mirror of
https://github.com/containers/podman.git
synced 2025-05-21 00:56:36 +08:00
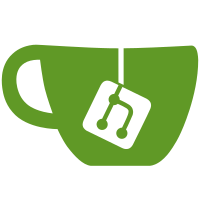
Rename all files to _test.go and rename the package to e2e_test. This makes the linter less strict about things like dot imports. Add some unused nolint directives to silence some warnings, these can be used to find untested options so someone could add tests for them. Fixes #14996 Signed-off-by: Paul Holzinger <pholzing@redhat.com>
68 lines
2.0 KiB
Go
68 lines
2.0 KiB
Go
package e2e_test
|
|
|
|
import (
|
|
. "github.com/onsi/ginkgo"
|
|
. "github.com/onsi/gomega"
|
|
. "github.com/onsi/gomega/gexec"
|
|
)
|
|
|
|
var _ = Describe("podman machine ssh", func() {
|
|
var (
|
|
mb *machineTestBuilder
|
|
testDir string
|
|
)
|
|
|
|
BeforeEach(func() {
|
|
testDir, mb = setup()
|
|
})
|
|
AfterEach(func() {
|
|
teardown(originalHomeDir, testDir, mb)
|
|
})
|
|
|
|
It("bad machine name", func() {
|
|
name := randomString()
|
|
ssh := sshMachine{}
|
|
session, err := mb.setName(name).setCmd(ssh).run()
|
|
Expect(err).To(BeNil())
|
|
Expect(session).To(Exit(125))
|
|
// TODO seems like stderr is not being returned; re-enabled when fixed
|
|
// Expect(session.outputToString()).To(ContainSubstring("not exist"))
|
|
})
|
|
|
|
It("ssh to non-running machine", func() {
|
|
name := randomString()
|
|
i := new(initMachine)
|
|
session, err := mb.setName(name).setCmd(i.withImagePath(mb.imagePath)).run()
|
|
Expect(err).To(BeNil())
|
|
Expect(session).To(Exit(0))
|
|
|
|
ssh := sshMachine{}
|
|
sshSession, err := mb.setName(name).setCmd(ssh).run()
|
|
Expect(err).To(BeNil())
|
|
// TODO seems like stderr is not being returned; re-enabled when fixed
|
|
// Expect(sshSession.outputToString()).To(ContainSubstring("is not running"))
|
|
Expect(sshSession).To(Exit(125))
|
|
})
|
|
|
|
It("ssh to running machine and check os-type", func() {
|
|
name := randomString()
|
|
i := new(initMachine)
|
|
session, err := mb.setName(name).setCmd(i.withImagePath(mb.imagePath).withNow()).run()
|
|
Expect(err).To(BeNil())
|
|
Expect(session).To(Exit(0))
|
|
|
|
ssh := sshMachine{}
|
|
sshSession, err := mb.setName(name).setCmd(ssh.withSSHComand([]string{"cat", "/etc/os-release"})).run()
|
|
Expect(err).To(BeNil())
|
|
Expect(sshSession).To(Exit(0))
|
|
Expect(sshSession.outputToString()).To(ContainSubstring("Fedora CoreOS"))
|
|
|
|
// keep exit code
|
|
sshSession, err = mb.setName(name).setCmd(ssh.withSSHComand([]string{"false"})).run()
|
|
Expect(err).To(BeNil())
|
|
Expect(sshSession).To(Exit(1))
|
|
Expect(sshSession.outputToString()).To(Equal(""))
|
|
Expect(sshSession.errorToString()).To(Equal(""))
|
|
})
|
|
})
|