
|
||||
import androidx.test.ext.junit.runners.AndroidJUnit4
|
||||
import org.junit.Rule
|
||||
import org.junit.Test
|
||||
import org.junit.runner.RunWith
|
||||
|
||||
/**
|
||||
* UI tests for SleepActivity.
|
||||
*/
|
||||
@RunWith(AndroidJUnit4::class)
|
||||
class SleepActivityUITest : UITestBase() {
|
||||
@JvmField
|
||||
@Rule
|
||||
var activityScenarioRule = ActivityScenarioRule(MainActivity::class.java)
|
||||
|
||||
@Test
|
||||
fun testUpdate() {
|
||||
resetDatabase()
|
||||
createSleep()
|
||||
|
||||
findObjectByRes("sleep_swipeable").click()
|
||||
findObjectByRes("sleep_start_time").click()
|
||||
findObjectByText("AM").click()
|
||||
findObjectByDesc("10").click()
|
||||
findObjectByDesc("0").click()
|
||||
findObjectByText("OK").click()
|
||||
findObjectByRes("sleep_stop_time").click()
|
||||
findObjectByText("PM").click()
|
||||
findObjectByDesc("10").click()
|
||||
findObjectByDesc("0").click()
|
||||
findObjectByText("OK").click()
|
||||
|
||||
assertResText("sleep_start_time", "10:00")
|
||||
assertResText("sleep_stop_time", "22:00")
|
||||
}
|
||||
}
|
||||
|
||||
/* vim:set shiftwidth=4 softtabstop=4 expandtab: */
|
@ -0,0 +1,53 @@
|
||||
/*
|
||||
* Copyright 2024 Miklos Vajna
|
||||
*
|
||||
* SPDX-License-Identifier: MIT
|
||||
*/
|
||||
|
||||
package hu.vmiklos.plees_tracker
|
||||
|
||||
import androidx.test.platform.app.InstrumentationRegistry
|
||||
import androidx.test.uiautomator.By
|
||||
import androidx.test.uiautomator.UiDevice
|
||||
import androidx.test.uiautomator.UiObject2
|
||||
import androidx.test.uiautomator.Until
|
||||
import org.junit.Assert.assertEquals
|
||||
|
||||
open class UITestBase {
|
||||
protected val timeout: Long = 5000
|
||||
private val instrumentation = InstrumentationRegistry.getInstrumentation()
|
||||
protected val pkg = instrumentation.processName
|
||||
protected val device = UiDevice.getInstance(instrumentation)
|
||||
|
||||
protected fun findObjectByRes(resourceId: String): UiObject2 {
|
||||
return device.wait(Until.findObject(By.res(pkg, resourceId)), timeout)
|
||||
}
|
||||
|
||||
protected fun findObjectByText(text: String): UiObject2 {
|
||||
return device.wait(Until.findObject(By.text(text)), timeout)
|
||||
}
|
||||
|
||||
protected fun findObjectByDesc(desc: String): UiObject2 {
|
||||
return device.wait(Until.findObject(By.desc(desc)), timeout)
|
||||
}
|
||||
|
||||
protected fun assertResText(resourceId: String, textValue: String) {
|
||||
device.wait(Until.findObject(By.res(pkg, resourceId).text(textValue)), timeout)
|
||||
val obj = device.findObject(By.res(pkg, resourceId))
|
||||
assertEquals(textValue, obj.text)
|
||||
}
|
||||
|
||||
protected fun resetDatabase() {
|
||||
device.pressMenu()
|
||||
findObjectByText("Delete All Sleep").click()
|
||||
findObjectByText("YES").click()
|
||||
}
|
||||
|
||||
protected fun createSleep() {
|
||||
val startStop = findObjectByRes("start_stop")
|
||||
startStop.click()
|
||||
startStop.click()
|
||||
}
|
||||
}
|
||||
|
||||
/* vim:set shiftwidth=4 softtabstop=4 expandtab: */
|
Reference in New Issue
Block a user