mirror of
https://github.com/containers/podman.git
synced 2025-05-22 17:46:52 +08:00
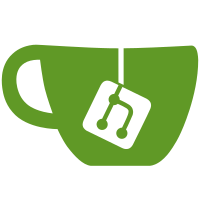
Do not require 0755 permissons for the ~/.config directory but require at least 0700 which should be sufficient. The current implementation internally creates this directory with 0755 if it does not exist, but if the directory already exists with different perissions the current code returns an empty string. Signed-off-by: Christian Felder <c.felder@fz-juelich.de>
109 lines
3.0 KiB
Go
109 lines
3.0 KiB
Go
// +build linux darwin
|
|
|
|
package util
|
|
|
|
// TODO once rootless function is consolidated under libpod, we
|
|
// should work to take darwin from this
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"path/filepath"
|
|
"syscall"
|
|
|
|
"github.com/containers/libpod/pkg/rootless"
|
|
"github.com/pkg/errors"
|
|
"github.com/sirupsen/logrus"
|
|
)
|
|
|
|
// GetRuntimeDir returns the runtime directory
|
|
func GetRuntimeDir() (string, error) {
|
|
var rootlessRuntimeDirError error
|
|
|
|
rootlessRuntimeDirOnce.Do(func() {
|
|
runtimeDir := os.Getenv("XDG_RUNTIME_DIR")
|
|
uid := fmt.Sprintf("%d", rootless.GetRootlessUID())
|
|
if runtimeDir == "" {
|
|
tmpDir := filepath.Join("/run", "user", uid)
|
|
if err := os.MkdirAll(tmpDir, 0700); err != nil {
|
|
logrus.Debugf("unable to make temp dir %s", tmpDir)
|
|
}
|
|
st, err := os.Stat(tmpDir)
|
|
if err == nil && int(st.Sys().(*syscall.Stat_t).Uid) == os.Geteuid() && st.Mode().Perm() == 0700 {
|
|
runtimeDir = tmpDir
|
|
}
|
|
}
|
|
if runtimeDir == "" {
|
|
tmpDir := filepath.Join(os.TempDir(), fmt.Sprintf("run-%s", uid))
|
|
if err := os.MkdirAll(tmpDir, 0700); err != nil {
|
|
logrus.Debugf("unable to make temp dir %s", tmpDir)
|
|
}
|
|
st, err := os.Stat(tmpDir)
|
|
if err == nil && int(st.Sys().(*syscall.Stat_t).Uid) == os.Geteuid() && st.Mode().Perm() == 0700 {
|
|
runtimeDir = tmpDir
|
|
}
|
|
}
|
|
if runtimeDir == "" {
|
|
home := os.Getenv("HOME")
|
|
if home == "" {
|
|
rootlessRuntimeDirError = fmt.Errorf("neither XDG_RUNTIME_DIR nor HOME was set non-empty")
|
|
return
|
|
}
|
|
resolvedHome, err := filepath.EvalSymlinks(home)
|
|
if err != nil {
|
|
rootlessRuntimeDirError = errors.Wrapf(err, "cannot resolve %s", home)
|
|
return
|
|
}
|
|
runtimeDir = filepath.Join(resolvedHome, "rundir")
|
|
}
|
|
rootlessRuntimeDir = runtimeDir
|
|
})
|
|
|
|
if rootlessRuntimeDirError != nil {
|
|
return "", rootlessRuntimeDirError
|
|
}
|
|
return rootlessRuntimeDir, nil
|
|
}
|
|
|
|
// GetRootlessConfigHomeDir returns the config home directory when running as non root
|
|
func GetRootlessConfigHomeDir() (string, error) {
|
|
var rootlessConfigHomeDirError error
|
|
|
|
rootlessConfigHomeDirOnce.Do(func() {
|
|
cfgHomeDir := os.Getenv("XDG_CONFIG_HOME")
|
|
if cfgHomeDir == "" {
|
|
home := os.Getenv("HOME")
|
|
resolvedHome, err := filepath.EvalSymlinks(home)
|
|
if err != nil {
|
|
rootlessConfigHomeDirError = errors.Wrapf(err, "cannot resolve %s", home)
|
|
return
|
|
}
|
|
tmpDir := filepath.Join(resolvedHome, ".config")
|
|
if err := os.MkdirAll(tmpDir, 0755); err != nil {
|
|
logrus.Errorf("unable to make temp dir %s", tmpDir)
|
|
}
|
|
st, err := os.Stat(tmpDir)
|
|
if err == nil && int(st.Sys().(*syscall.Stat_t).Uid) == os.Geteuid() && st.Mode().Perm() >= 0700 {
|
|
cfgHomeDir = tmpDir
|
|
}
|
|
}
|
|
rootlessConfigHomeDir = cfgHomeDir
|
|
})
|
|
|
|
if rootlessConfigHomeDirError != nil {
|
|
return "", rootlessConfigHomeDirError
|
|
}
|
|
|
|
return rootlessConfigHomeDir, nil
|
|
}
|
|
|
|
// GetRootlessPauseProcessPidPath returns the path to the file that holds the pid for
|
|
// the pause process
|
|
func GetRootlessPauseProcessPidPath() (string, error) {
|
|
runtimeDir, err := GetRuntimeDir()
|
|
if err != nil {
|
|
return "", err
|
|
}
|
|
return filepath.Join(runtimeDir, "libpod", "pause.pid"), nil
|
|
}
|