mirror of
https://github.com/containers/podman.git
synced 2025-05-21 17:16:22 +08:00
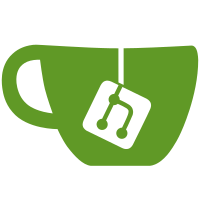
By default golang programs exit 2 on special exit signals that can be cought and produce a stack trace. However this is behavior that can be modfied via GOTRACEBACK=crash[1], in that case it does not exit(2) but rather sends itself SIGABRT to the parent sees the signal exit and out test sees that es exit code 134, 128 + 6 (SIGABRT), like most shells do. As it turns out GOTRACEBACK=crash is the default mode on all fedora and RHEL rpm builds as they patch the build with a special "rpm_crashtraceback" go build tag. While that change is old and existing for a very long time it was never caught until commit 5e240ab1f5, which switched the old ExitWithError() check that accepted anything > 0, to just accept 2. And as CI only test upstream builds that are build without rpm_crashtraceback we did not catch in CI either. Only once a user actually used distro build against the source e2e test it failed. I like to highlight that running distro builds against upstream e2e tests is not something we really support or plan to support but given this is a easy fix I decided to just fix it here as any user with GOTRACEBACK=crash set would face the same issue. While I touch this test remove the unnecessary RestoreArtifact() call which is not needed at all as we do nothing with the image and just slows the test down for now reason. [1] https://pkg.go.dev/runtime#section-sourcefiles Fixes #24213 Signed-off-by: Paul Holzinger <pholzing@redhat.com>
120 lines
3.5 KiB
Go
120 lines
3.5 KiB
Go
//go:build linux || freebsd
|
|
|
|
package integration
|
|
|
|
import (
|
|
"fmt"
|
|
"io"
|
|
"os"
|
|
"path/filepath"
|
|
"strings"
|
|
"syscall"
|
|
"time"
|
|
|
|
. "github.com/containers/podman/v5/test/utils"
|
|
. "github.com/onsi/ginkgo/v2"
|
|
. "github.com/onsi/gomega"
|
|
"golang.org/x/sys/unix"
|
|
)
|
|
|
|
const sigCatch = "trap \"echo FOO >> /h/fifo \" 8; echo READY >> /h/fifo; while :; do sleep 0.25; done"
|
|
const sigCatch2 = "trap \"echo Received\" SIGFPE; while :; do sleep 0.25; done"
|
|
|
|
var _ = Describe("Podman run with --sig-proxy", func() {
|
|
|
|
Specify("signals are forwarded to container using sig-proxy", func() {
|
|
if podmanTest.Host.Arch == "ppc64le" {
|
|
Skip("Doesn't work on ppc64le")
|
|
}
|
|
signal := syscall.SIGFPE
|
|
// Set up a socket for communication
|
|
udsDir := filepath.Join(tempdir, "socket")
|
|
err := os.Mkdir(udsDir, 0700)
|
|
Expect(err).ToNot(HaveOccurred())
|
|
udsPath := filepath.Join(udsDir, "fifo")
|
|
err = syscall.Mkfifo(udsPath, 0600)
|
|
Expect(err).ToNot(HaveOccurred())
|
|
if isRootless() {
|
|
err = podmanTest.RestoreArtifact(fedoraMinimal)
|
|
Expect(err).ToNot(HaveOccurred())
|
|
}
|
|
_, pid := podmanTest.PodmanPID([]string{"run", "-v", fmt.Sprintf("%s:/h:Z", udsDir), fedoraMinimal, "bash", "-c", sigCatch})
|
|
|
|
uds, _ := os.OpenFile(udsPath, os.O_RDONLY|syscall.O_NONBLOCK, 0600)
|
|
defer uds.Close()
|
|
|
|
// Wait for the script in the container to alert us that it is READY
|
|
counter := 0
|
|
for {
|
|
buf := make([]byte, 1024)
|
|
n, err := uds.Read(buf)
|
|
if err != nil && err != io.EOF {
|
|
GinkgoWriter.Println(err)
|
|
return
|
|
}
|
|
data := string(buf[0:n])
|
|
if strings.Contains(data, "READY") {
|
|
break
|
|
}
|
|
time.Sleep(1 * time.Second)
|
|
if counter == 15 {
|
|
Fail("Timed out waiting for READY from container")
|
|
}
|
|
counter++
|
|
}
|
|
// Ok, container is up and running now and so is the script
|
|
|
|
if err := unix.Kill(pid, signal); err != nil {
|
|
Fail(fmt.Sprintf("error killing podman process %d: %v", pid, err))
|
|
}
|
|
|
|
// The sending of the signal above will send FOO to the socket; here we
|
|
// listen to the socket for that.
|
|
counter = 0
|
|
for {
|
|
buf := make([]byte, 1024)
|
|
n, err := uds.Read(buf)
|
|
if err != nil {
|
|
GinkgoWriter.Println(err)
|
|
return
|
|
}
|
|
data := string(buf[0:n])
|
|
if strings.Contains(data, "FOO") {
|
|
break
|
|
}
|
|
time.Sleep(1 * time.Second)
|
|
if counter == 15 {
|
|
Fail("timed out waiting for FOO from container")
|
|
}
|
|
counter++
|
|
}
|
|
})
|
|
|
|
Specify("signals are not forwarded to container with sig-proxy false", func() {
|
|
signal := syscall.SIGFPE
|
|
session, pid := podmanTest.PodmanPID([]string{"run", "--name", "test2", "--sig-proxy=false", fedoraMinimal, "bash", "-c", sigCatch2})
|
|
|
|
Expect(WaitForContainer(podmanTest)).To(BeTrue(), "WaitForContainer()")
|
|
|
|
// Kill with given signal
|
|
// Should be no output, SIGPOLL is usually ignored
|
|
if err := unix.Kill(pid, signal); err != nil {
|
|
Fail(fmt.Sprintf("error killing podman process %d: %v", pid, err))
|
|
}
|
|
|
|
// Kill with -9 to guarantee the container dies
|
|
killSession := podmanTest.Podman([]string{"kill", "-s", "9", "test2"})
|
|
killSession.WaitWithDefaultTimeout()
|
|
Expect(killSession).Should(ExitCleanly())
|
|
|
|
session.WaitWithDefaultTimeout()
|
|
// Exit code is normally 2, however with GOTRACEBACK=crash (default in
|
|
// Fedora/RHEL rpm builds) it will be 134 thus allow both.
|
|
// https://github.com/containers/podman/issues/24213
|
|
errorMsg := "SIGFPE: floating-point exception"
|
|
Expect(session).To(Or(ExitWithError(2, errorMsg), ExitWithError(134, errorMsg)))
|
|
Expect(session.OutputToString()).To(Not(ContainSubstring("Received")))
|
|
})
|
|
|
|
})
|