mirror of
https://github.com/containers/podman.git
synced 2025-07-01 08:07:03 +08:00
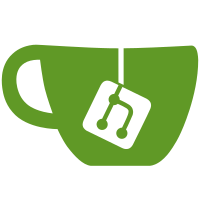
We also considered ordering with sort.Strings, but Matthew rejected that because it uses a byte-by-byte UTF-8 comparison [1] which would fail many language-specific conventions [2]. There's some more discussion of the localeToLanguage mapping in [3]. Currently language.Parse does not handle either 'C' or 'POSIX', returning: und, language: tag is not well-formed for both. [1]: https://github.com/projectatomic/libpod/pull/686#issuecomment-387914358 [2]: https://en.wikipedia.org/wiki/Alphabetical_order#Language-specific_conventions [3]: https://github.com/golang/go/issues/25340 Signed-off-by: W. Trevor King <wking@tremily.us> Closes: #686 Approved by: mheon
142 lines
2.8 KiB
Go
142 lines
2.8 KiB
Go
package hooks
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
"io/ioutil"
|
|
"os"
|
|
"path/filepath"
|
|
"testing"
|
|
"time"
|
|
|
|
rspec "github.com/opencontainers/runtime-spec/specs-go"
|
|
"github.com/stretchr/testify/assert"
|
|
"golang.org/x/text/language"
|
|
)
|
|
|
|
func TestMonitorGood(t *testing.T) {
|
|
ctx, cancel := context.WithCancel(context.Background())
|
|
dir, err := ioutil.TempDir("", "hooks-test-")
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
defer os.RemoveAll(dir)
|
|
|
|
lang, err := language.Parse("und-u-va-posix")
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
manager, err := New(ctx, []string{dir}, lang)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
sync := make(chan error, 2)
|
|
go manager.Monitor(ctx, sync)
|
|
err = <-sync
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
jsonPath := filepath.Join(dir, "a.json")
|
|
|
|
t.Run("good-addition", func(t *testing.T) {
|
|
err = ioutil.WriteFile(jsonPath, []byte(fmt.Sprintf("{\"version\": \"1.0.0\", \"hook\": {\"path\": \"%s\"}, \"when\": {\"always\": true}, \"stages\": [\"prestart\", \"poststart\", \"poststop\"]}", path)), 0644)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
time.Sleep(100 * time.Millisecond) // wait for monitor to notice
|
|
|
|
config := &rspec.Spec{}
|
|
err = manager.Hooks(config, map[string]string{}, false)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
assert.Equal(t, &rspec.Hooks{
|
|
Prestart: []rspec.Hook{
|
|
{
|
|
Path: path,
|
|
},
|
|
},
|
|
Poststart: []rspec.Hook{
|
|
{
|
|
Path: path,
|
|
},
|
|
},
|
|
Poststop: []rspec.Hook{
|
|
{
|
|
Path: path,
|
|
},
|
|
},
|
|
}, config.Hooks)
|
|
})
|
|
|
|
t.Run("good-removal", func(t *testing.T) {
|
|
err = os.Remove(jsonPath)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
time.Sleep(100 * time.Millisecond) // wait for monitor to notice
|
|
|
|
config := &rspec.Spec{}
|
|
expected := config.Hooks
|
|
err = manager.Hooks(config, map[string]string{}, false)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
assert.Equal(t, expected, config.Hooks)
|
|
})
|
|
|
|
t.Run("bad-addition", func(t *testing.T) {
|
|
err = ioutil.WriteFile(jsonPath, []byte("{\"version\": \"-1\"]}"), 0644)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
time.Sleep(100 * time.Millisecond) // wait for monitor to notice
|
|
|
|
config := &rspec.Spec{}
|
|
expected := config.Hooks
|
|
err = manager.Hooks(config, map[string]string{}, false)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
assert.Equal(t, expected, config.Hooks)
|
|
|
|
err = os.Remove(jsonPath)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
})
|
|
|
|
cancel()
|
|
err = <-sync
|
|
assert.Equal(t, context.Canceled, err)
|
|
}
|
|
|
|
func TestMonitorBadWatcher(t *testing.T) {
|
|
ctx := context.Background()
|
|
|
|
lang, err := language.Parse("und-u-va-posix")
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
manager, err := New(ctx, []string{}, lang)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
manager.directories = []string{"/does/not/exist"}
|
|
|
|
sync := make(chan error, 2)
|
|
go manager.Monitor(ctx, sync)
|
|
err = <-sync
|
|
if !os.IsNotExist(err) {
|
|
t.Fatal("opaque wrapping for not-exist errors")
|
|
}
|
|
}
|