mirror of
https://github.com/containers/podman.git
synced 2025-05-20 00:27:03 +08:00
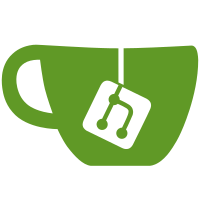
* To aid in debugging log API request and response bodies at trace level. Events can be correlated using the X-Reference-Id. * Server now echos X-Reference-Id from client if set, otherwise generates an unique id. * Move logic for X-Reference-Id into middleware * Change uses of Header.Add() to Set() when setting Content-Type * Log API operations in Apache format using gorilla middleware * Port server code to use BaseContext and ConnContext Fixes #10053 Signed-off-by: Jhon Honce <jhonce@redhat.com>
52 lines
1.4 KiB
Go
52 lines
1.4 KiB
Go
package compat
|
|
|
|
import (
|
|
"net/http"
|
|
|
|
api "github.com/containers/podman/v3/pkg/api/types"
|
|
"github.com/sirupsen/logrus"
|
|
|
|
"github.com/containers/podman/v3/libpod"
|
|
"github.com/containers/podman/v3/libpod/define"
|
|
"github.com/containers/podman/v3/pkg/api/handlers/utils"
|
|
"github.com/gorilla/schema"
|
|
)
|
|
|
|
func StartContainer(w http.ResponseWriter, r *http.Request) {
|
|
decoder := r.Context().Value(api.DecoderKey).(*schema.Decoder)
|
|
query := struct {
|
|
DetachKeys string `schema:"detachKeys"`
|
|
}{
|
|
// Override golang default values for types
|
|
}
|
|
if err := decoder.Decode(&query, r.URL.Query()); err != nil {
|
|
utils.BadRequest(w, "url", r.URL.String(), err)
|
|
return
|
|
}
|
|
if len(query.DetachKeys) > 0 {
|
|
// TODO - start does not support adding detach keys
|
|
logrus.Info("the detach keys parameter is not supported on start container")
|
|
}
|
|
runtime := r.Context().Value(api.RuntimeKey).(*libpod.Runtime)
|
|
name := utils.GetName(r)
|
|
con, err := runtime.LookupContainer(name)
|
|
if err != nil {
|
|
utils.ContainerNotFound(w, name, err)
|
|
return
|
|
}
|
|
state, err := con.State()
|
|
if err != nil {
|
|
utils.InternalServerError(w, err)
|
|
return
|
|
}
|
|
if state == define.ContainerStateRunning {
|
|
utils.WriteResponse(w, http.StatusNotModified, nil)
|
|
return
|
|
}
|
|
if err := con.Start(r.Context(), true); err != nil {
|
|
utils.InternalServerError(w, err)
|
|
return
|
|
}
|
|
utils.WriteResponse(w, http.StatusNoContent, nil)
|
|
}
|