mirror of
https://github.com/containers/podman.git
synced 2025-05-21 09:05:56 +08:00
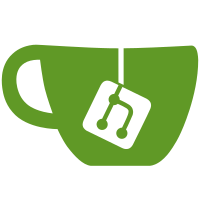
Fix handling of "bind" and "tmpfs" olumes to actually work. Allow bind, tmpfs local volumes to work in rootless mode. Also removed the string "error" from all error messages that begine with it. All Podman commands are printed with Error:, so this causes an ugly stutter. Fixes: https://github.com/containers/podman/issues/12013 Signed-off-by: Daniel J Walsh <dwalsh@redhat.com>
90 lines
2.2 KiB
Go
90 lines
2.2 KiB
Go
package libpod
|
|
|
|
import (
|
|
"os"
|
|
"path/filepath"
|
|
|
|
"github.com/containers/podman/v3/libpod/define"
|
|
"github.com/pkg/errors"
|
|
)
|
|
|
|
// Creates a new volume
|
|
func newVolume(runtime *Runtime) *Volume {
|
|
volume := new(Volume)
|
|
volume.config = new(VolumeConfig)
|
|
volume.state = new(VolumeState)
|
|
volume.runtime = runtime
|
|
volume.config.Labels = make(map[string]string)
|
|
volume.config.Options = make(map[string]string)
|
|
volume.state.NeedsCopyUp = true
|
|
volume.state.NeedsChown = true
|
|
return volume
|
|
}
|
|
|
|
// teardownStorage deletes the volume from volumePath
|
|
func (v *Volume) teardownStorage() error {
|
|
if v.UsesVolumeDriver() {
|
|
return nil
|
|
}
|
|
|
|
// TODO: Should this be converted to use v.config.MountPoint?
|
|
return os.RemoveAll(filepath.Join(v.runtime.config.Engine.VolumePath, v.Name()))
|
|
}
|
|
|
|
// Volumes with options set, or a filesystem type, or a device to mount need to
|
|
// be mounted and unmounted.
|
|
func (v *Volume) needsMount() bool {
|
|
// Non-local driver always needs mount
|
|
if v.UsesVolumeDriver() {
|
|
return true
|
|
}
|
|
|
|
// Commit 28138dafcc added the UID and GID options to this map
|
|
// However we should only mount when options other than uid and gid are set.
|
|
// see https://github.com/containers/podman/issues/10620
|
|
index := 0
|
|
if _, ok := v.config.Options["UID"]; ok {
|
|
index++
|
|
}
|
|
if _, ok := v.config.Options["GID"]; ok {
|
|
index++
|
|
}
|
|
if _, ok := v.config.Options["SIZE"]; ok {
|
|
index++
|
|
}
|
|
// when uid or gid is set there is also the "o" option
|
|
// set so we have to ignore this one as well
|
|
if index > 0 {
|
|
index++
|
|
}
|
|
// Local driver with options other than uid,gid needs mount
|
|
return len(v.config.Options) > index
|
|
}
|
|
|
|
// update() updates the volume state from the DB.
|
|
func (v *Volume) update() error {
|
|
if err := v.runtime.state.UpdateVolume(v); err != nil {
|
|
return err
|
|
}
|
|
if !v.valid {
|
|
return define.ErrVolumeRemoved
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// save() saves the volume state to the DB
|
|
func (v *Volume) save() error {
|
|
return v.runtime.state.SaveVolume(v)
|
|
}
|
|
|
|
// Refresh volume state after a restart.
|
|
func (v *Volume) refresh() error {
|
|
lock, err := v.runtime.lockManager.AllocateAndRetrieveLock(v.config.LockID)
|
|
if err != nil {
|
|
return errors.Wrapf(err, "acquiring lock %d for volume %s", v.config.LockID, v.Name())
|
|
}
|
|
v.lock = lock
|
|
|
|
return nil
|
|
}
|