mirror of
https://github.com/containers/podman.git
synced 2025-07-04 01:48:28 +08:00
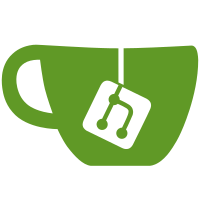
With the advent of Podman 2.0.0 we crossed the magical barrier of go modules. While we were able to continue importing all packages inside of the project, the project could not be vendored anymore from the outside. Move the go module to new major version and change all imports to `github.com/containers/libpod/v2`. The renaming of the imports was done via `gomove` [1]. [1] https://github.com/KSubedi/gomove Signed-off-by: Valentin Rothberg <rothberg@redhat.com>
88 lines
2.3 KiB
Go
88 lines
2.3 KiB
Go
package libpod
|
|
|
|
import (
|
|
"fmt"
|
|
"path/filepath"
|
|
"time"
|
|
|
|
"github.com/containers/common/pkg/config"
|
|
"github.com/containers/libpod/v2/libpod/define"
|
|
"github.com/containers/storage/pkg/stringid"
|
|
"github.com/pkg/errors"
|
|
"github.com/sirupsen/logrus"
|
|
)
|
|
|
|
// Creates a new, empty pod
|
|
func newPod(runtime *Runtime) *Pod {
|
|
pod := new(Pod)
|
|
pod.config = new(PodConfig)
|
|
pod.config.ID = stringid.GenerateNonCryptoID()
|
|
pod.config.Labels = make(map[string]string)
|
|
pod.config.CreatedTime = time.Now()
|
|
pod.config.InfraContainer = new(InfraContainerConfig)
|
|
pod.state = new(podState)
|
|
pod.runtime = runtime
|
|
|
|
return pod
|
|
}
|
|
|
|
// Update pod state from database
|
|
func (p *Pod) updatePod() error {
|
|
if err := p.runtime.state.UpdatePod(p); err != nil {
|
|
return err
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// Save pod state to database
|
|
func (p *Pod) save() error {
|
|
if err := p.runtime.state.SavePod(p); err != nil {
|
|
return errors.Wrapf(err, "error saving pod %s state", p.ID())
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// Refresh a pod's state after restart
|
|
// This cannot lock any other pod, but may lock individual containers, as those
|
|
// will have refreshed by the time pod refresh runs.
|
|
func (p *Pod) refresh() error {
|
|
// Need to to an update from the DB to pull potentially-missing state
|
|
if err := p.runtime.state.UpdatePod(p); err != nil {
|
|
return err
|
|
}
|
|
|
|
if !p.valid {
|
|
return define.ErrPodRemoved
|
|
}
|
|
|
|
// Retrieve the pod's lock
|
|
lock, err := p.runtime.lockManager.AllocateAndRetrieveLock(p.config.LockID)
|
|
if err != nil {
|
|
return errors.Wrapf(err, "error retrieving lock %d for pod %s", p.config.LockID, p.ID())
|
|
}
|
|
p.lock = lock
|
|
|
|
// We need to recreate the pod's cgroup
|
|
if p.config.UsePodCgroup {
|
|
switch p.runtime.config.Engine.CgroupManager {
|
|
case config.SystemdCgroupsManager:
|
|
cgroupPath, err := systemdSliceFromPath(p.config.CgroupParent, fmt.Sprintf("libpod_pod_%s", p.ID()))
|
|
if err != nil {
|
|
logrus.Errorf("Error creating CGroup for pod %s: %v", p.ID(), err)
|
|
}
|
|
p.state.CgroupPath = cgroupPath
|
|
case config.CgroupfsCgroupsManager:
|
|
p.state.CgroupPath = filepath.Join(p.config.CgroupParent, p.ID())
|
|
|
|
logrus.Debugf("setting pod cgroup to %s", p.state.CgroupPath)
|
|
default:
|
|
return errors.Wrapf(define.ErrInvalidArg, "unknown cgroups manager %s specified", p.runtime.config.Engine.CgroupManager)
|
|
}
|
|
}
|
|
|
|
// Save changes
|
|
return p.save()
|
|
}
|