mirror of
https://github.com/containers/podman.git
synced 2025-05-20 08:36:23 +08:00
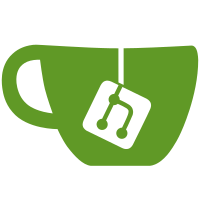
Commit 2 of 2: individual special-case handling of tests which did not pass under ExitCleanly(), one or more of: - add "-q" to commit & push commands - add ErrorToString() checks - remove unnecessary ErrorToString() checks Signed-off-by: Ed Santiago <santiago@redhat.com>
394 lines
12 KiB
Go
394 lines
12 KiB
Go
package integration
|
|
|
|
import (
|
|
"fmt"
|
|
"net/url"
|
|
"os"
|
|
"os/exec"
|
|
"os/user"
|
|
"path/filepath"
|
|
|
|
"github.com/containers/common/pkg/config"
|
|
. "github.com/containers/podman/v4/test/utils"
|
|
. "github.com/onsi/ginkgo/v2"
|
|
. "github.com/onsi/gomega"
|
|
. "github.com/onsi/gomega/gbytes"
|
|
. "github.com/onsi/gomega/gexec"
|
|
)
|
|
|
|
func setupEmptyContainersConf() {
|
|
// make sure connections are not written to real user config on host
|
|
file := filepath.Join(podmanTest.TempDir, "containersconf")
|
|
f, err := os.Create(file)
|
|
Expect(err).ToNot(HaveOccurred())
|
|
f.Close()
|
|
os.Setenv("CONTAINERS_CONF", file)
|
|
}
|
|
|
|
var _ = Describe("podman system connection", func() {
|
|
|
|
BeforeEach(setupEmptyContainersConf)
|
|
|
|
Context("without running API service", func() {
|
|
It("add ssh://", func() {
|
|
cmd := []string{"system", "connection", "add",
|
|
"--default",
|
|
"--identity", "~/.ssh/id_rsa",
|
|
"QA",
|
|
"ssh://root@podman.test:2222/run/podman/podman.sock",
|
|
}
|
|
session := podmanTest.Podman(cmd)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(ExitCleanly())
|
|
Expect(session.Out.Contents()).Should(BeEmpty())
|
|
|
|
cfg, err := config.ReadCustomConfig()
|
|
Expect(err).ShouldNot(HaveOccurred())
|
|
Expect(cfg).Should(HaveActiveService("QA"))
|
|
Expect(cfg).Should(VerifyService(
|
|
"QA",
|
|
"ssh://root@podman.test:2222/run/podman/podman.sock",
|
|
"~/.ssh/id_rsa",
|
|
))
|
|
|
|
cmd = []string{"system", "connection", "rename",
|
|
"QA",
|
|
"QE",
|
|
}
|
|
session = podmanTest.Podman(cmd)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(ExitCleanly())
|
|
|
|
Expect(config.ReadCustomConfig()).Should(HaveActiveService("QE"))
|
|
})
|
|
|
|
It("add UDS", func() {
|
|
cmd := []string{"system", "connection", "add",
|
|
"QA-UDS",
|
|
"unix:///run/podman/podman.sock",
|
|
}
|
|
session := podmanTest.Podman(cmd)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(Exit(0))
|
|
Expect(session.Out.Contents()).Should(BeEmpty())
|
|
// stderr will probably warn (ENOENT or EACCESS) about socket
|
|
// but it's too unreliable to test for.
|
|
|
|
Expect(config.ReadCustomConfig()).Should(VerifyService(
|
|
"QA-UDS",
|
|
"unix:///run/podman/podman.sock",
|
|
"",
|
|
))
|
|
|
|
cmd = []string{"system", "connection", "add",
|
|
"QA-UDS1",
|
|
"--socket-path", "/run/user/podman/podman.sock",
|
|
"unix:///run/podman/podman.sock",
|
|
}
|
|
session = podmanTest.Podman(cmd)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(Exit(0))
|
|
Expect(session.Out.Contents()).Should(BeEmpty())
|
|
|
|
Expect(config.ReadCustomConfig()).Should(HaveActiveService("QA-UDS"))
|
|
Expect(config.ReadCustomConfig()).Should(VerifyService(
|
|
"QA-UDS1",
|
|
"unix:///run/user/podman/podman.sock",
|
|
"",
|
|
))
|
|
})
|
|
|
|
It("add tcp", func() {
|
|
cmd := []string{"system", "connection", "add",
|
|
"QA-TCP",
|
|
"tcp://localhost:8888",
|
|
}
|
|
session := podmanTest.Podman(cmd)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(ExitCleanly())
|
|
Expect(session.Out.Contents()).Should(BeEmpty())
|
|
|
|
Expect(config.ReadCustomConfig()).Should(VerifyService(
|
|
"QA-TCP",
|
|
"tcp://localhost:8888",
|
|
"",
|
|
))
|
|
})
|
|
|
|
It("add to new farm", func() {
|
|
cfg, err := config.ReadCustomConfig()
|
|
Expect(err).ShouldNot(HaveOccurred())
|
|
Expect(cfg.Farms.List).Should(BeEmpty())
|
|
|
|
cmd := []string{"system", "connection", "add",
|
|
"--default",
|
|
"--identity", "~/.ssh/id_rsa",
|
|
"--farm", "farm1",
|
|
"QA",
|
|
"ssh://root@podman.test:2222/run/podman/podman.sock",
|
|
}
|
|
session := podmanTest.Podman(cmd)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(ExitCleanly())
|
|
Expect(session.Out.Contents()).Should(BeEmpty())
|
|
|
|
cfg, err = config.ReadCustomConfig()
|
|
Expect(err).ShouldNot(HaveOccurred())
|
|
Expect(cfg).Should(HaveActiveService("QA"))
|
|
Expect(cfg).Should(VerifyService(
|
|
"QA",
|
|
"ssh://root@podman.test:2222/run/podman/podman.sock",
|
|
"~/.ssh/id_rsa",
|
|
))
|
|
Expect(cfg.Farms.List).Should(HaveKeyWithValue("farm1", []string{"QA"}))
|
|
})
|
|
|
|
It("add to existing farm", func() {
|
|
// create empty farm
|
|
cmd := []string{"farm", "create", "empty-farm"}
|
|
session := podmanTest.Podman(cmd)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(ExitCleanly())
|
|
Expect(session.Out.Contents()).Should(ContainSubstring("Farm \"empty-farm\" created"))
|
|
|
|
cfg, err := config.ReadCustomConfig()
|
|
Expect(err).ShouldNot(HaveOccurred())
|
|
Expect(cfg.Farms.List).Should(HaveKeyWithValue("empty-farm", []string{}))
|
|
|
|
cmd = []string{"system", "connection", "add",
|
|
"--default",
|
|
"--identity", "~/.ssh/id_rsa",
|
|
"--farm", "empty-farm",
|
|
"QA",
|
|
"ssh://root@podman.test:2222/run/podman/podman.sock",
|
|
}
|
|
session = podmanTest.Podman(cmd)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(ExitCleanly())
|
|
Expect(session.Out.Contents()).Should(BeEmpty())
|
|
|
|
cfg, err = config.ReadCustomConfig()
|
|
Expect(err).ShouldNot(HaveOccurred())
|
|
Expect(cfg).Should(HaveActiveService("QA"))
|
|
Expect(cfg).Should(VerifyService(
|
|
"QA",
|
|
"ssh://root@podman.test:2222/run/podman/podman.sock",
|
|
"~/.ssh/id_rsa",
|
|
))
|
|
Expect(cfg.Farms.List).Should(HaveKeyWithValue("empty-farm", []string{"QA"}))
|
|
})
|
|
|
|
It("removing connection should remove from farm also", func() {
|
|
cfg, err := config.ReadCustomConfig()
|
|
Expect(err).ShouldNot(HaveOccurred())
|
|
Expect(cfg.Farms.List).Should(BeEmpty())
|
|
|
|
cmd := []string{"system", "connection", "add",
|
|
"--default",
|
|
"--identity", "~/.ssh/id_rsa",
|
|
"--farm", "farm1",
|
|
"QA",
|
|
"ssh://root@podman.test:2222/run/podman/podman.sock",
|
|
}
|
|
session := podmanTest.Podman(cmd)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(ExitCleanly())
|
|
Expect(session.Out.Contents()).Should(BeEmpty())
|
|
|
|
cfg, err = config.ReadCustomConfig()
|
|
Expect(err).ShouldNot(HaveOccurred())
|
|
Expect(cfg).Should(HaveActiveService("QA"))
|
|
Expect(cfg).Should(VerifyService(
|
|
"QA",
|
|
"ssh://root@podman.test:2222/run/podman/podman.sock",
|
|
"~/.ssh/id_rsa",
|
|
))
|
|
Expect(cfg.Farms.List).Should(HaveKeyWithValue("farm1", []string{"QA"}))
|
|
|
|
// Remove the QA connection
|
|
session = podmanTest.Podman([]string{"system", "connection", "remove", "QA"})
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(ExitCleanly())
|
|
Expect(session.Out.Contents()).Should(BeEmpty())
|
|
|
|
cfg, err = config.ReadCustomConfig()
|
|
Expect(err).ShouldNot(HaveOccurred())
|
|
Expect(cfg.Engine.ActiveService).Should(BeEmpty())
|
|
Expect(cfg.Engine.ServiceDestinations).Should(BeEmpty())
|
|
Expect(cfg.Farms.List).Should(HaveKeyWithValue("farm1", []string{}))
|
|
})
|
|
|
|
It("remove", func() {
|
|
session := podmanTest.Podman([]string{"system", "connection", "add",
|
|
"--default",
|
|
"--identity", "~/.ssh/id_rsa",
|
|
"QA",
|
|
"ssh://root@podman.test:2222/run/podman/podman.sock",
|
|
})
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(ExitCleanly())
|
|
|
|
// two passes to test that removing non-existent connection is not an error
|
|
for i := 0; i < 2; i++ {
|
|
session = podmanTest.Podman([]string{"system", "connection", "remove", "QA"})
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(ExitCleanly())
|
|
Expect(session.Out.Contents()).Should(BeEmpty())
|
|
|
|
cfg, err := config.ReadCustomConfig()
|
|
Expect(err).ShouldNot(HaveOccurred())
|
|
Expect(cfg.Engine.ActiveService).Should(BeEmpty())
|
|
Expect(cfg.Engine.ServiceDestinations).Should(BeEmpty())
|
|
}
|
|
})
|
|
|
|
It("remove --all", func() {
|
|
session := podmanTest.Podman([]string{"system", "connection", "add",
|
|
"--default",
|
|
"--identity", "~/.ssh/id_rsa",
|
|
"QA",
|
|
"ssh://root@podman.test:2222/run/podman/podman.sock",
|
|
})
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(ExitCleanly())
|
|
|
|
session = podmanTest.Podman([]string{"system", "connection", "remove", "--all"})
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(ExitCleanly())
|
|
Expect(session.Out.Contents()).Should(BeEmpty())
|
|
Expect(session.Err.Contents()).Should(BeEmpty())
|
|
|
|
session = podmanTest.Podman([]string{"system", "connection", "list"})
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(ExitCleanly())
|
|
})
|
|
|
|
It("default", func() {
|
|
for _, name := range []string{"devl", "qe"} {
|
|
cmd := []string{"system", "connection", "add",
|
|
"--default",
|
|
"--identity", "~/.ssh/id_rsa",
|
|
name,
|
|
"ssh://root@podman.test:2222/run/podman/podman.sock",
|
|
}
|
|
session := podmanTest.Podman(cmd)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(ExitCleanly())
|
|
}
|
|
|
|
cmd := []string{"system", "connection", "default", "devl"}
|
|
session := podmanTest.Podman(cmd)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(ExitCleanly())
|
|
Expect(session.Out.Contents()).Should(BeEmpty())
|
|
|
|
Expect(config.ReadCustomConfig()).Should(HaveActiveService("devl"))
|
|
|
|
cmd = []string{"system", "connection", "list"}
|
|
session = podmanTest.Podman(cmd)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(ExitCleanly())
|
|
Expect(session.Out).Should(Say("Name *URI *Identity *Default"))
|
|
|
|
cmd = []string{"system", "connection", "list", "--format", "{{.Name}}"}
|
|
session = podmanTest.Podman(cmd)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(ExitCleanly())
|
|
Expect(session.OutputToString()).Should(Equal("devl qe"))
|
|
})
|
|
|
|
It("failed default", func() {
|
|
cmd := []string{"system", "connection", "default", "devl"}
|
|
session := podmanTest.Podman(cmd)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).ShouldNot(ExitCleanly())
|
|
Expect(session.Err).Should(Say("destination is not defined"))
|
|
})
|
|
|
|
It("failed rename", func() {
|
|
cmd := []string{"system", "connection", "rename", "devl", "QE"}
|
|
session := podmanTest.Podman(cmd)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).ShouldNot(ExitCleanly())
|
|
Expect(session.Err).Should(Say("destination is not defined"))
|
|
})
|
|
|
|
It("empty list", func() {
|
|
cmd := []string{"system", "connection", "list"}
|
|
session := podmanTest.Podman(cmd)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).Should(ExitCleanly())
|
|
Expect(session.OutputToStringArray()).Should(HaveLen(1))
|
|
Expect(session.Err.Contents()).Should(BeEmpty())
|
|
})
|
|
})
|
|
|
|
Context("sshd and API services required", func() {
|
|
BeforeEach(func() {
|
|
// These tests are unique in as much as they require podman, podman-remote, systemd and sshd.
|
|
// podman-remote commands will be executed by ginkgo directly.
|
|
SkipIfContainerized("sshd is not available when running in a container")
|
|
SkipIfRemote("connection heuristic requires both podman and podman-remote binaries")
|
|
SkipIfNotRootless(fmt.Sprintf("FIXME: set up ssh keys when root. uid(%d) euid(%d)", os.Getuid(), os.Geteuid()))
|
|
SkipIfSystemdNotRunning("cannot test connection heuristic if systemd is not running")
|
|
SkipIfNotActive("sshd", "cannot test connection heuristic if sshd is not running")
|
|
})
|
|
|
|
It("add ssh:// socket path using connection heuristic", func() {
|
|
u, err := user.Current()
|
|
Expect(err).ShouldNot(HaveOccurred())
|
|
|
|
// Ensure that the remote end uses our built podman
|
|
if os.Getenv("PODMAN_BINARY") == "" {
|
|
err = os.Setenv("PODMAN_BINARY", podmanTest.PodmanBinary)
|
|
Expect(err).ShouldNot(HaveOccurred())
|
|
|
|
defer func() {
|
|
os.Unsetenv("PODMAN_BINARY")
|
|
}()
|
|
}
|
|
|
|
cmd := exec.Command(podmanTest.RemotePodmanBinary,
|
|
"system", "connection", "add",
|
|
"--default",
|
|
"--identity", filepath.Join(u.HomeDir, ".ssh", "id_ed25519"),
|
|
"QA",
|
|
fmt.Sprintf("ssh://%s@localhost", u.Username))
|
|
|
|
session, err := Start(cmd, GinkgoWriter, GinkgoWriter)
|
|
Expect(err).ToNot(HaveOccurred(), fmt.Sprintf("%q failed to execute", podmanTest.RemotePodmanBinary))
|
|
Eventually(session, DefaultWaitTimeout).Should(Exit(0))
|
|
Expect(session.Out.Contents()).Should(BeEmpty())
|
|
Expect(session.Err.Contents()).Should(BeEmpty())
|
|
|
|
cmd = exec.Command(podmanTest.RemotePodmanBinary,
|
|
"--connection", "QA", "ps")
|
|
_, err = Start(cmd, GinkgoWriter, GinkgoWriter)
|
|
Expect(err).ToNot(HaveOccurred())
|
|
|
|
// export the container_host env var and try again
|
|
err = os.Setenv("CONTAINER_HOST", fmt.Sprintf("ssh://%s@localhost", u.Username))
|
|
Expect(err).ToNot(HaveOccurred())
|
|
defer os.Unsetenv("CONTAINER_HOST")
|
|
|
|
cmd = exec.Command(podmanTest.RemotePodmanBinary, "ps")
|
|
_, err = Start(cmd, GinkgoWriter, GinkgoWriter)
|
|
Expect(err).ToNot(HaveOccurred())
|
|
|
|
uri := url.URL{
|
|
Scheme: "ssh",
|
|
User: url.User(u.Username),
|
|
Host: "localhost:22",
|
|
Path: fmt.Sprintf("/run/user/%s/podman/podman.sock", u.Uid),
|
|
}
|
|
|
|
Expect(config.ReadCustomConfig()).Should(HaveActiveService("QA"))
|
|
Expect(config.ReadCustomConfig()).Should(VerifyService(
|
|
"QA",
|
|
uri.String(),
|
|
filepath.Join(u.HomeDir, ".ssh", "id_ed25519"),
|
|
))
|
|
})
|
|
})
|
|
})
|