mirror of
https://github.com/jeertmans/manim-slides.git
synced 2025-05-22 04:56:24 +08:00
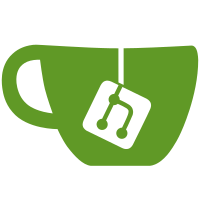
* wip: rewrite player * wip(cli): new player * wip(player): allow to close * Auto stash before merge of "rewrite-player" and "origin/rewrite-player" * feat(cli): new player * chore(docs): document changes * feat(cli): add info window
81 lines
2.1 KiB
Python
81 lines
2.1 KiB
Python
import hashlib
|
|
import subprocess
|
|
import tempfile
|
|
from pathlib import Path
|
|
from typing import List
|
|
|
|
from .manim import FFMPEG_BIN, logger
|
|
|
|
|
|
def concatenate_video_files(files: List[Path], dest: Path) -> None:
|
|
"""
|
|
Concatenate multiple video files into one.
|
|
"""
|
|
|
|
f = tempfile.NamedTemporaryFile(mode="w", delete=False)
|
|
f.writelines(f"file '{path.absolute()}'\n" for path in files)
|
|
f.close()
|
|
|
|
command: List[str] = [
|
|
str(FFMPEG_BIN),
|
|
"-f",
|
|
"concat",
|
|
"-safe",
|
|
"0",
|
|
"-i",
|
|
f.name,
|
|
"-c",
|
|
"copy",
|
|
str(dest),
|
|
"-y",
|
|
]
|
|
logger.debug(" ".join(command))
|
|
process = subprocess.Popen(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
|
|
output, error = process.communicate()
|
|
|
|
if output:
|
|
logger.debug(output.decode())
|
|
|
|
if error:
|
|
logger.debug(error.decode())
|
|
|
|
if not dest.exists():
|
|
raise ValueError(
|
|
"could not properly concatenate files, use `-v DEBUG` for more details"
|
|
)
|
|
|
|
|
|
def merge_basenames(files: List[Path]) -> Path:
|
|
"""
|
|
Merge multiple filenames by concatenating basenames.
|
|
"""
|
|
|
|
dirname: Path = files[0].parent
|
|
ext = files[0].suffix
|
|
|
|
basenames = list(file.stem for file in files)
|
|
|
|
basenames_str = ",".join(f"{len(b)}:{b}" for b in basenames)
|
|
|
|
# We use hashes to prevent too-long filenames, see issue #123:
|
|
# https://github.com/jeertmans/manim-slides/issues/123
|
|
basename = hashlib.sha256(basenames_str.encode()).hexdigest()
|
|
|
|
logger.debug(f"Generated a new basename for basenames: {basenames} -> '{basename}'")
|
|
|
|
return dirname.joinpath(basename + ext)
|
|
|
|
|
|
def reverse_video_file(src: Path, dst: Path) -> None:
|
|
"""Reverses a video file, writting the result to `dst`."""
|
|
command = [str(FFMPEG_BIN), "-y", "-i", str(src), "-vf", "reverse", str(dst)]
|
|
logger.debug(" ".join(command))
|
|
process = subprocess.Popen(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
|
|
output, error = process.communicate()
|
|
|
|
if output:
|
|
logger.debug(output.decode())
|
|
|
|
if error:
|
|
logger.debug(error.decode())
|