mirror of
https://github.com/containers/podman.git
synced 2025-07-04 18:27:33 +08:00
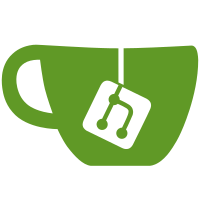
create the /etc/passwd and /etc/group files before any user/group lookup so that the entries added dynamically are found by --user. As a side effect, do not automatically create the group with same value as the uid when not specified, since it is expected to run with gid=0. Closes: https://github.com/containers/podman/issues/25805 Signed-off-by: Giuseppe Scrivano <gscrivan@redhat.com>
63 lines
1.3 KiB
Go
63 lines
1.3 KiB
Go
//go:build !remote
|
|
|
|
package libpod
|
|
|
|
import (
|
|
"testing"
|
|
|
|
spec "github.com/opencontainers/runtime-spec/specs-go"
|
|
"github.com/stretchr/testify/assert"
|
|
)
|
|
|
|
func TestGenerateUserPasswdEntry(t *testing.T) {
|
|
c := Container{
|
|
config: &ContainerConfig{
|
|
Spec: &spec.Spec{},
|
|
ContainerSecurityConfig: ContainerSecurityConfig{
|
|
User: "123456:456789",
|
|
},
|
|
},
|
|
state: &ContainerState{
|
|
Mountpoint: "/does/not/exist/tmp/",
|
|
},
|
|
}
|
|
user, err := c.generateUserPasswdEntry(0)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
assert.Equal(t, user, "123456:*:123456:456789:container user:/:/bin/sh\n")
|
|
|
|
c.config.User = "567890"
|
|
user, err = c.generateUserPasswdEntry(0)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
assert.Equal(t, user, "567890:*:567890:0:container user:/:/bin/sh\n")
|
|
}
|
|
|
|
func TestGenerateUserGroupEntry(t *testing.T) {
|
|
c := Container{
|
|
config: &ContainerConfig{
|
|
Spec: &spec.Spec{},
|
|
ContainerSecurityConfig: ContainerSecurityConfig{
|
|
User: "123456:456789",
|
|
},
|
|
},
|
|
state: &ContainerState{
|
|
Mountpoint: "/does/not/exist/tmp/",
|
|
},
|
|
}
|
|
group, err := c.generateUserGroupEntry(-1)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
assert.Equal(t, group, "456789:x:456789:123456\n")
|
|
|
|
c.config.User = "567890"
|
|
group, err = c.generateUserGroupEntry(-1)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
assert.Equal(t, group, "0:x:0:567890\n")
|
|
}
|