mirror of
https://github.com/containers/podman.git
synced 2025-07-03 01:08:02 +08:00
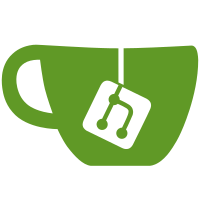
when using the bindings to *only* make a connection, the binary was rough 28MB. This PR reduces it down to 11. There is more work to do but it will come in a secondary PR. Signed-off-by: baude <bbaude@redhat.com>
45 lines
963 B
Go
45 lines
963 B
Go
package bindings
|
|
|
|
import (
|
|
"encoding/json"
|
|
"io/ioutil"
|
|
|
|
"github.com/containers/podman/v2/pkg/errorhandling"
|
|
"github.com/pkg/errors"
|
|
)
|
|
|
|
var (
|
|
ErrNotImplemented = errors.New("function not implemented")
|
|
)
|
|
|
|
func handleError(data []byte) error {
|
|
e := errorhandling.ErrorModel{}
|
|
if err := json.Unmarshal(data, &e); err != nil {
|
|
return err
|
|
}
|
|
return e
|
|
}
|
|
|
|
func (a APIResponse) Process(unmarshalInto interface{}) error {
|
|
data, err := ioutil.ReadAll(a.Response.Body)
|
|
if err != nil {
|
|
return errors.Wrap(err, "unable to process API response")
|
|
}
|
|
if a.IsSuccess() || a.IsRedirection() {
|
|
if unmarshalInto != nil {
|
|
return json.Unmarshal(data, unmarshalInto)
|
|
}
|
|
return nil
|
|
}
|
|
// TODO should we add a debug here with the response code?
|
|
return handleError(data)
|
|
}
|
|
|
|
func CheckResponseCode(inError error) (int, error) {
|
|
e, ok := inError.(errorhandling.ErrorModel)
|
|
if !ok {
|
|
return -1, errors.New("error is not type ErrorModel")
|
|
}
|
|
return e.Code(), nil
|
|
}
|