mirror of
https://github.com/containers/podman.git
synced 2025-05-22 09:36:57 +08:00
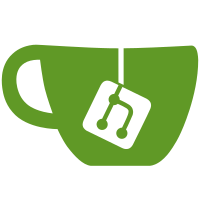
Add binding for networks and begin documentation for binding methods for godoc. Also, add major functions to their own subpackages so reduce the amount of of method confusion. So instead of: bindings.ListImages(), we now do a [bindings].images.List(). Also, the connection is passed to each binding method via a context to allow for future growth. Lastly, add first set of tests. There are a couple of things to work out for rootless tests yet. Signed-off-by: Brent Baude <bbaude@redhat.com>
103 lines
2.9 KiB
Go
103 lines
2.9 KiB
Go
package utils
|
|
|
|
import (
|
|
"fmt"
|
|
"net/http"
|
|
|
|
"github.com/containers/libpod/libpod/define"
|
|
"github.com/pkg/errors"
|
|
log "github.com/sirupsen/logrus"
|
|
)
|
|
|
|
var (
|
|
ErrLinkNotSupport = errors.New("Link is not supported")
|
|
)
|
|
|
|
// Error formats an API response to an error
|
|
//
|
|
// apiMessage and code must match the container API, and are sent to client
|
|
// err is logged on the system running the podman service
|
|
func Error(w http.ResponseWriter, apiMessage string, code int, err error) {
|
|
// Log detailed message of what happened to machine running podman service
|
|
log.Infof("Request Failed(%s): %s", http.StatusText(code), err.Error())
|
|
em := ErrorModel{
|
|
Because: (errors.Cause(err)).Error(),
|
|
Message: err.Error(),
|
|
ResponseCode: code,
|
|
}
|
|
WriteJSON(w, code, em)
|
|
}
|
|
|
|
func VolumeNotFound(w http.ResponseWriter, name string, err error) {
|
|
if errors.Cause(err) != define.ErrNoSuchVolume {
|
|
InternalServerError(w, err)
|
|
}
|
|
msg := fmt.Sprintf("No such volume: %s", name)
|
|
Error(w, msg, http.StatusNotFound, err)
|
|
}
|
|
func ContainerNotFound(w http.ResponseWriter, name string, err error) {
|
|
if errors.Cause(err) != define.ErrNoSuchCtr {
|
|
InternalServerError(w, err)
|
|
}
|
|
msg := fmt.Sprintf("No such container: %s", name)
|
|
Error(w, msg, http.StatusNotFound, err)
|
|
}
|
|
|
|
func ImageNotFound(w http.ResponseWriter, name string, err error) {
|
|
if errors.Cause(err) != define.ErrNoSuchImage {
|
|
InternalServerError(w, err)
|
|
}
|
|
msg := fmt.Sprintf("No such image: %s", name)
|
|
Error(w, msg, http.StatusNotFound, err)
|
|
}
|
|
|
|
func PodNotFound(w http.ResponseWriter, name string, err error) {
|
|
if errors.Cause(err) != define.ErrNoSuchPod {
|
|
InternalServerError(w, err)
|
|
}
|
|
msg := fmt.Sprintf("No such pod: %s", name)
|
|
Error(w, msg, http.StatusNotFound, err)
|
|
}
|
|
|
|
func ContainerNotRunning(w http.ResponseWriter, containerID string, err error) {
|
|
msg := fmt.Sprintf("Container %s is not running", containerID)
|
|
Error(w, msg, http.StatusConflict, err)
|
|
}
|
|
|
|
func InternalServerError(w http.ResponseWriter, err error) {
|
|
Error(w, http.StatusText(http.StatusInternalServerError), http.StatusInternalServerError, err)
|
|
}
|
|
|
|
func BadRequest(w http.ResponseWriter, key string, value string, err error) {
|
|
e := errors.Wrapf(err, "Failed to parse query parameter '%s': %q", key, value)
|
|
Error(w, http.StatusText(http.StatusBadRequest), http.StatusBadRequest, e)
|
|
}
|
|
|
|
type ErrorModel struct {
|
|
// API root cause formatted for automated parsing
|
|
// example: API root cause
|
|
Because string `json:"cause"`
|
|
// human error message, formatted for a human to read
|
|
// example: human error message
|
|
Message string `json:"message"`
|
|
// http response code
|
|
ResponseCode int `json:"response"`
|
|
}
|
|
|
|
func (e ErrorModel) Error() string {
|
|
return e.Message
|
|
}
|
|
|
|
func (e ErrorModel) Cause() error {
|
|
return errors.New(e.Because)
|
|
}
|
|
|
|
func (e ErrorModel) Code() int {
|
|
return e.ResponseCode
|
|
}
|
|
|
|
// UnsupportedParameter logs a given param by its string name as not supported.
|
|
func UnSupportedParameter(param string) {
|
|
log.Infof("API parameter %q: not supported", param)
|
|
}
|