mirror of
https://github.com/containers/podman.git
synced 2025-05-22 01:27:07 +08:00
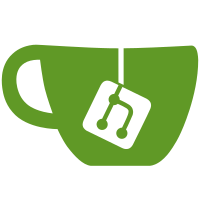
Pull the trigger on the `pkg/registries` package which acted as a proxy for `c/image/pkg/sysregistriesv2`. Callers should be using the packages from c/image directly, if needed at all. Also make use of libimage's SystemContext() method which returns a copy of a system context, further reducing the risk of unintentionally altering global data. [NO TESTS NEEDED] Signed-off-by: Valentin Rothberg <rothberg@redhat.com>
58 lines
1.9 KiB
Go
58 lines
1.9 KiB
Go
package compat
|
|
|
|
import (
|
|
"context"
|
|
"encoding/json"
|
|
"fmt"
|
|
"net/http"
|
|
"strings"
|
|
|
|
DockerClient "github.com/containers/image/v5/docker"
|
|
"github.com/containers/image/v5/types"
|
|
"github.com/containers/podman/v3/libpod"
|
|
"github.com/containers/podman/v3/pkg/api/handlers/utils"
|
|
"github.com/containers/podman/v3/pkg/domain/entities"
|
|
docker "github.com/docker/docker/api/types"
|
|
"github.com/pkg/errors"
|
|
)
|
|
|
|
func stripAddressOfScheme(address string) string {
|
|
for _, s := range []string{"https", "http"} {
|
|
address = strings.TrimPrefix(address, s+"://")
|
|
}
|
|
return address
|
|
}
|
|
|
|
func Auth(w http.ResponseWriter, r *http.Request) {
|
|
var authConfig docker.AuthConfig
|
|
err := json.NewDecoder(r.Body).Decode(&authConfig)
|
|
if err != nil {
|
|
utils.Error(w, "Something went wrong.", http.StatusInternalServerError, errors.Wrapf(err, "failed to parse request"))
|
|
return
|
|
}
|
|
|
|
skipTLS := types.NewOptionalBool(false)
|
|
if strings.HasPrefix(authConfig.ServerAddress, "https://localhost/") || strings.HasPrefix(authConfig.ServerAddress, "https://localhost:") || strings.HasPrefix(authConfig.ServerAddress, "localhost:") {
|
|
// support for local testing
|
|
skipTLS = types.NewOptionalBool(true)
|
|
}
|
|
|
|
runtime := r.Context().Value("runtime").(*libpod.Runtime)
|
|
sysCtx := runtime.SystemContext()
|
|
sysCtx.DockerInsecureSkipTLSVerify = skipTLS
|
|
|
|
fmt.Println("Authenticating with existing credentials...")
|
|
registry := stripAddressOfScheme(authConfig.ServerAddress)
|
|
if err := DockerClient.CheckAuth(context.Background(), sysCtx, authConfig.Username, authConfig.Password, registry); err == nil {
|
|
utils.WriteResponse(w, http.StatusOK, entities.AuthReport{
|
|
IdentityToken: "",
|
|
Status: "Login Succeeded",
|
|
})
|
|
} else {
|
|
utils.WriteResponse(w, http.StatusBadRequest, entities.AuthReport{
|
|
IdentityToken: "",
|
|
Status: "login attempt to " + authConfig.ServerAddress + " failed with status: " + err.Error(),
|
|
})
|
|
}
|
|
}
|