mirror of
https://github.com/containers/podman.git
synced 2025-05-17 06:59:07 +08:00
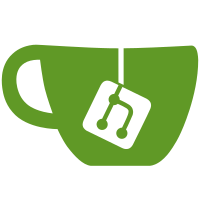
The new golangci-lint version 1.60.1 has problems with typecheck when linting remote files. We have certain pakcages that should never be inlcuded in remote but the typecheck tries to compile all of them but this never works and it seems to ignore the exclude files we gave it. To fix this the proper way is to mark all packages we only use locally with !remote tags. This is a bit ugly but more correct. I also moved the DecodeChanges() code around as it is called from the client so the handles package which should only be remote doesn't really fit anyway. Signed-off-by: Paul Holzinger <pholzing@redhat.com>
35 lines
1.2 KiB
Go
35 lines
1.2 KiB
Go
package util
|
|
|
|
import (
|
|
"strings"
|
|
"unicode"
|
|
)
|
|
|
|
// DecodeChanges reads one or more changes from a slice and cleans them up,
|
|
// since what we've advertised as being acceptable in the past isn't really.
|
|
func DecodeChanges(changes []string) []string {
|
|
result := make([]string, 0, len(changes))
|
|
for _, possiblyMultilineChange := range changes {
|
|
for _, change := range strings.Split(possiblyMultilineChange, "\n") {
|
|
// In particular, we document that we accept values
|
|
// like "CMD=/bin/sh", which is not valid Dockerfile
|
|
// syntax, so we can't just pass such a value directly
|
|
// to a parser that's going to rightfully reject it.
|
|
// If we trim the string of whitespace at both ends,
|
|
// and the first occurrence of "=" is before the first
|
|
// whitespace, replace that "=" with whitespace.
|
|
change = strings.TrimSpace(change)
|
|
if change == "" {
|
|
continue
|
|
}
|
|
firstEqualIndex := strings.Index(change, "=")
|
|
firstSpaceIndex := strings.IndexFunc(change, unicode.IsSpace)
|
|
if firstEqualIndex != -1 && (firstSpaceIndex == -1 || firstEqualIndex < firstSpaceIndex) {
|
|
change = change[:firstEqualIndex] + " " + change[firstEqualIndex+1:]
|
|
}
|
|
result = append(result, change)
|
|
}
|
|
}
|
|
return result
|
|
}
|