mirror of
https://github.com/containers/podman.git
synced 2025-07-06 03:34:55 +08:00
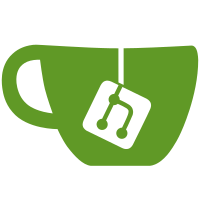
sort.Search returns the smallest index, so provide the available IDs in decreasing order. It fixes an issue when splitting the current mappings over multiple available IDs. Signed-off-by: Giuseppe Scrivano <gscrivan@redhat.com>
159 lines
2.6 KiB
Go
159 lines
2.6 KiB
Go
package rootless
|
|
|
|
import (
|
|
"reflect"
|
|
"testing"
|
|
|
|
"github.com/opencontainers/runc/libcontainer/user"
|
|
spec "github.com/opencontainers/runtime-spec/specs-go"
|
|
)
|
|
|
|
func TestMaybeSplitMappings(t *testing.T) {
|
|
mappings := []spec.LinuxIDMapping{
|
|
{
|
|
ContainerID: 0,
|
|
HostID: 0,
|
|
Size: 2,
|
|
},
|
|
}
|
|
desiredMappings := []spec.LinuxIDMapping{
|
|
{
|
|
ContainerID: 0,
|
|
HostID: 0,
|
|
Size: 1,
|
|
},
|
|
{
|
|
ContainerID: 1,
|
|
HostID: 1,
|
|
Size: 1,
|
|
},
|
|
}
|
|
availableMappings := []user.IDMap{
|
|
{
|
|
ID: 1,
|
|
ParentID: 1000000,
|
|
Count: 65536,
|
|
},
|
|
{
|
|
ID: 0,
|
|
ParentID: 1000,
|
|
Count: 1,
|
|
},
|
|
}
|
|
newMappings := MaybeSplitMappings(mappings, availableMappings)
|
|
if !reflect.DeepEqual(newMappings, desiredMappings) {
|
|
t.Fatal("wrong mappings generated")
|
|
}
|
|
|
|
mappings = []spec.LinuxIDMapping{
|
|
{
|
|
ContainerID: 0,
|
|
HostID: 0,
|
|
Size: 2,
|
|
},
|
|
}
|
|
desiredMappings = []spec.LinuxIDMapping{
|
|
{
|
|
ContainerID: 0,
|
|
HostID: 0,
|
|
Size: 2,
|
|
},
|
|
}
|
|
availableMappings = []user.IDMap{
|
|
{
|
|
ID: 0,
|
|
ParentID: 1000000,
|
|
Count: 65536,
|
|
},
|
|
}
|
|
newMappings = MaybeSplitMappings(mappings, availableMappings)
|
|
|
|
if !reflect.DeepEqual(newMappings, desiredMappings) {
|
|
t.Fatal("wrong mappings generated")
|
|
}
|
|
|
|
mappings = []spec.LinuxIDMapping{
|
|
{
|
|
ContainerID: 0,
|
|
HostID: 0,
|
|
Size: 1,
|
|
},
|
|
}
|
|
desiredMappings = []spec.LinuxIDMapping{
|
|
{
|
|
ContainerID: 0,
|
|
HostID: 0,
|
|
Size: 1,
|
|
},
|
|
}
|
|
availableMappings = []user.IDMap{
|
|
{
|
|
ID: 10000,
|
|
ParentID: 10000,
|
|
Count: 65536,
|
|
},
|
|
}
|
|
|
|
newMappings = MaybeSplitMappings(mappings, availableMappings)
|
|
if !reflect.DeepEqual(newMappings, desiredMappings) {
|
|
t.Fatal("wrong mappings generated")
|
|
}
|
|
|
|
mappings = []spec.LinuxIDMapping{
|
|
{
|
|
ContainerID: 0,
|
|
HostID: 0,
|
|
Size: 4,
|
|
},
|
|
}
|
|
desiredMappings = []spec.LinuxIDMapping{
|
|
{
|
|
ContainerID: 0,
|
|
HostID: 0,
|
|
Size: 1,
|
|
},
|
|
{
|
|
ContainerID: 1,
|
|
HostID: 1,
|
|
Size: 1,
|
|
},
|
|
{
|
|
ContainerID: 2,
|
|
HostID: 2,
|
|
Size: 1,
|
|
},
|
|
{
|
|
ContainerID: 3,
|
|
HostID: 3,
|
|
Size: 1,
|
|
},
|
|
}
|
|
availableMappings = []user.IDMap{
|
|
{
|
|
ID: 0,
|
|
ParentID: 0,
|
|
Count: 1,
|
|
},
|
|
{
|
|
ID: 1,
|
|
ParentID: 1,
|
|
Count: 1,
|
|
},
|
|
{
|
|
ID: 2,
|
|
ParentID: 2,
|
|
Count: 1,
|
|
},
|
|
{
|
|
ID: 3,
|
|
ParentID: 3,
|
|
Count: 1,
|
|
},
|
|
}
|
|
|
|
newMappings = MaybeSplitMappings(mappings, availableMappings)
|
|
if !reflect.DeepEqual(newMappings, desiredMappings) {
|
|
t.Fatal("wrong mappings generated")
|
|
}
|
|
}
|