mirror of
https://github.com/containers/podman.git
synced 2025-05-20 16:47:39 +08:00
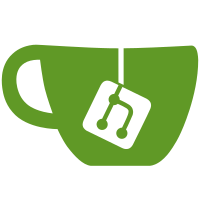
Add the ability to run the integration (ginkgo) suite using the remote client. Only the images_test.go file is run right now; all the rest are isolated with a // +build !remotelinux. As more content is developed for the remote client, we can unblock the files and just block single tests as needed. Signed-off-by: baude <bbaude@redhat.com>
75 lines
2.2 KiB
Go
75 lines
2.2 KiB
Go
// +build !remoteclient
|
|
|
|
package integration
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"time"
|
|
|
|
. "github.com/containers/libpod/test/utils"
|
|
. "github.com/onsi/ginkgo"
|
|
. "github.com/onsi/gomega"
|
|
)
|
|
|
|
var _ = Describe("Podman refresh", func() {
|
|
var (
|
|
tmpdir string
|
|
err error
|
|
podmanTest *PodmanTestIntegration
|
|
)
|
|
|
|
BeforeEach(func() {
|
|
tmpdir, err = CreateTempDirInTempDir()
|
|
if err != nil {
|
|
os.Exit(1)
|
|
}
|
|
podmanTest = PodmanTestCreate(tmpdir)
|
|
podmanTest.RestoreAllArtifacts()
|
|
})
|
|
|
|
AfterEach(func() {
|
|
podmanTest.Cleanup()
|
|
f := CurrentGinkgoTestDescription()
|
|
timedResult := fmt.Sprintf("Test: %s completed in %f seconds", f.TestText, f.Duration.Seconds())
|
|
GinkgoWriter.Write([]byte(timedResult))
|
|
})
|
|
|
|
Specify("Refresh with no containers succeeds", func() {
|
|
session := podmanTest.Podman([]string{"container", "refresh"})
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Equal(0))
|
|
})
|
|
|
|
Specify("Refresh with created container succeeds", func() {
|
|
createSession := podmanTest.Podman([]string{"create", ALPINE, "ls"})
|
|
createSession.WaitWithDefaultTimeout()
|
|
Expect(createSession.ExitCode()).To(Equal(0))
|
|
Expect(podmanTest.NumberOfContainers()).To(Equal(1))
|
|
Expect(podmanTest.NumberOfContainersRunning()).To(Equal(0))
|
|
|
|
refreshSession := podmanTest.Podman([]string{"container", "refresh"})
|
|
refreshSession.WaitWithDefaultTimeout()
|
|
Expect(refreshSession.ExitCode()).To(Equal(0))
|
|
Expect(podmanTest.NumberOfContainers()).To(Equal(1))
|
|
Expect(podmanTest.NumberOfContainersRunning()).To(Equal(0))
|
|
})
|
|
|
|
Specify("Refresh with running container restarts container", func() {
|
|
createSession := podmanTest.Podman([]string{"run", "-dt", ALPINE, "top"})
|
|
createSession.WaitWithDefaultTimeout()
|
|
Expect(createSession.ExitCode()).To(Equal(0))
|
|
Expect(podmanTest.NumberOfContainers()).To(Equal(1))
|
|
Expect(podmanTest.NumberOfContainersRunning()).To(Equal(1))
|
|
|
|
// HACK: ensure container starts before we move on
|
|
time.Sleep(1 * time.Second)
|
|
|
|
refreshSession := podmanTest.Podman([]string{"container", "refresh"})
|
|
refreshSession.WaitWithDefaultTimeout()
|
|
Expect(refreshSession.ExitCode()).To(Equal(0))
|
|
Expect(podmanTest.NumberOfContainers()).To(Equal(1))
|
|
Expect(podmanTest.NumberOfContainersRunning()).To(Equal(1))
|
|
})
|
|
})
|