mirror of
https://github.com/containers/podman.git
synced 2025-05-28 21:46:51 +08:00
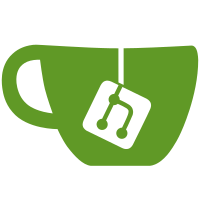
reworking binding and endpoint to actually work. added documentation in swagger for and various return code possibilities. add a good start on tests though we need some other container functions not yet implemented for that. Signed-off-by: Brent Baude <bbaude@redhat.com>
44 lines
1.0 KiB
Go
44 lines
1.0 KiB
Go
package libpod
|
|
|
|
import (
|
|
"net/http"
|
|
|
|
"github.com/containers/libpod/libpod"
|
|
"github.com/containers/libpod/pkg/api/handlers/utils"
|
|
)
|
|
|
|
func RunHealthCheck(w http.ResponseWriter, r *http.Request) {
|
|
runtime := r.Context().Value("runtime").(*libpod.Runtime)
|
|
name := utils.GetName(r)
|
|
status, err := runtime.HealthCheck(name)
|
|
if err != nil {
|
|
if status == libpod.HealthCheckContainerNotFound {
|
|
utils.ContainerNotFound(w, name, err)
|
|
return
|
|
}
|
|
if status == libpod.HealthCheckNotDefined {
|
|
utils.Error(w, "no healthcheck defined", http.StatusConflict, err)
|
|
return
|
|
}
|
|
if status == libpod.HealthCheckContainerStopped {
|
|
utils.Error(w, "container not running", http.StatusConflict, err)
|
|
return
|
|
}
|
|
utils.InternalServerError(w, err)
|
|
return
|
|
}
|
|
ctr, err := runtime.LookupContainer(name)
|
|
if err != nil {
|
|
utils.InternalServerError(w, err)
|
|
return
|
|
}
|
|
|
|
hcLog, err := ctr.GetHealthCheckLog()
|
|
if err != nil {
|
|
utils.InternalServerError(w, err)
|
|
return
|
|
}
|
|
|
|
utils.WriteResponse(w, http.StatusOK, hcLog)
|
|
}
|