mirror of
https://github.com/containers/podman.git
synced 2025-05-20 08:36:23 +08:00
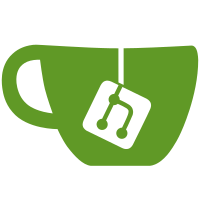
In Docker anything but "", "0", "no", "false", "none" (ignoring case) is considered to be true. Signed-off-by: Matej Vasek <mvasek@redhat.com>
64 lines
1.7 KiB
Go
64 lines
1.7 KiB
Go
package compat
|
|
|
|
import (
|
|
"errors"
|
|
"fmt"
|
|
"net/http"
|
|
|
|
"github.com/containers/podman/v4/libpod"
|
|
"github.com/containers/podman/v4/libpod/define"
|
|
"github.com/containers/podman/v4/pkg/api/handlers/utils"
|
|
api "github.com/containers/podman/v4/pkg/api/types"
|
|
"github.com/containers/podman/v4/pkg/domain/entities"
|
|
"github.com/containers/podman/v4/pkg/domain/infra/abi"
|
|
)
|
|
|
|
func RestartContainer(w http.ResponseWriter, r *http.Request) {
|
|
runtime := r.Context().Value(api.RuntimeKey).(*libpod.Runtime)
|
|
decoder := utils.GetDecoder(r)
|
|
// Now use the ABI implementation to prevent us from having duplicate
|
|
// code.
|
|
containerEngine := abi.ContainerEngine{Libpod: runtime}
|
|
|
|
// /{version}/containers/(name)/restart
|
|
query := struct {
|
|
All bool `schema:"all"`
|
|
DockerTimeout uint `schema:"t"`
|
|
LibpodTimeout uint `schema:"timeout"`
|
|
}{
|
|
// override any golang type defaults
|
|
}
|
|
if err := decoder.Decode(&query, r.URL.Query()); err != nil {
|
|
utils.Error(w, http.StatusBadRequest, fmt.Errorf("failed to parse parameters for %s: %w", r.URL.String(), err))
|
|
return
|
|
}
|
|
|
|
name := utils.GetName(r)
|
|
|
|
options := entities.RestartOptions{
|
|
All: query.All,
|
|
Timeout: &query.DockerTimeout,
|
|
}
|
|
if utils.IsLibpodRequest(r) {
|
|
options.Timeout = &query.LibpodTimeout
|
|
}
|
|
report, err := containerEngine.ContainerRestart(r.Context(), []string{name}, options)
|
|
if err != nil {
|
|
if errors.Is(err, define.ErrNoSuchCtr) {
|
|
utils.ContainerNotFound(w, name, err)
|
|
return
|
|
}
|
|
|
|
utils.InternalServerError(w, err)
|
|
return
|
|
}
|
|
|
|
if len(report) > 0 && report[0].Err != nil {
|
|
utils.InternalServerError(w, report[0].Err)
|
|
return
|
|
}
|
|
|
|
// Success
|
|
utils.WriteResponse(w, http.StatusNoContent, nil)
|
|
}
|