mirror of
https://github.com/containers/podman.git
synced 2025-05-17 06:59:07 +08:00
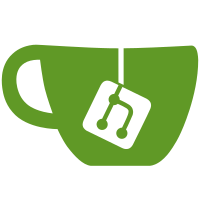
Only in 000-TEMPLATE. I know I need to write more thorough documentation. I choose to defer that. Signed-off-by: Ed Santiago <santiago@redhat.com>
178 lines
6.9 KiB
Bash
178 lines
6.9 KiB
Bash
#!/usr/bin/env bats -*- bats -*-
|
|
#
|
|
# FIXME: short description of the purpose of this module
|
|
#
|
|
# FIXME: copy this file to 'NNN-yourtestname.bats' and edit as needed.
|
|
#
|
|
|
|
load helpers
|
|
|
|
@test "podman subcmd - description of this particular test" {
|
|
args="some sort of argument list"
|
|
run_podman subcmd $args
|
|
assert "$output" == "what we expect" "output from 'podman subcmd $args'"
|
|
|
|
# safename() provides a lower-case string with both the BATS test number
|
|
# and a pseudorandom element. Its use is strongly encouraged for container
|
|
# names, volumes, networks, images, everything, because the test number
|
|
# makes it possible to find leaked elements.
|
|
cname="c-$(safename)"
|
|
|
|
# Run "top" in a detached container with a safe name
|
|
run_podman run -d --name $cname --this-option --that $IMAGE top
|
|
|
|
# A number ("17") as the first run_podman arg means "expect this exit code".
|
|
# By default, run_podman expects success, and will barf on nonzero status.
|
|
run_podman 17 run --this-option --that $IMAGE exit 17
|
|
|
|
# Give a hoot, don't pollute
|
|
run_podman rm -f -t0 $cname
|
|
}
|
|
|
|
# vim: filetype=sh
|
|
|
|
###############################################################################
|
|
#
|
|
# FIXME FIXME FIXME: Most of the time you can cut from here on down.
|
|
# FIXME FIXME FIXME: The above template is probably enough for many tests.
|
|
# FIXME FIXME FIXME:
|
|
# FIXME FIXME FIXME: If you need anything more complicated, read on.
|
|
#
|
|
# FIXME: This is a bloated test template. It provides mostly stuff for you
|
|
# FIXME: to remove, plus stuff for you to base your tests on.
|
|
# FIXME:
|
|
# FIXME: copy this file to 'NNN-yourtestname.bats' and edit as needed.
|
|
# FIXME: Read all FIXMEs, act on them as needed, then remove them.
|
|
# FIXME: test w/ $ PODMAN=./bin/podman bats test/system/NNN-yourtestname.bats
|
|
#
|
|
|
|
load helpers
|
|
|
|
# FIXME: DELETE THESE LINES UNLESS YOU ABSOLUTELY NEED THEM.
|
|
# FIXME: Most tests will not need a custom setup/teardown: they are
|
|
# FIXME: provided by helpers.bash.
|
|
# FIXME: But if you have to do anything special, these give you the
|
|
# FIXME: names of the standard setup/teardown so you can call them
|
|
# FIXME: before or after your own additions.
|
|
function setup() {
|
|
basic_setup
|
|
# FIXME: you almost certainly want to do your own setup _after_ basic.
|
|
}
|
|
function teardown() {
|
|
# FIXME: you almost certainly want to do your own teardown _before_ basic.
|
|
basic_teardown
|
|
}
|
|
|
|
|
|
# FIXME: very basic one-pass example
|
|
@test "podman FOO - description of test" {
|
|
# FIXME: please try to remove this line; that is, try to write tests
|
|
# that will pass as both root and rootless.
|
|
skip_if_rootless "Short explanation of why this doesn't work rootless"
|
|
|
|
# FIXME: template for run commands. Always use 'run_podman'!
|
|
# FIXME: The '?' means 'ignore exit status'; use a number if you
|
|
# FIXME: expect a precise nonzero code, or omit for 0 (usual case).
|
|
# FIXME: NEVER EVER RUN 'podman' DIRECTLY. See helpers.bash for why.
|
|
run_podman '?' run -d $IMAGE sh -c 'prep..; echo READY'
|
|
cid="$output"
|
|
wait_for_ready $cid
|
|
|
|
run_podman logs $cid
|
|
# FIXME: example of dprint. This will trigger if PODMAN_TEST_DEBUG=FOO
|
|
# FIXME: ...or anything that matches the name assigned in the @test line.
|
|
dprint "podman logs $cid -> '$output'"
|
|
assert "$output" == "what are we expecting?" "description of this check"
|
|
|
|
# Clean up
|
|
run_podman rm $cid
|
|
}
|
|
|
|
|
|
# FIXME: another example, this time with a test table loop
|
|
@test "podman FOO - json - template for playing with json output" {
|
|
# FIXME: Define a multiline string in tabular form, using '|' as separator.
|
|
# FIXME: Each row defines one test. Each column (there may be as many as
|
|
# FIXME: you want) is one field. In the case below we have two, a
|
|
# FIXME: json field descriptor and an expected value.
|
|
tests="
|
|
id | [0-9a-f]\\\{64\\\}
|
|
created | [0-9-]\\\+T[0-9:]\\\+\\\.[0-9]\\\+Z
|
|
size | -\\\?[0-9]\\\+
|
|
"
|
|
|
|
# FIXME: Run a basic podman command. We'll check $output multiple times
|
|
# FIXME: in the while loop below.
|
|
run_podman history --format json $IMAGE
|
|
|
|
# FIXME: parse_table is what does all the work, giving us test cases.
|
|
while read field expect; do
|
|
# FIXME: this shows a drawback of BATS and bash: we can't include '|'
|
|
# FIXME: in the table, but we need to because some images don't
|
|
# FIXME: have a CID. So, yeah, this is ugly -- but rare.
|
|
if [ "$field" = "id" ]; then expect="$expect\|<missing>";fi
|
|
|
|
# output is an array of dicts; check each one
|
|
count=$(echo "$output" | jq '. | length')
|
|
i=0
|
|
while [ $i -lt $count ]; do
|
|
actual=$(echo "$output" | jq -r ".[$i].$field")
|
|
# FIXME: please be sure to note the third field!
|
|
# FIXME: that's the test name. Make it something useful! Include
|
|
# FIXME: loop variables whenever possible. Don't just say "my test"
|
|
assert "$actual" =~ "$expect\$" "jq .[$i].$field"
|
|
i=$(expr $i + 1)
|
|
done
|
|
done < <(parse_table "$tests")
|
|
}
|
|
|
|
# Whenever possible, please add the ci:parallel tag to new tests,
|
|
# not only for speed but for stress testing.
|
|
#
|
|
# Some test files have '# bats file_tags=ci:parallel' at the top.
|
|
# ^^^^---- instead of test_tags on each test
|
|
# This indicates that ALL tests in the file run parallel, and if
|
|
# you add new tests, you need to guarantee that your new test
|
|
# will also run parallel-safe.
|
|
#
|
|
# Below is an example of what NOT to do when enabling parallel tests.
|
|
#
|
|
# bats test_tags=ci:parallel
|
|
@test "this test is completely broken in parallel" {
|
|
# Never use "--name HARDCODED". Define 'cname=c-$(safename)' instead:
|
|
# cname="c-$(safename)"
|
|
# run_podman --name $cname ...
|
|
# Not only does that guarantee uniqueness, it also gives the test number
|
|
# in the name, so if there's a leak (at end of tests) it will be possible
|
|
# to identify the culprit.
|
|
run_podman --name mycontainer $IMAGE top
|
|
|
|
# Same thing for build and -t:
|
|
# imgname="i-$(safename)"
|
|
# run_podman build -t $imgname ...
|
|
# Ed's convention is "c-$(safename)" for containers, "i-" images,
|
|
# "n-" namespaces, "p-" pods, "v-" volumes, "z-" zebras.
|
|
# When there are multiple objects needed, it is slightly easier
|
|
# to differentiate in front rather than at the tail:
|
|
# yes: c1="ctr1-$(safename)"
|
|
# c2="ctr2-$(safename)"
|
|
# no: c1="ctr-$(safename)-1"
|
|
run_podman build -t myimage ...
|
|
|
|
# Never assume exact output from podman ps! Many other containers may be running.
|
|
run_podman ps
|
|
assert "$output" = "mycontainer"
|
|
|
|
# Never use "-l". It is meaningless when other processes are running.
|
|
run_podman container inspect -l
|
|
|
|
# "userns=auto" can NEVER be parallelized: multiple jobs running
|
|
# at once will cause "not enough unused IDs in user namespace"
|
|
run_podman run --userns=auto ....
|
|
|
|
# Never 'rm -a'!!! OMG like seriously just don't.
|
|
run_podman rm -f -a
|
|
}
|
|
|
|
# vim: filetype=sh
|