mirror of
https://github.com/containers/podman.git
synced 2025-05-21 00:56:36 +08:00
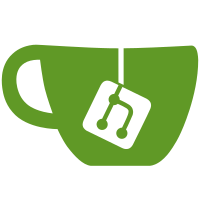
Currently --tmpdir changes the location of the pause.pid file. this causes issues because the c code in pkg/rootless does not know about that. I tried to fix this[1] by fixing the c code to not use the shortcut. While this fix worked it will result in many pause processes leaking in the integrration tests. Commit ab88632 added this behavior but following the disccusion it was never the intention that we end up having more than one pause process. The issues that was trying to fix was caused by somthing else AFAICT, the main problem seems to be that the pause.pid file parent directory may not be created when we try to create the pid file so it failed with ENOENT. This patch fixes it by creating this directory always and revert the change to no longer depend on the tmpdir value. With this commit we now always use XDG_RUNTIME_DIR/libpod/tmp/pause.pid for all podman processes. This allows the c shortcut to work reliably and should therefore improve perfomance over my other approach. A system test is added to ensure we see the right behavior and that podman system migrate actually stops the pause process. Thanks to Ed Santiago for the improved test to make it work for both `catatonit` and `podman pause`. This should fix the issues with namespace missmatches that we can see in CI as flakes. [1] https://github.com/containers/podman/pull/18057 Fixes #18057 Signed-off-by: Paul Holzinger <pholzing@redhat.com>
101 lines
2.6 KiB
Go
101 lines
2.6 KiB
Go
//go:build linux
|
|
// +build linux
|
|
|
|
package libpod
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"path/filepath"
|
|
"strconv"
|
|
"syscall"
|
|
|
|
"github.com/containers/podman/v4/libpod/define"
|
|
"github.com/containers/podman/v4/pkg/rootless"
|
|
"github.com/containers/podman/v4/pkg/util"
|
|
"github.com/sirupsen/logrus"
|
|
)
|
|
|
|
func (r *Runtime) stopPauseProcess() error {
|
|
if rootless.IsRootless() {
|
|
pausePidPath, err := util.GetRootlessPauseProcessPidPath()
|
|
if err != nil {
|
|
return fmt.Errorf("could not get pause process pid file path: %w", err)
|
|
}
|
|
data, err := os.ReadFile(pausePidPath)
|
|
if err != nil {
|
|
if os.IsNotExist(err) {
|
|
return nil
|
|
}
|
|
return fmt.Errorf("cannot read pause process pid file: %w", err)
|
|
}
|
|
pausePid, err := strconv.Atoi(string(data))
|
|
if err != nil {
|
|
return fmt.Errorf("cannot parse pause pid file %s: %w", pausePidPath, err)
|
|
}
|
|
if err := os.Remove(pausePidPath); err != nil {
|
|
return fmt.Errorf("cannot delete pause pid file %s: %w", pausePidPath, err)
|
|
}
|
|
if err := syscall.Kill(pausePid, syscall.SIGKILL); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (r *Runtime) migrate() error {
|
|
runningContainers, err := r.GetRunningContainers()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
allCtrs, err := r.state.AllContainers(false)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
logrus.Infof("Stopping all containers")
|
|
for _, ctr := range runningContainers {
|
|
fmt.Printf("stopped %s\n", ctr.ID())
|
|
if err := ctr.Stop(); err != nil {
|
|
return fmt.Errorf("cannot stop container %s: %w", ctr.ID(), err)
|
|
}
|
|
}
|
|
|
|
// Did the user request a new runtime?
|
|
runtimeChangeRequested := r.migrateRuntime != ""
|
|
requestedRuntime, runtimeExists := r.ociRuntimes[r.migrateRuntime]
|
|
if !runtimeExists && runtimeChangeRequested {
|
|
return fmt.Errorf("change to runtime %q requested but no such runtime is defined: %w", r.migrateRuntime, define.ErrInvalidArg)
|
|
}
|
|
|
|
for _, ctr := range allCtrs {
|
|
needsWrite := false
|
|
|
|
// Reset pause process location
|
|
oldLocation := filepath.Join(ctr.state.RunDir, "conmon.pid")
|
|
if ctr.config.ConmonPidFile == oldLocation {
|
|
logrus.Infof("Changing conmon PID file for %s", ctr.ID())
|
|
ctr.config.ConmonPidFile = filepath.Join(ctr.config.StaticDir, "conmon.pid")
|
|
needsWrite = true
|
|
}
|
|
|
|
// Reset runtime
|
|
if runtimeChangeRequested {
|
|
logrus.Infof("Resetting container %s runtime to runtime %s", ctr.ID(), r.migrateRuntime)
|
|
ctr.config.OCIRuntime = r.migrateRuntime
|
|
ctr.ociRuntime = requestedRuntime
|
|
|
|
needsWrite = true
|
|
}
|
|
|
|
if needsWrite {
|
|
if err := r.state.RewriteContainerConfig(ctr, ctr.config); err != nil {
|
|
return fmt.Errorf("rewriting config for container %s: %w", ctr.ID(), err)
|
|
}
|
|
}
|
|
}
|
|
|
|
return r.stopPauseProcess()
|
|
}
|