mirror of
https://github.com/containers/podman.git
synced 2025-05-17 23:26:08 +08:00
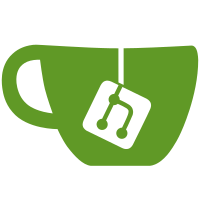
The --env is used to add new environment variable to container or override the existing one. The --unsetenv is used to remove the environment variable. It is done by sharing "env" and "unsetenv" flags between both "update" and "create" commands and later handling these flags in the "update" command handler. The list of environment variables to add/remove is stored in newly added variables in the ContainerUpdateOptions. The Container.Update API call is refactored to take the ContainerUpdateOptions as an input to limit the number of its arguments. The Env and UnsetEnv lists are later handled using the envLib package and the Container is updated. The remote API is also extended to handle Env and EnvUnset. Fixes: #24875 Signed-off-by: Jan Kaluza <jkaluza@redhat.com>
49 lines
1.4 KiB
Go
49 lines
1.4 KiB
Go
package containers
|
|
|
|
import (
|
|
"context"
|
|
"net/http"
|
|
"net/url"
|
|
"strconv"
|
|
"strings"
|
|
|
|
"github.com/containers/podman/v5/pkg/api/handlers"
|
|
"github.com/containers/podman/v5/pkg/bindings"
|
|
"github.com/containers/podman/v5/pkg/domain/entities/types"
|
|
jsoniter "github.com/json-iterator/go"
|
|
)
|
|
|
|
func Update(ctx context.Context, options *types.ContainerUpdateOptions) (string, error) {
|
|
conn, err := bindings.GetClient(ctx)
|
|
if err != nil {
|
|
return "", err
|
|
}
|
|
|
|
params := url.Values{}
|
|
if options.RestartPolicy != nil {
|
|
params.Set("restartPolicy", *options.RestartPolicy)
|
|
if options.RestartRetries != nil {
|
|
params.Set("restartRetries", strconv.Itoa(int(*options.RestartRetries)))
|
|
}
|
|
}
|
|
updateEntities := &handlers.UpdateEntities{
|
|
LinuxResources: *options.Resources,
|
|
UpdateHealthCheckConfig: *options.ChangedHealthCheckConfiguration,
|
|
UpdateContainerDevicesLimits: *options.DevicesLimits,
|
|
Env: options.Env,
|
|
UnsetEnv: options.UnsetEnv,
|
|
}
|
|
requestData, err := jsoniter.MarshalToString(updateEntities)
|
|
if err != nil {
|
|
return "", err
|
|
}
|
|
stringReader := strings.NewReader(requestData)
|
|
response, err := conn.DoRequest(ctx, stringReader, http.MethodPost, "/containers/%s/update", params, nil, options.NameOrID)
|
|
if err != nil {
|
|
return "", err
|
|
}
|
|
defer response.Body.Close()
|
|
|
|
return options.NameOrID, response.Process(nil)
|
|
}
|