mirror of
https://github.com/containers/podman.git
synced 2025-05-20 08:36:23 +08:00
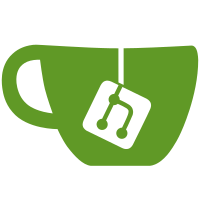
Cache cleanups only happen if there is a cache miss, and we need to pull a new image For quay.io/podman/machine-os, we remove all old images from the cache dir. This means we will delete any file that exists in the cache dir; this should be safe to do since the machine pull code should be the only thing touching this cache dir. OCI machine images will always have a different manifest, and won’t be updated with the same manifest, so if the version moves on, there isn’t a reason to keep the old version in the cache, it really doesn’t change. For Fedora (WSL), we use the cache, so we go through the cache dir and remove any old cached images, on a cache miss. We also switch to using ~/.local/share/containers/podman/machine/wsl/cache as the cache dir rather than ~/.local/share/containers/podman/machine/wsl. Both these behaviors existed in v4.9, but are now added back into 5.x. For generic files pulled from a URL or a non-default OCI image, we shouldn’t actually cache, so we delete the pulled file immediately after creating a machine image. This restores the behavior from v4.9. For generic files from a local path, the original file will never be cleaned up Unsure how to test, so: [NO NEW TESTS NEEDED] Signed-off-by: Ashley Cui <acui@redhat.com>
44 lines
1.2 KiB
Go
44 lines
1.2 KiB
Go
package e2e_test
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
"path/filepath"
|
|
"runtime"
|
|
"strings"
|
|
|
|
"github.com/containers/podman/v5/pkg/machine/compression"
|
|
"github.com/containers/podman/v5/pkg/machine/define"
|
|
"github.com/containers/podman/v5/pkg/machine/ocipull"
|
|
)
|
|
|
|
func pullOCITestDisk(finalDir string, vmType define.VMType) error {
|
|
imageCacheDir, err := define.NewMachineFile(finalDir, nil)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
unusedFinalPath, err := imageCacheDir.AppendToNewVMFile(fmt.Sprintf("machinetest-%s", runtime.GOOS), nil)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
dirs := define.MachineDirs{ImageCacheDir: imageCacheDir}
|
|
ociArtPull, err := ocipull.NewOCIArtifactPull(context.Background(), &dirs, "", "e2emachine", vmType, unusedFinalPath)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
_, err = ociArtPull.GetNoCompress()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
fp, originalName := ociArtPull.OriginalFileName()
|
|
// Rename the download to something we recognize
|
|
compressionExt := filepath.Ext(fp)
|
|
fqImageName = filepath.Join(tmpDir, strings.TrimSuffix(originalName, compressionExt))
|
|
suiteImageName = filepath.Base(fqImageName)
|
|
compressedImage, err := define.NewMachineFile(fp, nil)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
return compression.Decompress(compressedImage, fqImageName)
|
|
}
|