mirror of
https://github.com/containers/podman.git
synced 2025-07-02 00:30:00 +08:00
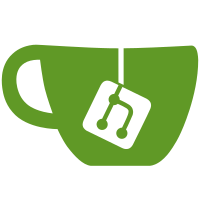
We need to vendor in the latest containerd/cgroups for a fix related to slice delegation and systemd <= 239. The opencontainer/runtime-spec is brought along for the ride. Signed-off-by: baude <bbaude@redhat.com> Closes: #1414 Approved by: mheon
82 lines
1.8 KiB
Go
82 lines
1.8 KiB
Go
package libpod
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
"io/ioutil"
|
|
"os"
|
|
"path/filepath"
|
|
"runtime"
|
|
"strings"
|
|
"testing"
|
|
|
|
rspec "github.com/opencontainers/runtime-spec/specs-go"
|
|
"github.com/stretchr/testify/assert"
|
|
)
|
|
|
|
// hookPath is the path to an example hook executable.
|
|
var hookPath string
|
|
|
|
func TestPostDeleteHooks(t *testing.T) {
|
|
ctx := context.Background()
|
|
dir, err := ioutil.TempDir("", "libpod_test_")
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
defer os.RemoveAll(dir)
|
|
|
|
statePath := filepath.Join(dir, "state")
|
|
copyPath := filepath.Join(dir, "copy")
|
|
c := Container{
|
|
config: &ContainerConfig{
|
|
ID: "123abc",
|
|
Spec: &rspec.Spec{
|
|
Annotations: map[string]string{
|
|
"a": "b",
|
|
},
|
|
},
|
|
StaticDir: dir, // not the bundle, but good enough for this test
|
|
},
|
|
state: &containerState{
|
|
ExtensionStageHooks: map[string][]rspec.Hook{
|
|
"poststop": {
|
|
rspec.Hook{
|
|
Path: hookPath,
|
|
Args: []string{"sh", "-c", fmt.Sprintf("cat >%s", statePath)},
|
|
},
|
|
rspec.Hook{
|
|
Path: "/does/not/exist",
|
|
},
|
|
rspec.Hook{
|
|
Path: hookPath,
|
|
Args: []string{"sh", "-c", fmt.Sprintf("cp %s %s", statePath, copyPath)},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
}
|
|
err = c.postDeleteHooks(ctx)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
stateRegexp := `{"ociVersion":"1\.0\.1-dev","id":"123abc","status":"stopped","bundle":"` + strings.TrimSuffix(os.TempDir(), "/") + `/libpod_test_[0-9]*","annotations":{"a":"b"}}`
|
|
for _, path := range []string{statePath, copyPath} {
|
|
t.Run(path, func(t *testing.T) {
|
|
content, err := ioutil.ReadFile(path)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
assert.Regexp(t, stateRegexp, string(content))
|
|
})
|
|
}
|
|
}
|
|
|
|
func init() {
|
|
if runtime.GOOS != "windows" {
|
|
hookPath = "/bin/sh"
|
|
} else {
|
|
panic("we need a reliable executable path on Windows")
|
|
}
|
|
}
|