mirror of
https://github.com/jeertmans/manim-slides.git
synced 2025-05-20 12:05:56 +08:00
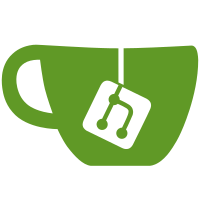
* fix(lib/cli): relative paths, empty slides, and tests This fixes two issues: 1. Empty slides are now reported as error, to prevent indexing error; 2. Changing the folder path will now produce an absolute path to slides, which was not the case before and would lead to a "file does not exist error". A few tests were also added to cover those * fix(lib): fix from_file, remove useless field, and more * chore(tests): remove print * [pre-commit.ci] auto fixes from pre-commit.com hooks for more information, see https://pre-commit.ci --------- Co-authored-by: pre-commit-ci[bot] <66853113+pre-commit-ci[bot]@users.noreply.github.com>
104 lines
2.6 KiB
Python
104 lines
2.6 KiB
Python
import random
|
|
import string
|
|
import tempfile
|
|
from pathlib import Path
|
|
from typing import Any, Generator, List
|
|
|
|
import pytest
|
|
from pydantic import ValidationError
|
|
|
|
from manim_slides.config import (
|
|
Key,
|
|
PresentationConfig,
|
|
SlideConfig,
|
|
SlideType,
|
|
merge_basenames,
|
|
)
|
|
|
|
|
|
def random_path(
|
|
length: int = 20,
|
|
dirname: Path = Path("./media/videos/example"),
|
|
suffix: str = ".mp4",
|
|
touch: bool = False,
|
|
) -> Path:
|
|
basename = "".join(random.choices(string.ascii_letters, k=length))
|
|
|
|
filepath = dirname.joinpath(basename + suffix)
|
|
|
|
if touch:
|
|
filepath.touch()
|
|
|
|
return filepath
|
|
|
|
|
|
@pytest.fixture
|
|
def paths() -> Generator[List[Path], None, None]:
|
|
random.seed(1234)
|
|
|
|
yield [random_path() for _ in range(20)]
|
|
|
|
|
|
@pytest.fixture
|
|
def presentation_config(paths: List[Path]) -> Generator[PresentationConfig, None, None]:
|
|
dirname = Path(tempfile.mkdtemp())
|
|
files = [random_path(dirname=dirname, touch=True) for _ in range(10)]
|
|
|
|
slides = [
|
|
SlideConfig(
|
|
type=SlideType.slide,
|
|
start_animation=0,
|
|
end_animation=5,
|
|
number=1,
|
|
),
|
|
SlideConfig(
|
|
type=SlideType.loop,
|
|
start_animation=5,
|
|
end_animation=6,
|
|
number=2,
|
|
),
|
|
SlideConfig(
|
|
type=SlideType.last,
|
|
start_animation=6,
|
|
end_animation=10,
|
|
number=3,
|
|
),
|
|
]
|
|
|
|
yield PresentationConfig(
|
|
slides=slides,
|
|
files=files,
|
|
)
|
|
|
|
|
|
def test_merge_basenames(paths: List[Path]) -> None:
|
|
path = merge_basenames(paths)
|
|
assert path.suffix == paths[0].suffix
|
|
assert path.parent == paths[0].parent
|
|
|
|
|
|
class TestKey:
|
|
@pytest.mark.parametrize(("ids", "name"), [([1], None), ([1], "some key name")])
|
|
def test_valid_keys(self, ids: Any, name: Any) -> None:
|
|
_ = Key(ids=ids, name=name)
|
|
|
|
@pytest.mark.parametrize(
|
|
("ids", "name"), [([], None), ([-1], None), ([1], {"an": " invalid name"})]
|
|
)
|
|
def test_invalid_keys(self, ids: Any, name: Any) -> None:
|
|
with pytest.raises(ValidationError):
|
|
_ = Key(ids=ids, name=name)
|
|
|
|
|
|
class TestPresentationConfig:
|
|
def test_validate(self, presentation_config: PresentationConfig) -> None:
|
|
obj = presentation_config.model_dump()
|
|
_ = PresentationConfig.model_validate(obj)
|
|
|
|
def test_bump_to_json(self, presentation_config: PresentationConfig) -> None:
|
|
_ = presentation_config.model_dump_json(indent=2)
|
|
|
|
def test_empty_presentation_config(self) -> None:
|
|
with pytest.raises(ValidationError):
|
|
_ = PresentationConfig(slides=[], files=[])
|