mirror of
https://github.com/containers/podman.git
synced 2025-05-20 16:47:39 +08:00
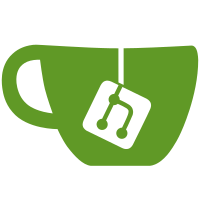
Some packages used by the remote client imported the libpod package. This is not wanted because it adds unnecessary bloat to the client and also causes problems with platform specific code(linux only), see #9710. The solution is to move the used functions/variables into extra packages which do not import libpod. This change shrinks the remote client size more than 6MB compared to the current master. [NO TESTS NEEDED] I have no idea how to test this properly but with #9710 the cross compile should fail. Signed-off-by: Paul Holzinger <paul.holzinger@web.de>
104 lines
3.1 KiB
Go
104 lines
3.1 KiB
Go
package generate
|
|
|
|
import (
|
|
"strconv"
|
|
"strings"
|
|
|
|
"github.com/containers/podman/v3/pkg/systemd/define"
|
|
"github.com/pkg/errors"
|
|
)
|
|
|
|
// minTimeoutStopSec is the minimal stop timeout for generated systemd units.
|
|
// Once exceeded, processes of the services are killed and the cgroup(s) are
|
|
// cleaned up.
|
|
const minTimeoutStopSec = 60
|
|
|
|
// validateRestartPolicy checks that the user-provided policy is valid.
|
|
func validateRestartPolicy(restart string) error {
|
|
for _, i := range define.RestartPolicies {
|
|
if i == restart {
|
|
return nil
|
|
}
|
|
}
|
|
return errors.Errorf("%s is not a valid restart policy", restart)
|
|
}
|
|
|
|
const headerTemplate = `# {{{{.ServiceName}}}}.service
|
|
{{{{- if (eq .GenerateNoHeader false) }}}}
|
|
# autogenerated by Podman {{{{.PodmanVersion}}}}
|
|
{{{{- if .TimeStamp}}}}
|
|
# {{{{.TimeStamp}}}}
|
|
{{{{- end}}}}
|
|
{{{{- end}}}}
|
|
|
|
[Unit]
|
|
Description=Podman {{{{.ServiceName}}}}.service
|
|
Documentation=man:podman-generate-systemd(1)
|
|
Wants=network.target
|
|
After=network-online.target
|
|
`
|
|
|
|
// filterPodFlags removes --pod and --pod-id-file from the specified command.
|
|
func filterPodFlags(command []string) []string {
|
|
processed := []string{}
|
|
for i := 0; i < len(command); i++ {
|
|
s := command[i]
|
|
if s == "--pod" || s == "--pod-id-file" {
|
|
i++
|
|
continue
|
|
}
|
|
if strings.HasPrefix(s, "--pod=") || strings.HasPrefix(s, "--pod-id-file=") {
|
|
continue
|
|
}
|
|
processed = append(processed, s)
|
|
}
|
|
return processed
|
|
}
|
|
|
|
// escapeSystemdArguments makes sure that all arguments with at least one whitespace
|
|
// are quoted to make sure those are interpreted as one argument instead of
|
|
// multiple ones. Also make sure to escape all characters which have a special
|
|
// meaning to systemd -> $,% and \
|
|
// see: https://www.freedesktop.org/software/systemd/man/systemd.service.html#Command%20lines
|
|
func escapeSystemdArguments(command []string) []string {
|
|
for i := range command {
|
|
command[i] = strings.ReplaceAll(command[i], "$", "$$")
|
|
command[i] = strings.ReplaceAll(command[i], "%", "%%")
|
|
if strings.ContainsAny(command[i], " \t") {
|
|
command[i] = strconv.Quote(command[i])
|
|
} else if strings.Contains(command[i], `\`) {
|
|
// strconv.Quote also escapes backslashes so
|
|
// we should replace only if strconv.Quote was not used
|
|
command[i] = strings.ReplaceAll(command[i], `\`, `\\`)
|
|
}
|
|
}
|
|
return command
|
|
}
|
|
|
|
func removeDetachArg(args []string, argCount int) []string {
|
|
// "--detach=false" could also be in the container entrypoint
|
|
// split them off so we do not remove it there
|
|
realArgs := args[len(args)-argCount:]
|
|
flagArgs := removeArg("-d=false", args[:len(args)-argCount])
|
|
flagArgs = removeArg("--detach=false", flagArgs)
|
|
return append(flagArgs, realArgs...)
|
|
}
|
|
|
|
func removeReplaceArg(args []string, argCount int) []string {
|
|
// "--replace=false" could also be in the container entrypoint
|
|
// split them off so we do not remove it there
|
|
realArgs := args[len(args)-argCount:]
|
|
flagArgs := removeArg("--replace=false", args[:len(args)-argCount])
|
|
return append(flagArgs, realArgs...)
|
|
}
|
|
|
|
func removeArg(arg string, args []string) []string {
|
|
newArgs := []string{}
|
|
for _, a := range args {
|
|
if a != arg {
|
|
newArgs = append(newArgs, a)
|
|
}
|
|
}
|
|
return newArgs
|
|
}
|