mirror of
https://github.com/jeertmans/manim-slides.git
synced 2025-05-20 03:57:38 +08:00
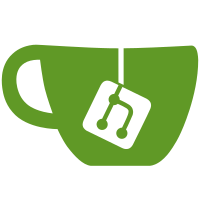
This PR refactors a lot of the code in order to have a better organized codebase. If will that I needed to create a second level of submodules, to better distinguish the different parts of this project.
72 lines
1.6 KiB
Python
72 lines
1.6 KiB
Python
"""Slides module with logic to either import ManimCE or ManimGL."""
|
|
|
|
__all__ = (
|
|
"MANIM",
|
|
"MANIMGL",
|
|
"API_NAME",
|
|
"Slide",
|
|
"ThreeDSlide",
|
|
)
|
|
|
|
|
|
import os
|
|
import sys
|
|
|
|
|
|
class ManimApiNotFoundError(ImportError):
|
|
"""Error raised if specified manim API could be imported."""
|
|
|
|
_msg = "Could not import the specified manim API: `{api}`."
|
|
|
|
def __init__(self, api: str) -> None:
|
|
super().__init__(self._msg.format(api=api))
|
|
|
|
|
|
API_NAMES = {
|
|
"manim": "manim",
|
|
"manimce": "manim",
|
|
"manimlib": "manimlib",
|
|
"manimgl": "manimlib",
|
|
}
|
|
"""Allowed values for API."""
|
|
|
|
MANIM_API: str = "MANIM_API"
|
|
"""API environ variable name."""
|
|
FORCE_MANIM_API: str = "FORCE_" + MANIM_API
|
|
"""FORCE API environ variable name."""
|
|
|
|
API: str = os.environ.get(MANIM_API, "manim").lower()
|
|
|
|
|
|
if API not in API_NAMES:
|
|
raise ImportError(
|
|
f"Specified MANIM_API={API!r} is not in valid options: " f"{API_NAMES}",
|
|
)
|
|
|
|
API_NAME = API_NAMES[API]
|
|
|
|
if not os.environ.get(FORCE_MANIM_API):
|
|
if "manim" in sys.modules:
|
|
API_NAME = "manim"
|
|
elif "manimlib" in sys.modules:
|
|
API_NAME = "manimlib"
|
|
|
|
MANIM: bool = API_NAME == "manim"
|
|
MANIMGL: bool = API_NAME == "manimlib"
|
|
|
|
if MANIM:
|
|
try:
|
|
from .manim import Slide, ThreeDSlide
|
|
except ImportError as e:
|
|
raise ManimApiNotFoundError("manim") from e
|
|
elif MANIMGL:
|
|
try:
|
|
from .manimlib import Slide, ThreeDSlide
|
|
except ImportError as e:
|
|
raise ManimApiNotFoundError("manimlib") from e
|
|
else:
|
|
raise ValueError(
|
|
"This error should never occur. "
|
|
"Please report an issue on GitHub if you encounter it."
|
|
)
|