mirror of
https://github.com/jeertmans/manim-slides.git
synced 2025-05-18 03:05:21 +08:00
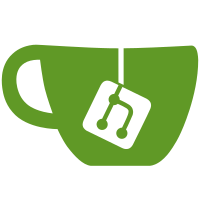
* chore(ci): test builds on multiple oses * [pre-commit.ci] auto fixes from pre-commit.com hooks for more information, see https://pre-commit.ci * fix: add tqdm to setup * chore(ci): test builds on multiple oses * [pre-commit.ci] auto fixes from pre-commit.com hooks for more information, see https://pre-commit.ci * fix: install deps in two steps * fix(bug): allows shape to be 2 or 3 dims * release new version * chore(ci): test builds on multiple oses * [pre-commit.ci] auto fixes from pre-commit.com hooks for more information, see https://pre-commit.ci * chore(ci): test builds on multiple oses * [pre-commit.ci] auto fixes from pre-commit.com hooks for more information, see https://pre-commit.ci * fix: install deps in two steps * fix: deps string * fix: ci * fix: single quote strings * fix: choco install manimce * Add pyopengl * Update test_examples.yml * fix: custom 3d example for manimgl * [pre-commit.ci] auto fixes from pre-commit.com hooks for more information, see https://pre-commit.ci * fix: virtual frame buffer * fix: add ffmpeg dep * feat(cli): add skip-all option for testing * chore(lint): black fmt * fix: typo in deps * fix: python -m pip install * fix: typo * Update test_examples.yml * fix: try fix * fix: pip install --user * pip install -e * only on windows * fix: tmp fix * fix: typo in int parsing * Update test_examples.yml * Update test_examples.yml * Update test_examples.yml * Update test_examples.yml * Update test_examples.yml * Update test_examples.yml * Update test_examples.yml * Update test_examples.yml * Update test_examples.yml * Update test_examples.yml * Update test_examples.yml * Update test_examples.yml * Update test_examples.yml * Update test_examples.yml * Update test_examples.yml * Update test_examples.yml * Update test_examples.yml * Update test_examples.yml * Update test_examples.yml * Update __version__.py Co-authored-by: pre-commit-ci[bot] <66853113+pre-commit-ci[bot]@users.noreply.github.com>
128 lines
3.6 KiB
Python
128 lines
3.6 KiB
Python
import sys
|
|
|
|
if "manim" in sys.modules:
|
|
from manim import *
|
|
|
|
MANIMGL = False
|
|
elif "manimlib" in sys.modules:
|
|
from manimlib import *
|
|
|
|
MANIMGL = True
|
|
else:
|
|
raise ImportError("This script must be run with either `manim` or `manimgl`")
|
|
|
|
from manim_slides import Slide, ThreeDSlide
|
|
|
|
|
|
class Example(Slide):
|
|
def construct(self):
|
|
circle = Circle(radius=3, color=BLUE)
|
|
dot = Dot()
|
|
|
|
self.play(GrowFromCenter(circle))
|
|
self.pause()
|
|
|
|
self.start_loop()
|
|
self.play(MoveAlongPath(dot, circle), run_time=2, rate_func=linear)
|
|
self.end_loop()
|
|
|
|
self.play(dot.animate.move_to(ORIGIN))
|
|
self.pause()
|
|
|
|
self.play(dot.animate.move_to(RIGHT * 3))
|
|
self.pause()
|
|
|
|
self.start_loop()
|
|
self.play(MoveAlongPath(dot, circle), run_time=2, rate_func=linear)
|
|
self.end_loop()
|
|
|
|
# Each slide MUST end with an animation (a self.wait is considered an animation)
|
|
self.play(dot.animate.move_to(ORIGIN))
|
|
|
|
|
|
# For ThreeDExample, things are different
|
|
|
|
if not MANIMGL:
|
|
|
|
class ThreeDExample(ThreeDSlide):
|
|
def construct(self):
|
|
axes = ThreeDAxes()
|
|
circle = Circle(radius=3, color=BLUE)
|
|
dot = Dot(color=RED)
|
|
|
|
self.add(axes)
|
|
|
|
self.set_camera_orientation(phi=75 * DEGREES, theta=30 * DEGREES)
|
|
|
|
self.play(GrowFromCenter(circle))
|
|
self.begin_ambient_camera_rotation(rate=75 * DEGREES / 4)
|
|
|
|
self.pause()
|
|
|
|
self.start_loop()
|
|
self.play(MoveAlongPath(dot, circle), run_time=4, rate_func=linear)
|
|
self.end_loop()
|
|
|
|
self.stop_ambient_camera_rotation()
|
|
self.move_camera(phi=75 * DEGREES, theta=30 * DEGREES)
|
|
|
|
self.play(dot.animate.move_to(ORIGIN))
|
|
self.pause()
|
|
|
|
self.play(dot.animate.move_to(RIGHT * 3))
|
|
self.pause()
|
|
|
|
self.start_loop()
|
|
self.play(MoveAlongPath(dot, circle), run_time=2, rate_func=linear)
|
|
self.end_loop()
|
|
|
|
# Each slide MUST end with an animation (a self.wait is considered an animation)
|
|
self.play(dot.animate.move_to(ORIGIN))
|
|
|
|
else:
|
|
# WARNING: 3b1b's manim change how ThreeDScene work,
|
|
# this is why things have to be managed differently.
|
|
class ThreeDExample(Slide):
|
|
CONFIG = {
|
|
"camera_class": ThreeDCamera,
|
|
}
|
|
|
|
def construct(self):
|
|
axes = ThreeDAxes()
|
|
circle = Circle(radius=3, color=BLUE)
|
|
dot = Dot(color=RED)
|
|
|
|
self.add(axes)
|
|
|
|
frame = self.camera.frame
|
|
frame.set_euler_angles(
|
|
theta=30 * DEGREES,
|
|
phi=75 * DEGREES,
|
|
gamma=0,
|
|
)
|
|
|
|
self.play(GrowFromCenter(circle))
|
|
updater = lambda m, dt: m.increment_theta((75 * DEGREES / 4) * dt)
|
|
frame.add_updater(updater)
|
|
|
|
self.pause()
|
|
|
|
self.start_loop()
|
|
self.play(MoveAlongPath(dot, circle), run_time=4, rate_func=linear)
|
|
self.end_loop()
|
|
|
|
frame.remove_updater(updater)
|
|
self.play(frame.animate.set_theta(30 * DEGREES))
|
|
self.play(dot.animate.move_to(ORIGIN))
|
|
self.pause()
|
|
|
|
self.play(dot.animate.move_to(RIGHT * 3))
|
|
self.pause()
|
|
|
|
self.start_loop()
|
|
self.play(MoveAlongPath(dot, circle), run_time=2, rate_func=linear)
|
|
self.end_loop()
|
|
|
|
# Each slide MUST end with an animation (a self.wait is considered an animation)
|
|
self.play(dot.animate.move_to(ORIGIN))
|