mirror of
https://github.com/containers/podman.git
synced 2025-05-22 01:27:07 +08:00
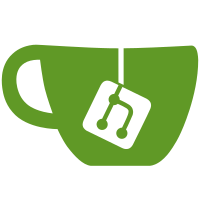
Install ginkgo on demand via `go get -u` rather than keeping a copy it's entire source code in the vendor dirctory. The main motivation for that is to make `golangci-lint` happy which is continuously throwing up on the import of a program (i.e., ginkgo). The linter is broken and stupid as it ignores flags to ignore dirs and ignores build tags (at least some linters do) which is blocking us from updating to newer versions. Signed-off-by: Valentin Rothberg <rothberg@redhat.com>
78 lines
1.8 KiB
Go
78 lines
1.8 KiB
Go
// Copyright 2009 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// illumos system calls not present on Solaris.
|
|
|
|
// +build amd64,illumos
|
|
|
|
package unix
|
|
|
|
import "unsafe"
|
|
|
|
func bytes2iovec(bs [][]byte) []Iovec {
|
|
iovecs := make([]Iovec, len(bs))
|
|
for i, b := range bs {
|
|
iovecs[i].SetLen(len(b))
|
|
if len(b) > 0 {
|
|
// somehow Iovec.Base on illumos is (*int8), not (*byte)
|
|
iovecs[i].Base = (*int8)(unsafe.Pointer(&b[0]))
|
|
} else {
|
|
iovecs[i].Base = (*int8)(unsafe.Pointer(&_zero))
|
|
}
|
|
}
|
|
return iovecs
|
|
}
|
|
|
|
//sys readv(fd int, iovs []Iovec) (n int, err error)
|
|
|
|
func Readv(fd int, iovs [][]byte) (n int, err error) {
|
|
iovecs := bytes2iovec(iovs)
|
|
n, err = readv(fd, iovecs)
|
|
return n, err
|
|
}
|
|
|
|
//sys preadv(fd int, iovs []Iovec, off int64) (n int, err error)
|
|
|
|
func Preadv(fd int, iovs [][]byte, off int64) (n int, err error) {
|
|
iovecs := bytes2iovec(iovs)
|
|
n, err = preadv(fd, iovecs, off)
|
|
return n, err
|
|
}
|
|
|
|
//sys writev(fd int, iovs []Iovec) (n int, err error)
|
|
|
|
func Writev(fd int, iovs [][]byte) (n int, err error) {
|
|
iovecs := bytes2iovec(iovs)
|
|
n, err = writev(fd, iovecs)
|
|
return n, err
|
|
}
|
|
|
|
//sys pwritev(fd int, iovs []Iovec, off int64) (n int, err error)
|
|
|
|
func Pwritev(fd int, iovs [][]byte, off int64) (n int, err error) {
|
|
iovecs := bytes2iovec(iovs)
|
|
n, err = pwritev(fd, iovecs, off)
|
|
return n, err
|
|
}
|
|
|
|
//sys accept4(s int, rsa *RawSockaddrAny, addrlen *_Socklen, flags int) (fd int, err error) = libsocket.accept4
|
|
|
|
func Accept4(fd int, flags int) (nfd int, sa Sockaddr, err error) {
|
|
var rsa RawSockaddrAny
|
|
var len _Socklen = SizeofSockaddrAny
|
|
nfd, err = accept4(fd, &rsa, &len, flags)
|
|
if err != nil {
|
|
return
|
|
}
|
|
if len > SizeofSockaddrAny {
|
|
panic("RawSockaddrAny too small")
|
|
}
|
|
sa, err = anyToSockaddr(fd, &rsa)
|
|
if err != nil {
|
|
Close(nfd)
|
|
nfd = 0
|
|
}
|
|
return
|
|
}
|