mirror of
https://github.com/containers/podman.git
synced 2025-05-21 17:16:22 +08:00
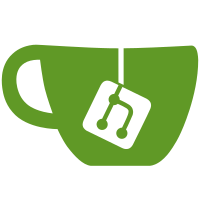
Add auto-update support to `podman kube play`. Auto-update policies can be configured for: * the entire pod via the `io.containers.autoupdate` annotation * a specific container via the `io.containers.autoupdate/$name` annotation To make use of rollbacks, the `io.containers.sdnotify` policy should be set to `container` such that the workload running _inside_ the container can send the READY message via the NOTIFY_SOCKET once ready. For further details on auto updates and rollbacks, please refer to the specific article [1]. Since auto updates and rollbacks bases on Podman's systemd integration, the k8s YAML must be executed in the `podman-kube@` systemd template. For further details on how to run k8s YAML in systemd via Podman, please refer to the specific article [2]. An examplary k8s YAML may look as follows: ```YAML apiVersion: v1 kind: Pod metadata: annotations: io.containers.autoupdate: "local" io.containers.autoupdate/b: "registry" labels: app: test name: test_pod spec: containers: - command: - top image: alpine name: a - command: - top image: alpine name: b ``` [1] https://www.redhat.com/sysadmin/podman-auto-updates-rollbacks [2] https://www.redhat.com/sysadmin/kubernetes-workloads-podman-systemd Signed-off-by: Valentin Rothberg <vrothberg@redhat.com>
59 lines
1.3 KiB
Go
59 lines
1.3 KiB
Go
package notifyproxy
|
|
|
|
import (
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/coreos/go-systemd/v22/daemon"
|
|
"github.com/stretchr/testify/require"
|
|
)
|
|
|
|
// Helper function to send the specified message over the socket of the proxy.
|
|
func sendMessage(t *testing.T, proxy *NotifyProxy, message string) {
|
|
err := SendMessage(proxy.SocketPath(), message)
|
|
require.NoError(t, err)
|
|
}
|
|
|
|
func TestNotifyProxy(t *testing.T) {
|
|
proxy, err := New("")
|
|
require.NoError(t, err)
|
|
require.FileExists(t, proxy.SocketPath())
|
|
require.NoError(t, proxy.close())
|
|
require.NoFileExists(t, proxy.SocketPath())
|
|
}
|
|
|
|
func TestWaitAndClose(t *testing.T) {
|
|
proxy, err := New("")
|
|
require.NoError(t, err)
|
|
require.FileExists(t, proxy.SocketPath())
|
|
|
|
ch := make(chan error)
|
|
|
|
go func() {
|
|
ch <- proxy.WaitAndClose()
|
|
}()
|
|
|
|
sendMessage(t, proxy, "foo\n")
|
|
time.Sleep(250 * time.Millisecond)
|
|
select {
|
|
case err := <-ch:
|
|
t.Fatalf("Should still be waiting but received %v", err)
|
|
default:
|
|
}
|
|
|
|
sendMessage(t, proxy, daemon.SdNotifyReady+"\nsomething else\n")
|
|
done := func() bool {
|
|
for i := 0; i < 10; i++ {
|
|
select {
|
|
case err := <-ch:
|
|
require.NoError(t, err, "Waiting should succeed")
|
|
return true
|
|
default:
|
|
time.Sleep(time.Duration(i*250) * time.Millisecond)
|
|
}
|
|
}
|
|
return false
|
|
}()
|
|
require.True(t, done, "READY MESSAGE SHOULD HAVE ARRIVED")
|
|
}
|