mirror of
https://github.com/containers/podman.git
synced 2025-05-17 15:18:43 +08:00
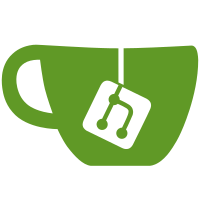
Shuffle the code around to eliminate "unused" warnings when linting with various GOOS and build tags. The only change in functionality should be that now NewEventer returns ErrNoJournaldLogging (rather than "unknown event logger type") on freebsd when journald is requested. Signed-off-by: Kir Kolyshkin <kolyshkin@gmail.com>
43 lines
1.0 KiB
Go
43 lines
1.0 KiB
Go
//go:build linux || freebsd
|
|
|
|
package events
|
|
|
|
import (
|
|
"encoding/json"
|
|
"fmt"
|
|
"strings"
|
|
|
|
"github.com/sirupsen/logrus"
|
|
)
|
|
|
|
// NewEventer creates an eventer based on the eventer type
|
|
func NewEventer(options EventerOptions) (Eventer, error) {
|
|
logrus.Debugf("Initializing event backend %s", options.EventerType)
|
|
switch EventerType(strings.ToLower(options.EventerType)) {
|
|
case Journald:
|
|
return newJournalDEventer(options)
|
|
case LogFile:
|
|
return newLogFileEventer(options)
|
|
case Null:
|
|
return newNullEventer(), nil
|
|
default:
|
|
return nil, fmt.Errorf("unknown event logger type: %s", strings.ToLower(options.EventerType))
|
|
}
|
|
}
|
|
|
|
// newEventFromJSONString takes stringified json and converts
|
|
// it to an event
|
|
func newEventFromJSONString(event string) (*Event, error) {
|
|
e := new(Event)
|
|
if err := json.Unmarshal([]byte(event), e); err != nil {
|
|
return nil, err
|
|
}
|
|
return e, nil
|
|
}
|
|
|
|
// newNullEventer returns a new null eventer. You should only do this for
|
|
// the purposes of internal libpod testing.
|
|
func newNullEventer() Eventer {
|
|
return EventToNull{}
|
|
}
|