mirror of
https://github.com/containers/podman.git
synced 2025-05-20 08:36:23 +08:00
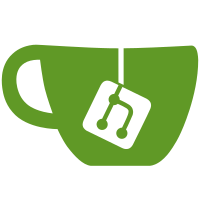
The default policy file /etc/containers/policy.json location does not work on windows and for packages that ship a default. Now we search for the policy.json in the following overwrite locations: macos and linux: - ~/.config/containers/policy.json - /etc/containers/policy.json windows: - %APPDATA%\containers\policy.json Also it offers an additional DefaultPolicyJSONPath var that should be overwritten at built time with the path of the file that is shipped by packagers. Thile file is used when none of the overwrite paths exist. [NO NEW TESTS NEEDED] Signed-off-by: Paul Holzinger <pholzing@redhat.com>
86 lines
2.3 KiB
Go
86 lines
2.3 KiB
Go
package ocipull
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
"os"
|
|
|
|
"github.com/containers/buildah/pkg/parse"
|
|
"github.com/containers/image/v5/copy"
|
|
"github.com/containers/image/v5/oci/layout"
|
|
"github.com/containers/image/v5/pkg/shortnames"
|
|
"github.com/containers/image/v5/signature"
|
|
"github.com/containers/image/v5/transports/alltransports"
|
|
"github.com/containers/image/v5/types"
|
|
"github.com/containers/podman/v5/pkg/machine/define"
|
|
)
|
|
|
|
// PullOptions includes data to alter certain knobs when pulling a source
|
|
// image.
|
|
type PullOptions struct {
|
|
// Require HTTPS and verify certificates when accessing the registry.
|
|
TLSVerify bool
|
|
// [username[:password] to use when connecting to the registry.
|
|
Credentials string
|
|
// Quiet the progress bars when pushing.
|
|
Quiet bool
|
|
}
|
|
|
|
// Pull `imageInput` from a container registry to `sourcePath`.
|
|
func Pull(ctx context.Context, imageInput types.ImageReference, localDestPath *define.VMFile, options *PullOptions) error {
|
|
destRef, err := layout.ParseReference(localDestPath.GetPath())
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
sysCtx := &types.SystemContext{
|
|
DockerInsecureSkipTLSVerify: types.NewOptionalBool(!options.TLSVerify),
|
|
}
|
|
if options.Credentials != "" {
|
|
authConf, err := parse.AuthConfig(options.Credentials)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
sysCtx.DockerAuthConfig = authConf
|
|
}
|
|
|
|
path, err := policyPath()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
policy, err := signature.NewPolicyFromFile(path)
|
|
if err != nil {
|
|
return fmt.Errorf("obtaining signature policy: %w", err)
|
|
}
|
|
policyContext, err := signature.NewPolicyContext(policy)
|
|
if err != nil {
|
|
return fmt.Errorf("creating new signature policy context: %w", err)
|
|
}
|
|
|
|
copyOpts := copy.Options{
|
|
SourceCtx: sysCtx,
|
|
}
|
|
if !options.Quiet {
|
|
copyOpts.ReportWriter = os.Stderr
|
|
}
|
|
if _, err := copy.Image(ctx, policyContext, destRef, imageInput, ©Opts); err != nil {
|
|
return fmt.Errorf("pulling source image: %w", err)
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
func stringToImageReference(imageInput string) (types.ImageReference, error) { //nolint:unused
|
|
if shortnames.IsShortName(imageInput) {
|
|
return nil, fmt.Errorf("pulling source images by short name (%q) is not supported, please use a fully-qualified name", imageInput)
|
|
}
|
|
|
|
ref, err := alltransports.ParseImageName("docker://" + imageInput)
|
|
if err != nil {
|
|
return nil, fmt.Errorf("parsing image name: %w", err)
|
|
}
|
|
|
|
return ref, nil
|
|
}
|