mirror of
https://github.com/containers/podman.git
synced 2025-05-17 06:59:07 +08:00
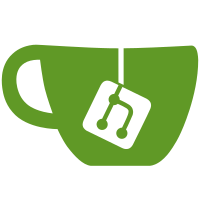
The backstory for this is that runc 1.2 (opencontainers/runc#3967) fixed a long-standing bug in our mount flag handling (a bug that crun still has). Before runc 1.2, when dealing with locked mount flags that user namespaced containers cannot clear, trying to explicitly clearing locked flags (like rw clearing MS_RDONLY) would silently ignore the rw flag in most cases and would result in a read-only mount. This is obviously not what the user expects. What runc 1.2 did is that it made it so that passing clearing flags like rw would always result in an attempt to clear the flag (which was not the case before), and would (in all cases) explicitly return an error if we try to clear locking flags. (This also let us finally fix a bunch of other long-standing issues with locked mount flags causing seemingly spurious errors). The problem is that podman sets rw on all mounts by default (even if the user doesn't specify anything). This is actually a no-op in runc 1.1 and crun because of a bug in how clearing flags were handled (rw is the absence of MS_RDONLY but until runc 1.2 we didn't correctly track clearing flags like that, meaning that rw would literally be handled as if it were not set at all by users) but in runc 1.2 leads to unfortunate breakages and a subtle change in behaviour (before, a ro mount being bind-mounted into a container would also be ro -- though due to the above bug even setting rw explicitly would result in ro in most cases -- but with runc 1.2 the mount will always be rw even if the user didn't explicitly request it which most users would find surprising). By the way, this "always set rw" behaviour is a departure from Docker and it is not necesssary. Signed-off-by: rcmadhankumar <madhankumar.chellamuthu@suse.com>
235 lines
7.3 KiB
Go
235 lines
7.3 KiB
Go
package util
|
|
|
|
import (
|
|
"errors"
|
|
"fmt"
|
|
"strings"
|
|
|
|
"github.com/containers/podman/v5/libpod/define"
|
|
"github.com/containers/podman/v5/pkg/rootless"
|
|
)
|
|
|
|
var (
|
|
// ErrBadMntOption indicates that an invalid mount option was passed.
|
|
ErrBadMntOption = errors.New("invalid mount option")
|
|
// ErrDupeMntOption indicates that a duplicate mount option was passed.
|
|
ErrDupeMntOption = errors.New("duplicate mount option passed")
|
|
)
|
|
|
|
type defaultMountOptions struct {
|
|
noexec bool
|
|
nosuid bool
|
|
nodev bool
|
|
}
|
|
|
|
type getDefaultMountOptionsFn func(path string) (defaultMountOptions, error)
|
|
|
|
// ProcessOptions parses the options for a bind or tmpfs mount and ensures that
|
|
// they are sensible and follow convention. The isTmpfs variable controls
|
|
// whether extra, tmpfs-specific options will be allowed.
|
|
// The sourcePath variable, if not empty, contains a bind mount source.
|
|
func ProcessOptions(options []string, isTmpfs bool, sourcePath string) ([]string, error) {
|
|
return processOptionsInternal(options, isTmpfs, sourcePath, getDefaultMountOptions)
|
|
}
|
|
|
|
func processOptionsInternal(options []string, isTmpfs bool, sourcePath string, getDefaultMountOptions getDefaultMountOptionsFn) ([]string, error) {
|
|
var (
|
|
foundWrite, foundSize, foundProp, foundMode, foundExec, foundSuid, foundDev, foundCopyUp, foundBind, foundZ, foundU, foundOverlay, foundIdmap, foundCopy, foundNoSwap, foundNoDereference bool
|
|
)
|
|
|
|
recursiveBind := true
|
|
|
|
newOptions := make([]string, 0, len(options))
|
|
for _, opt := range options {
|
|
// Some options have parameters - size, mode
|
|
key, _, _ := strings.Cut(opt, "=")
|
|
|
|
// add advanced options such as upperdir=/path and workdir=/path, when overlay is specified
|
|
if foundOverlay {
|
|
if strings.Contains(opt, "upperdir") {
|
|
newOptions = append(newOptions, opt)
|
|
continue
|
|
}
|
|
if strings.Contains(opt, "workdir") {
|
|
newOptions = append(newOptions, opt)
|
|
continue
|
|
}
|
|
}
|
|
if strings.HasPrefix(key, "subpath") {
|
|
newOptions = append(newOptions, opt)
|
|
continue
|
|
}
|
|
if strings.HasPrefix(key, "idmap") {
|
|
if foundIdmap {
|
|
return nil, fmt.Errorf("the 'idmap' option can only be set once: %w", ErrDupeMntOption)
|
|
}
|
|
foundIdmap = true
|
|
newOptions = append(newOptions, opt)
|
|
continue
|
|
}
|
|
|
|
switch key {
|
|
case "copy", "nocopy":
|
|
if foundCopy {
|
|
return nil, fmt.Errorf("only one of 'nocopy' and 'copy' can be used: %w", ErrDupeMntOption)
|
|
}
|
|
foundCopy = true
|
|
case "O":
|
|
foundOverlay = true
|
|
case "volume-opt":
|
|
// Volume-opt should be relayed and processed by driver.
|
|
newOptions = append(newOptions, opt)
|
|
case "exec", "noexec":
|
|
if foundExec {
|
|
return nil, fmt.Errorf("only one of 'noexec' and 'exec' can be used: %w", ErrDupeMntOption)
|
|
}
|
|
foundExec = true
|
|
case "suid", "nosuid":
|
|
if foundSuid {
|
|
return nil, fmt.Errorf("only one of 'nosuid' and 'suid' can be used: %w", ErrDupeMntOption)
|
|
}
|
|
foundSuid = true
|
|
case "nodev", "dev":
|
|
if foundDev {
|
|
return nil, fmt.Errorf("only one of 'nodev' and 'dev' can be used: %w", ErrDupeMntOption)
|
|
}
|
|
foundDev = true
|
|
case "rw", "ro":
|
|
if foundWrite {
|
|
return nil, fmt.Errorf("only one of 'rw' and 'ro' can be used: %w", ErrDupeMntOption)
|
|
}
|
|
foundWrite = true
|
|
case "private", "rprivate", "slave", "rslave", "shared", "rshared", "unbindable", "runbindable":
|
|
if foundProp {
|
|
return nil, fmt.Errorf("only one root propagation mode can be used: %w", ErrDupeMntOption)
|
|
}
|
|
foundProp = true
|
|
case "size":
|
|
if !isTmpfs {
|
|
return nil, fmt.Errorf("the 'size' option is only allowed with tmpfs mounts: %w", ErrBadMntOption)
|
|
}
|
|
if foundSize {
|
|
return nil, fmt.Errorf("only one tmpfs size can be specified: %w", ErrDupeMntOption)
|
|
}
|
|
foundSize = true
|
|
case "mode":
|
|
if !isTmpfs {
|
|
return nil, fmt.Errorf("the 'mode' option is only allowed with tmpfs mounts: %w", ErrBadMntOption)
|
|
}
|
|
if foundMode {
|
|
return nil, fmt.Errorf("only one tmpfs mode can be specified: %w", ErrDupeMntOption)
|
|
}
|
|
foundMode = true
|
|
case "tmpcopyup":
|
|
if !isTmpfs {
|
|
return nil, fmt.Errorf("the 'tmpcopyup' option is only allowed with tmpfs mounts: %w", ErrBadMntOption)
|
|
}
|
|
if foundCopyUp {
|
|
return nil, fmt.Errorf("the 'tmpcopyup' or 'notmpcopyup' option can only be set once: %w", ErrDupeMntOption)
|
|
}
|
|
foundCopyUp = true
|
|
case "consistency":
|
|
// Often used on MACs and mistakenly on Linux platforms.
|
|
// Since Docker ignores this option so shall we.
|
|
continue
|
|
case "notmpcopyup":
|
|
if !isTmpfs {
|
|
return nil, fmt.Errorf("the 'notmpcopyup' option is only allowed with tmpfs mounts: %w", ErrBadMntOption)
|
|
}
|
|
if foundCopyUp {
|
|
return nil, fmt.Errorf("the 'tmpcopyup' or 'notmpcopyup' option can only be set once: %w", ErrDupeMntOption)
|
|
}
|
|
foundCopyUp = true
|
|
// do not propagate notmpcopyup to the OCI runtime
|
|
continue
|
|
case "noswap":
|
|
|
|
if !isTmpfs {
|
|
return nil, fmt.Errorf("the 'noswap' option is only allowed with tmpfs mounts: %w", ErrBadMntOption)
|
|
}
|
|
if rootless.IsRootless() {
|
|
return nil, fmt.Errorf("the 'noswap' option is only allowed with rootful tmpfs mounts: %w", ErrBadMntOption)
|
|
}
|
|
if foundNoSwap {
|
|
return nil, fmt.Errorf("the 'tmpswap' option can only be set once: %w", ErrDupeMntOption)
|
|
}
|
|
foundNoSwap = true
|
|
newOptions = append(newOptions, opt)
|
|
continue
|
|
case "no-dereference":
|
|
if foundNoDereference {
|
|
return nil, fmt.Errorf("the 'no-dereference' option can only be set once: %w", ErrDupeMntOption)
|
|
}
|
|
foundNoDereference = true
|
|
case define.TypeBind:
|
|
recursiveBind = false
|
|
fallthrough
|
|
case "rbind":
|
|
if isTmpfs {
|
|
return nil, fmt.Errorf("the 'bind' and 'rbind' options are not allowed with tmpfs mounts: %w", ErrBadMntOption)
|
|
}
|
|
if foundBind {
|
|
return nil, fmt.Errorf("only one of 'rbind' and 'bind' can be used: %w", ErrDupeMntOption)
|
|
}
|
|
foundBind = true
|
|
case "z", "Z":
|
|
if isTmpfs {
|
|
return nil, fmt.Errorf("the 'z' and 'Z' options are not allowed with tmpfs mounts: %w", ErrBadMntOption)
|
|
}
|
|
if foundZ {
|
|
return nil, fmt.Errorf("only one of 'z' and 'Z' can be used: %w", ErrDupeMntOption)
|
|
}
|
|
foundZ = true
|
|
case "U":
|
|
if foundU {
|
|
return nil, fmt.Errorf("the 'U' option can only be set once: %w", ErrDupeMntOption)
|
|
}
|
|
foundU = true
|
|
default:
|
|
return nil, fmt.Errorf("unknown mount option %q: %w", opt, ErrBadMntOption)
|
|
}
|
|
newOptions = append(newOptions, opt)
|
|
}
|
|
|
|
if !foundProp {
|
|
if recursiveBind {
|
|
newOptions = append(newOptions, "rprivate")
|
|
} else {
|
|
newOptions = append(newOptions, "private")
|
|
}
|
|
}
|
|
defaults, err := getDefaultMountOptions(sourcePath)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
if !foundExec && defaults.noexec {
|
|
newOptions = append(newOptions, "noexec")
|
|
}
|
|
if !foundSuid && defaults.nosuid {
|
|
newOptions = append(newOptions, "nosuid")
|
|
}
|
|
if !foundDev && defaults.nodev {
|
|
newOptions = append(newOptions, "nodev")
|
|
}
|
|
if isTmpfs && !foundCopyUp {
|
|
newOptions = append(newOptions, "tmpcopyup")
|
|
}
|
|
if !isTmpfs && !foundBind {
|
|
newOptions = append(newOptions, "rbind")
|
|
}
|
|
|
|
return newOptions, nil
|
|
}
|
|
|
|
func ParseDriverOpts(option string) (string, string, error) {
|
|
_, val, hasVal := strings.Cut(option, "=")
|
|
if !hasVal {
|
|
return "", "", fmt.Errorf("cannot parse driver opts: %w", ErrBadMntOption)
|
|
}
|
|
optKey, optVal, hasOptVal := strings.Cut(val, "=")
|
|
if !hasOptVal {
|
|
return "", "", fmt.Errorf("cannot parse driver opts: %w", ErrBadMntOption)
|
|
}
|
|
return optKey, optVal, nil
|
|
}
|