mirror of
https://github.com/containers/podman.git
synced 2025-05-20 00:27:03 +08:00
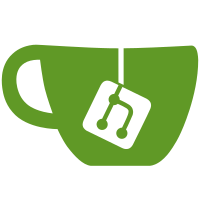
As @mheon pointed out in PR #17055[^1], isVirtualConsoleDevice() does not only matches VT device paths but also devices named like /dev/tty0abcd. This causes that non VT device paths named /dev/tty[0-9]+[A-Za-z]+ are not mounted into privileged container and systemd containers accidentally. This is an unlikely issue because the Linux kernel does not use device paths like that. To make it failproof and prevent issues in unlikely scenarios, change isVirtualConsoleDevice() to exactly match ^/dev/tty[0-9]+$ paths. Because it is not possible to match this path exactly with Glob syntax, the path is now checked with strings.TrimPrefix() and strconv.ParseUint(). ParseUint uses a bitsize of 16, this is sufficient because the max number of TTY devices is 512 in Linux 6.1.5. (Checked via 'git grep -e '#define' --and -e 'TTY_MINORS'). The commit also adds a unit-test for isVirtualConsoleDevice(). Fixes: f4c81b0aa5fd ("Only prevent VTs to be mounted inside...") [^1]: https://github.com/containers/podman/pull/17055#issuecomment-1378904068 Signed-off-by: Fabian Holler <mail@fholler.de> Signed-off-by: Daniel J Walsh <dwalsh@redhat.com>
55 lines
935 B
Go
55 lines
935 B
Go
package util
|
|
|
|
import (
|
|
"testing"
|
|
)
|
|
|
|
func TestIsVirtualConsoleDevice(t *testing.T) {
|
|
testcases := []struct {
|
|
expectedResult bool
|
|
path string
|
|
}{
|
|
{
|
|
expectedResult: true,
|
|
path: "/dev/tty10",
|
|
},
|
|
{
|
|
expectedResult: false,
|
|
path: "/dev/tty",
|
|
},
|
|
{
|
|
expectedResult: false,
|
|
path: "/dev/ttyUSB0",
|
|
},
|
|
{
|
|
expectedResult: false,
|
|
path: "/dev/tty0abcd",
|
|
},
|
|
{
|
|
expectedResult: false,
|
|
path: "1234",
|
|
},
|
|
{
|
|
expectedResult: false,
|
|
path: "abc",
|
|
},
|
|
{
|
|
expectedResult: false,
|
|
path: " ",
|
|
},
|
|
{
|
|
expectedResult: false,
|
|
path: "",
|
|
},
|
|
}
|
|
|
|
for _, tc := range testcases {
|
|
t.Run(tc.path, func(t *testing.T) {
|
|
result := isVirtualConsoleDevice(tc.path)
|
|
if result != tc.expectedResult {
|
|
t.Errorf("isVirtualConsoleDevice returned %t, expected %t", result, tc.expectedResult)
|
|
}
|
|
})
|
|
}
|
|
}
|