mirror of
https://github.com/containers/podman.git
synced 2025-07-07 04:14:48 +08:00
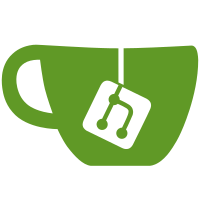
* Remove duplicate or unused types and constants * Move all documetation-only models and responses into swagger package * Remove all unecessary names, go-swagger will determine names from struct declarations * Use Libpod suffix to differentiate between compat and libpod models and responses. Taken from swagger:operation declarations. * Models and responses that start with lowercase are for swagger use only while uppercase are used "as is" in the code and swagger comments * Used gofumpt on new code ```release-note ``` Signed-off-by: Jhon Honce <jhonce@redhat.com>
117 lines
2.0 KiB
Go
117 lines
2.0 KiB
Go
//nolint:deadcode,unused // these types are used to wire generated swagger to API code
|
|
package swagger
|
|
|
|
import (
|
|
"github.com/containers/podman/v4/pkg/errorhandling"
|
|
)
|
|
|
|
// Error model embedded in swagger:response to aid in documentation generation
|
|
|
|
// No such image
|
|
// swagger:response
|
|
type imageNotFound struct {
|
|
// in:body
|
|
Body errorhandling.ErrorModel
|
|
}
|
|
|
|
// No such container
|
|
// swagger:response
|
|
type containerNotFound struct {
|
|
// in:body
|
|
Body errorhandling.ErrorModel
|
|
}
|
|
|
|
// No such network
|
|
// swagger:response
|
|
type networkNotFound struct {
|
|
// in:body
|
|
Body errorhandling.ErrorModel
|
|
}
|
|
|
|
// No such exec instance
|
|
// swagger:response
|
|
type execSessionNotFound struct {
|
|
// in:body
|
|
Body errorhandling.ErrorModel
|
|
}
|
|
|
|
// No such volume
|
|
// swagger:response
|
|
type volumeNotFound struct {
|
|
// in:body
|
|
Body errorhandling.ErrorModel
|
|
}
|
|
|
|
// No such pod
|
|
// swagger:response
|
|
type podNotFound struct {
|
|
// in:body
|
|
Body errorhandling.ErrorModel
|
|
}
|
|
|
|
// No such manifest
|
|
// swagger:response
|
|
type manifestNotFound struct {
|
|
// in:body
|
|
Body errorhandling.ErrorModel
|
|
}
|
|
|
|
// Internal server error
|
|
// swagger:response
|
|
type internalError struct {
|
|
// in:body
|
|
Body errorhandling.ErrorModel
|
|
}
|
|
|
|
// Conflict error in operation
|
|
// swagger:response
|
|
type conflictError struct {
|
|
// in:body
|
|
Body errorhandling.ErrorModel
|
|
}
|
|
|
|
// Bad parameter in request
|
|
// swagger:response
|
|
type badParamError struct {
|
|
// in:body
|
|
Body errorhandling.ErrorModel
|
|
}
|
|
|
|
// Container already started
|
|
// swagger:response
|
|
type containerAlreadyStartedError struct {
|
|
// in:body
|
|
Body errorhandling.ErrorModel
|
|
}
|
|
|
|
// Container already stopped
|
|
// swagger:response
|
|
type containerAlreadyStoppedError struct {
|
|
// in:body
|
|
Body errorhandling.ErrorModel
|
|
}
|
|
|
|
// Pod already started
|
|
// swagger:response
|
|
type podAlreadyStartedError struct {
|
|
// in:body
|
|
Body errorhandling.ErrorModel
|
|
}
|
|
|
|
// Pod already stopped
|
|
// swagger:response
|
|
type podAlreadyStoppedError struct {
|
|
// in:body
|
|
Body errorhandling.ErrorModel
|
|
}
|
|
|
|
// Success
|
|
// swagger:response
|
|
type ok struct {
|
|
// in:body
|
|
Body struct {
|
|
// example: OK
|
|
ok string
|
|
}
|
|
}
|