mirror of
https://github.com/containers/podman.git
synced 2025-07-03 17:27:18 +08:00
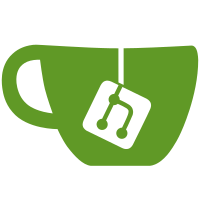
This will make it easier to structure the API, at the cost of making it a bit more opaque about which parts of PodmanExecOptions are implemented where. Should not change behavior. Signed-off-by: Miloslav Trmač <mitr@redhat.com>
52 lines
1.1 KiB
Go
52 lines
1.1 KiB
Go
package utils_test
|
|
|
|
import (
|
|
"io"
|
|
"os/exec"
|
|
"strings"
|
|
"testing"
|
|
|
|
. "github.com/containers/podman/v5/test/utils"
|
|
. "github.com/onsi/ginkgo/v2"
|
|
. "github.com/onsi/gomega"
|
|
"github.com/onsi/gomega/gexec"
|
|
)
|
|
|
|
var FakeOutputs map[string][]string
|
|
var GoechoPath = "../goecho/goecho"
|
|
|
|
type FakePodmanTest struct {
|
|
PodmanTest
|
|
}
|
|
|
|
func FakePodmanTestCreate() *FakePodmanTest {
|
|
FakeOutputs = make(map[string][]string)
|
|
p := &FakePodmanTest{
|
|
PodmanTest: PodmanTest{
|
|
PodmanBinary: GoechoPath,
|
|
},
|
|
}
|
|
|
|
p.PodmanMakeOptions = p.makeOptions
|
|
return p
|
|
}
|
|
|
|
func (p *FakePodmanTest) makeOptions(args []string, options PodmanExecOptions) []string {
|
|
return FakeOutputs[strings.Join(args, " ")]
|
|
}
|
|
|
|
func StartFakeCmdSession(args []string) *PodmanSession {
|
|
var outWriter, errWriter io.Writer
|
|
command := exec.Command(GoechoPath, args...)
|
|
session, err := gexec.Start(command, outWriter, errWriter)
|
|
if err != nil {
|
|
GinkgoWriter.Println(err)
|
|
}
|
|
return &PodmanSession{session}
|
|
}
|
|
|
|
func TestUtils(t *testing.T) {
|
|
RegisterFailHandler(Fail)
|
|
RunSpecs(t, "Unit test for test utils package")
|
|
}
|