mirror of
https://github.com/containers/podman.git
synced 2025-05-28 13:40:33 +08:00
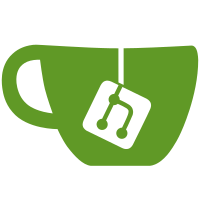
Note: this commit is merely adding swagger documentation and the golang stubs and types for the proposed endpoints. The implementation will follow in separate individual changes in the future. The ultimate goal is to prevent the libpod API from exposing the rather complex /images/create endpoint from Docker and split it into easier to implement, use and comprehend endpoints with a more narrow focus. # Import Add the v2 swagger documentation for the libpod/images/import endpoint. Note that we have intend to have separate backend and not mix it up with load since import allows for specifying a URL instead of a local tarball. # Load Complete the v2 swagger documentation for the libpod/images/load endpoint. Note that we are accounting for future plans to be able to load multiple images from one oci/docker archive by returning an array of image-load responses. Also move the (incomplete) implementation of the generic endpoint to the corresponding package and create a stub for the libpod handler, which will be implemented once there's an agreement on the proposed API. # Pull Add the v2 swagger documentation for the libpod/images/pull endpoint. Similar to the load endpoint, we return an array since more than one image can be pulled when the `all-tags` parameter is set. Signed-off-by: Valentin Rothberg <rothberg@redhat.com>
147 lines
2.8 KiB
Go
147 lines
2.8 KiB
Go
package handlers
|
|
|
|
import (
|
|
"github.com/containers/libpod/cmd/podman/shared"
|
|
"github.com/containers/libpod/libpod"
|
|
"github.com/containers/libpod/libpod/image"
|
|
"github.com/containers/libpod/pkg/inspect"
|
|
"github.com/docker/docker/api/types"
|
|
)
|
|
|
|
// History response
|
|
// swagger:response DocsHistory
|
|
type swagHistory struct {
|
|
// in:body
|
|
Body struct {
|
|
HistoryResponse
|
|
}
|
|
}
|
|
|
|
// Inspect response
|
|
// swagger:response DocsImageInspect
|
|
type swagImageInspect struct {
|
|
// in:body
|
|
Body struct {
|
|
ImageInspect
|
|
}
|
|
}
|
|
|
|
// Load response
|
|
// swagger:response DocsLibpodImagesLoadResponse
|
|
type swagLibpodImagesLoadResponse struct {
|
|
// in:body
|
|
Body []LibpodImagesLoadReport
|
|
}
|
|
|
|
// Import response
|
|
// swagger:response DocsLibpodImagesImportResponse
|
|
type swagLibpodImagesImportResponse struct {
|
|
// in:body
|
|
Body LibpodImagesImportReport
|
|
}
|
|
|
|
// Pull response
|
|
// swagger:response DocsLibpodImagesPullResponse
|
|
type swagLibpodImagesPullResponse struct {
|
|
// in:body
|
|
Body LibpodImagesPullReport
|
|
}
|
|
|
|
// Delete response
|
|
// swagger:response DocsImageDeleteResponse
|
|
type swagImageDeleteResponse struct {
|
|
// in:body
|
|
Body []image.ImageDeleteResponse
|
|
}
|
|
|
|
// Search results
|
|
// swagger:response DocsSearchResponse
|
|
type swagSearchResponse struct {
|
|
// in:body
|
|
Body struct {
|
|
image.SearchResult
|
|
}
|
|
}
|
|
|
|
// Inspect image
|
|
// swagger:response DocsLibpodInspectImageResponse
|
|
type swagLibpodInspectImageResponse struct {
|
|
// in:body
|
|
Body struct {
|
|
inspect.ImageData
|
|
}
|
|
}
|
|
|
|
// Prune containers
|
|
// swagger:response DocsContainerPruneReport
|
|
type swagContainerPruneReport struct {
|
|
// in: body
|
|
Body []ContainersPruneReport
|
|
}
|
|
|
|
// Prune containers
|
|
// swagger:response DocsLibpodPruneResponse
|
|
type swagLibpodContainerPruneReport struct {
|
|
// in: body
|
|
Body []LibpodContainersPruneReport
|
|
}
|
|
|
|
// Inspect container
|
|
// swagger:response DocsContainerInspectResponse
|
|
type swagContainerInspectResponse struct {
|
|
// in:body
|
|
Body struct {
|
|
types.ContainerJSON
|
|
}
|
|
}
|
|
|
|
// List processes in container
|
|
// swagger:response DockerTopResponse
|
|
type swagDockerTopResponse struct {
|
|
// in:body
|
|
Body struct {
|
|
ContainerTopOKBody
|
|
}
|
|
}
|
|
|
|
// List containers
|
|
// swagger:response LibpodListContainersResponse
|
|
type swagLibpodListContainersResponse struct {
|
|
// in:body
|
|
Body []shared.PsContainerOutput
|
|
}
|
|
|
|
// Inspect container
|
|
// swagger:response LibpodInspectContainerResponse
|
|
type swagLibpodInspectContainerResponse struct {
|
|
// in:body
|
|
Body struct {
|
|
libpod.InspectContainerData
|
|
}
|
|
}
|
|
|
|
// List pods
|
|
// swagger:response ListPodsResponse
|
|
type swagListPodsResponse struct {
|
|
// in:body
|
|
Body []libpod.PodInspect
|
|
}
|
|
|
|
// Inspect pod
|
|
// swagger:response InspectPodResponse
|
|
type swagInspectPodResponse struct {
|
|
// in:body
|
|
Body struct {
|
|
libpod.PodInspect
|
|
}
|
|
}
|
|
|
|
// Inspect volume
|
|
// swagger:response InspectVolumeResponse
|
|
type swagInspectVolumeResponse struct {
|
|
// in:body
|
|
Body struct {
|
|
libpod.InspectVolumeData
|
|
}
|
|
}
|