mirror of
https://github.com/containers/podman.git
synced 2025-07-03 01:08:02 +08:00
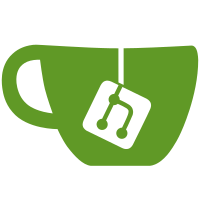
First: fix podman-registry script so it preserves the initial $PODMAN, so all subsequent invocations of ps, logs, and stop will use the same binary and arguments. Until now we've handled this by requiring that our caller manage $PODMAN (and keep it the same), but that's just wrong. Next, simplify the golang interface: move the $PODMAN setting into registry.go, instead of requiring e2e callers to set it. (This could use some work: the local/remote conditional is icky). IMPORTANT: To prevent registry.go from using the wrong podman binary, the Start() call is gone. Only StartWithOptions() is valid now. And, minor cleanup: comments, and add an actual error-message check Reason for this PR is a recurring flake, #18355, whose multiple failure modes I truly can't understand. I don't think this PR is going to fix it, but this is still necessary work. Signed-off-by: Ed Santiago <santiago@redhat.com>
45 lines
1.0 KiB
Go
45 lines
1.0 KiB
Go
package registry
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/hashicorp/go-multierror"
|
|
"github.com/stretchr/testify/assert"
|
|
"github.com/stretchr/testify/require"
|
|
)
|
|
|
|
func TestStartAndStopMultipleRegistries(t *testing.T) {
|
|
binary = "../podman-registry"
|
|
|
|
registries := []*Registry{}
|
|
|
|
registryOptions := &Options{
|
|
PodmanPath: "../../bin/podman",
|
|
}
|
|
|
|
// Start registries.
|
|
var errors *multierror.Error
|
|
for i := 0; i < 3; i++ {
|
|
reg, err := StartWithOptions(registryOptions)
|
|
if err != nil {
|
|
errors = multierror.Append(errors, err)
|
|
continue
|
|
}
|
|
assert.True(t, len(reg.Image) > 0)
|
|
assert.True(t, len(reg.User) > 0)
|
|
assert.True(t, len(reg.Password) > 0)
|
|
assert.True(t, len(reg.Port) > 0)
|
|
registries = append(registries, reg)
|
|
}
|
|
|
|
// Stop registries.
|
|
for _, reg := range registries {
|
|
// Make sure we can stop it properly.
|
|
errors = multierror.Append(errors, reg.Stop())
|
|
// Stopping an already stopped registry is fine as well.
|
|
errors = multierror.Append(errors, reg.Stop())
|
|
}
|
|
|
|
require.NoError(t, errors.ErrorOrNil())
|
|
}
|