mirror of
https://github.com/containers/podman.git
synced 2025-07-02 00:30:00 +08:00
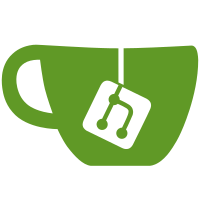
The ReadOnly and the RunInit keys affect options that have a variable default (configurable in containers.conf). This means we need to handle them a bit differently in quadlet to allow overriding the default. For example, we can't assume ReadOnly=false doesn't need to add any argument because no argument may mean readonly=true if the default is changed. We now don't add any argument (leaving the default) if the key is not specified, or we always add an argument (--foo or --foo=false) if the key is specified (overriding whatever the default is). Signed-off-by: Alexander Larsson <alexl@redhat.com>
77 lines
1.3 KiB
Go
77 lines
1.3 KiB
Go
package quadlet
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"path"
|
|
"sort"
|
|
)
|
|
|
|
// Overwritten at build time
|
|
var (
|
|
_binDir = "/usr/bin"
|
|
)
|
|
|
|
func podmanBinary() string {
|
|
podman := os.Getenv("PODMAN")
|
|
if len(podman) > 0 {
|
|
return podman
|
|
}
|
|
return path.Join(_binDir, "podman")
|
|
}
|
|
|
|
/* This is a helper for constructing podman commandlines */
|
|
type PodmanCmdline struct {
|
|
Args []string
|
|
}
|
|
|
|
func (c *PodmanCmdline) add(args ...string) {
|
|
c.Args = append(c.Args, args...)
|
|
}
|
|
|
|
func (c *PodmanCmdline) addf(format string, a ...interface{}) {
|
|
c.add(fmt.Sprintf(format, a...))
|
|
}
|
|
|
|
func (c *PodmanCmdline) addKeys(arg string, keys map[string]string) {
|
|
ks := make([]string, 0, len(keys))
|
|
for k := range keys {
|
|
ks = append(ks, k)
|
|
}
|
|
sort.Strings(ks)
|
|
|
|
for _, k := range ks {
|
|
c.add(arg, fmt.Sprintf("%s=%s", k, keys[k]))
|
|
}
|
|
}
|
|
|
|
func (c *PodmanCmdline) addEnv(env map[string]string) {
|
|
c.addKeys("--env", env)
|
|
}
|
|
|
|
func (c *PodmanCmdline) addLabels(labels map[string]string) {
|
|
c.addKeys("--label", labels)
|
|
}
|
|
|
|
func (c *PodmanCmdline) addAnnotations(annotations map[string]string) {
|
|
c.addKeys("--annotation", annotations)
|
|
}
|
|
|
|
func (c *PodmanCmdline) addBool(arg string, val bool) {
|
|
if val {
|
|
c.add(arg)
|
|
} else {
|
|
c.addf("%s=false", arg)
|
|
}
|
|
}
|
|
|
|
func NewPodmanCmdline(args ...string) *PodmanCmdline {
|
|
c := &PodmanCmdline{
|
|
Args: make([]string, 0),
|
|
}
|
|
|
|
c.add(podmanBinary())
|
|
c.add(args...)
|
|
return c
|
|
}
|