mirror of
https://github.com/containers/podman.git
synced 2025-05-20 00:27:03 +08:00
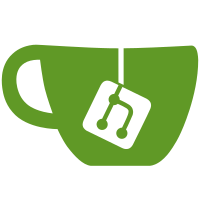
consumers of the api remarked how they would prefer a more strongly typed data structure from list containers oon the libpod side of things. for example, events should be consumable and consistent timestamps. also, for the sake of compatibility, it is helpful to have the json named atttributes for Id to not be ID. listcontainers on the libpod side no longer strongly uses the the ps cli to obtain information but we do benefit from turning on the ability to list the last X containers, something CLI does not have yet. we also flipped the bit on defaulting to truncated output in the return. thanks to the efforts of the cockpit team to help us here. Signed-off-by: Brent Baude <bbaude@redhat.com>
139 lines
2.6 KiB
Go
139 lines
2.6 KiB
Go
package handlers
|
|
|
|
import (
|
|
"github.com/containers/libpod/libpod"
|
|
"github.com/containers/libpod/libpod/image"
|
|
"github.com/containers/libpod/pkg/inspect"
|
|
"github.com/docker/docker/api/types"
|
|
)
|
|
|
|
// History response
|
|
// swagger:response DocsHistory
|
|
type swagHistory struct {
|
|
// in:body
|
|
Body struct {
|
|
HistoryResponse
|
|
}
|
|
}
|
|
|
|
// Inspect response
|
|
// swagger:response DocsImageInspect
|
|
type swagImageInspect struct {
|
|
// in:body
|
|
Body struct {
|
|
ImageInspect
|
|
}
|
|
}
|
|
|
|
// Load response
|
|
// swagger:response DocsLibpodImagesLoadResponse
|
|
type swagLibpodImagesLoadResponse struct {
|
|
// in:body
|
|
Body []LibpodImagesLoadReport
|
|
}
|
|
|
|
// Import response
|
|
// swagger:response DocsLibpodImagesImportResponse
|
|
type swagLibpodImagesImportResponse struct {
|
|
// in:body
|
|
Body LibpodImagesImportReport
|
|
}
|
|
|
|
// Pull response
|
|
// swagger:response DocsLibpodImagesPullResponse
|
|
type swagLibpodImagesPullResponse struct {
|
|
// in:body
|
|
Body LibpodImagesPullReport
|
|
}
|
|
|
|
// Delete response
|
|
// swagger:response DocsImageDeleteResponse
|
|
type swagImageDeleteResponse struct {
|
|
// in:body
|
|
Body []image.ImageDeleteResponse
|
|
}
|
|
|
|
// Search results
|
|
// swagger:response DocsSearchResponse
|
|
type swagSearchResponse struct {
|
|
// in:body
|
|
Body struct {
|
|
image.SearchResult
|
|
}
|
|
}
|
|
|
|
// Inspect image
|
|
// swagger:response DocsLibpodInspectImageResponse
|
|
type swagLibpodInspectImageResponse struct {
|
|
// in:body
|
|
Body struct {
|
|
inspect.ImageData
|
|
}
|
|
}
|
|
|
|
// Prune containers
|
|
// swagger:response DocsContainerPruneReport
|
|
type swagContainerPruneReport struct {
|
|
// in: body
|
|
Body []ContainersPruneReport
|
|
}
|
|
|
|
// Prune containers
|
|
// swagger:response DocsLibpodPruneResponse
|
|
type swagLibpodContainerPruneReport struct {
|
|
// in: body
|
|
Body []LibpodContainersPruneReport
|
|
}
|
|
|
|
// Inspect container
|
|
// swagger:response DocsContainerInspectResponse
|
|
type swagContainerInspectResponse struct {
|
|
// in:body
|
|
Body struct {
|
|
types.ContainerJSON
|
|
}
|
|
}
|
|
|
|
// List processes in container
|
|
// swagger:response DockerTopResponse
|
|
type swagDockerTopResponse struct {
|
|
// in:body
|
|
Body struct {
|
|
ContainerTopOKBody
|
|
}
|
|
}
|
|
|
|
// Inspect container
|
|
// swagger:response LibpodInspectContainerResponse
|
|
type swagLibpodInspectContainerResponse struct {
|
|
// in:body
|
|
Body struct {
|
|
libpod.InspectContainerData
|
|
}
|
|
}
|
|
|
|
// List pods
|
|
// swagger:response ListPodsResponse
|
|
type swagListPodsResponse struct {
|
|
// in:body
|
|
Body []libpod.PodInspect
|
|
}
|
|
|
|
// Inspect pod
|
|
// swagger:response InspectPodResponse
|
|
type swagInspectPodResponse struct {
|
|
// in:body
|
|
Body struct {
|
|
libpod.PodInspect
|
|
}
|
|
}
|
|
|
|
// Inspect volume
|
|
// swagger:response InspectVolumeResponse
|
|
type swagInspectVolumeResponse struct {
|
|
// in:body
|
|
Body struct {
|
|
libpod.InspectVolumeData
|
|
}
|
|
}
|