mirror of
https://github.com/containers/podman.git
synced 2025-05-18 07:36:21 +08:00
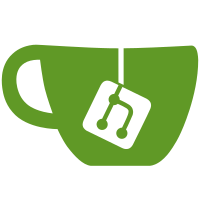
The `podman system prune` command is able to remove build containers that were created during the build, but were not removed because the build terminated unexpectedly. By default, build containers are not removed to prevent interference with builds in progress. Use the **--build** flag when running the command to remove build containers as well. Fixes: https://issues.redhat.com/browse/RHEL-62009 Signed-off-by: Jan Rodák <hony.com@seznam.cz>
62 lines
2.4 KiB
Go
62 lines
2.4 KiB
Go
package tunnel
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
|
|
"github.com/containers/podman/v5/libpod/define"
|
|
"github.com/containers/podman/v5/pkg/bindings/system"
|
|
"github.com/containers/podman/v5/pkg/domain/entities"
|
|
)
|
|
|
|
func (ic *ContainerEngine) Info(ctx context.Context) (*define.Info, error) {
|
|
return system.Info(ic.ClientCtx, nil)
|
|
}
|
|
|
|
func (ic *ContainerEngine) SetupRootless(_ context.Context, noMoveProcess bool, cgroupMode string) error {
|
|
panic(errors.New("rootless engine mode is not supported when tunneling"))
|
|
}
|
|
|
|
// SystemPrune prunes unused data from the system.
|
|
func (ic *ContainerEngine) SystemPrune(ctx context.Context, opts entities.SystemPruneOptions) (*entities.SystemPruneReport, error) {
|
|
options := new(system.PruneOptions).WithAll(opts.All).WithVolumes(opts.Volume).WithFilters(opts.Filters).WithExternal(opts.External).WithBuild(opts.Build)
|
|
return system.Prune(ic.ClientCtx, options)
|
|
}
|
|
|
|
func (ic *ContainerEngine) SystemCheck(ctx context.Context, opts entities.SystemCheckOptions) (*entities.SystemCheckReport, error) {
|
|
options := new(system.CheckOptions).WithQuick(opts.Quick).WithRepair(opts.Repair).WithRepairLossy(opts.RepairLossy)
|
|
if opts.UnreferencedLayerMaximumAge != nil {
|
|
duration := *opts.UnreferencedLayerMaximumAge
|
|
options = options.WithUnreferencedLayerMaximumAge(duration.String())
|
|
}
|
|
return system.Check(ic.ClientCtx, options)
|
|
}
|
|
|
|
func (ic *ContainerEngine) Migrate(ctx context.Context, options entities.SystemMigrateOptions) error {
|
|
return errors.New("runtime migration is not supported on remote clients")
|
|
}
|
|
|
|
func (ic *ContainerEngine) Renumber(ctx context.Context) error {
|
|
return errors.New("lock renumbering is not supported on remote clients")
|
|
}
|
|
|
|
func (ic *ContainerEngine) Reset(ctx context.Context) error {
|
|
return errors.New("system reset is not supported on remote clients")
|
|
}
|
|
|
|
func (ic *ContainerEngine) SystemDf(ctx context.Context, options entities.SystemDfOptions) (*entities.SystemDfReport, error) {
|
|
return system.DiskUsage(ic.ClientCtx, nil)
|
|
}
|
|
|
|
func (ic *ContainerEngine) Unshare(ctx context.Context, args []string, options entities.SystemUnshareOptions) error {
|
|
return errors.New("unshare is not supported on remote clients")
|
|
}
|
|
|
|
func (ic *ContainerEngine) Version(ctx context.Context) (*entities.SystemVersionReport, error) {
|
|
return system.Version(ic.ClientCtx, nil)
|
|
}
|
|
|
|
func (ic *ContainerEngine) Locks(ctx context.Context) (*entities.LocksReport, error) {
|
|
return nil, errors.New("locks is not supported on remote clients")
|
|
}
|