mirror of
https://github.com/containers/podman.git
synced 2025-05-22 09:36:57 +08:00
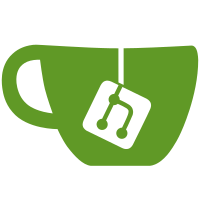
SpecGen is our primary container creation abstraction, and is used to connect our CLI to the Libpod container creation backend. Because container creation has a million options (I exaggerate only slightly), the struct is composed of several other structs, many of which are quite large. The core problem is that SpecGen is also an API type - it's used in remote Podman. There, we have a client and a server, and we want to respect the server's containers.conf. But how do we tell what parts of SpecGen were set by the client explicitly, and what parts were not? If we're not using nullable values, an explicit empty string and a value never being set are identical - and we can't tell if it's safe to grab a default from the server's containers.conf. Fortunately, we only really need to do this for booleans. An empty string is sufficient to tell us that a string was unset (even if the user explicitly gave us an empty string for an option, filling in a default from the config file is acceptable). This makes things a lot simpler. My initial attempt at this changed everything, including strings, and it was far larger and more painful. Also, begin the first steps of removing all uses of containers.conf defaults from client-side. Two are gone entirely, the rest are marked as remove-when-possible. [NO NEW TESTS NEEDED] This is just a refactor. Signed-off-by: Matt Heon <mheon@redhat.com>
39 lines
1.0 KiB
Go
39 lines
1.0 KiB
Go
package specgen
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/stretchr/testify/assert"
|
|
)
|
|
|
|
func TestNewSpecGeneratorWithRootfs(t *testing.T) {
|
|
idmap := "idmap"
|
|
idmapMappings := "idmap=uids=1-1-2000"
|
|
localTrue := true
|
|
tests := []struct {
|
|
rootfs string
|
|
expectedRootfsOverlay *bool
|
|
expectedRootfs string
|
|
expectedMapping *string
|
|
}{
|
|
{"/root/a:b:O", &localTrue, "/root/a:b", nil},
|
|
{"/root/a:b/c:O", &localTrue, "/root/a:b/c", nil},
|
|
{"/root/a:b/c:", nil, "/root/a:b/c:", nil},
|
|
{"/root/a/b", nil, "/root/a/b", nil},
|
|
{"/root/a:b/c:idmap", nil, "/root/a:b/c", &idmap},
|
|
{"/root/a:b/c:idmap=uids=1-1-2000", nil, "/root/a:b/c", &idmapMappings},
|
|
}
|
|
for _, args := range tests {
|
|
val := NewSpecGenerator(args.rootfs, true)
|
|
|
|
assert.Equal(t, val.RootfsOverlay, args.expectedRootfsOverlay)
|
|
assert.Equal(t, val.Rootfs, args.expectedRootfs)
|
|
if args.expectedMapping == nil {
|
|
assert.Nil(t, val.RootfsMapping)
|
|
} else {
|
|
assert.NotNil(t, val.RootfsMapping)
|
|
assert.Equal(t, *val.RootfsMapping, *args.expectedMapping)
|
|
}
|
|
}
|
|
}
|