mirror of
https://github.com/containers/podman.git
synced 2025-05-20 16:47:39 +08:00
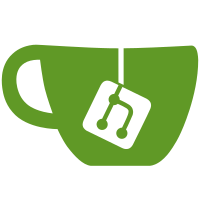
Instead switch to github.com/moby/sys/user, which we already had as an indirect dependency. Signed-off-by: Matt Heon <mheon@redhat.com>
42 lines
1.3 KiB
Go
42 lines
1.3 KiB
Go
//go:build !remote
|
|
|
|
package libpod
|
|
|
|
import (
|
|
"github.com/containers/common/pkg/capabilities"
|
|
"github.com/moby/sys/user"
|
|
spec "github.com/opencontainers/runtime-spec/specs-go"
|
|
)
|
|
|
|
func (c *Container) setProcessCapabilitiesExec(options *ExecOptions, user string, execUser *user.ExecUser, pspec *spec.Process) error {
|
|
ctrSpec, err := c.specFromState()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
allCaps, err := capabilities.BoundingSet()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if options.Privileged {
|
|
pspec.Capabilities.Bounding = allCaps
|
|
} else {
|
|
pspec.Capabilities.Bounding = ctrSpec.Process.Capabilities.Bounding
|
|
}
|
|
|
|
// Always unset the inheritable capabilities similarly to what the Linux kernel does
|
|
// They are used only when using capabilities with uid != 0.
|
|
pspec.Capabilities.Inheritable = []string{}
|
|
|
|
if execUser.Uid == 0 {
|
|
pspec.Capabilities.Effective = pspec.Capabilities.Bounding
|
|
pspec.Capabilities.Permitted = pspec.Capabilities.Bounding
|
|
} else if user == c.config.User {
|
|
pspec.Capabilities.Effective = ctrSpec.Process.Capabilities.Effective
|
|
pspec.Capabilities.Inheritable = ctrSpec.Process.Capabilities.Effective
|
|
pspec.Capabilities.Permitted = ctrSpec.Process.Capabilities.Effective
|
|
pspec.Capabilities.Ambient = ctrSpec.Process.Capabilities.Effective
|
|
}
|
|
return nil
|
|
}
|