diff --git a/.github/workflows/languagetool.yml b/.github/workflows/languagetool.yml
new file mode 100644
index 0000000..2e79b60
--- /dev/null
+++ b/.github/workflows/languagetool.yml
@@ -0,0 +1,15 @@
+on:
+ pull_request:
+ workflow_dispatch:
+
+name: LanguageTool check
+
+jobs:
+ languagetool_check:
+ runs-on: ubuntu-latest
+ steps:
+ - uses: actions/checkout@v1
+ - uses: reviewdog/action-languagetool@v1
+ with:
+ reporter: github-pr-review
+ level: warning
diff --git a/README.md b/README.md
index 93981d4..70ff790 100644
--- a/README.md
+++ b/README.md
@@ -5,31 +5,66 @@

# Manim Slides
-Tool for live presentations using either [manim-community](https://www.manim.community/) or [manimgl](https://3b1b.github.io/manim/). `manim-slides` will automatically detect the one you are using!
+Tool for live presentations using either [Manim (community edition)](https://www.manim.community/) or [ManimGL](https://3b1b.github.io/manim/). Manim Slides will *automatically* detect the one you are using!
> **_NOTE:_** This project extends the work of [`manim-presentation`](https://github.com/galatolofederico/manim-presentation), with a lot more features!
-## Install
+- [Install](#install)
+ * [Dependencies](#dependencies)
+ * [Pip install](#pip-install)
+ * [Install From Repository](#install-from-repository)
+- [Usage](#usage)
+ * [Basic Example](#basic-example)
+ * [Key Bindings](#key-bindings)
+ * [Other Examples](#other-examples)
+- [Features and Comparison with Original manim-presentation](#features-and-comparison-with-original-manim-presentation)
+- [Contributing](#contributing)
-```
+## Installation
+
+While installing Manim Slides and its dependencies on your global Python is fine, I recommend using a [virtualenv](https://docs.python.org/3/tutorial/venv.html) for a local installation.
+
+### Dependencies
+
+Manim Slides requires either Manim or ManimGL to be installed. Having both packages installed is fine too.
+
+If none of those packages are installed, please refer to their specifc installation guidelines:
+- [Manim](https://docs.manim.community/en/stable/installation.html)
+- [ManimGL](https://3b1b.github.io/manim/getting_started/installation.html)
+
+### Pip Install
+
+The recommended way to install the latest release is to use pip:
+
+```bash
pip install manim-slides
```
-## Usage
+### Install From Repository
-Use the class `Slide` as your scenes base class:
-```python
-from manim_slides import Slide
+An alternative way to install Manim Slides is to clone the git repository, and install from there:
-class Example(Slide):
- def construct(self):
- ...
+```bash
+git clone https://github.com/jeertmans/manim-slides
+pip install -e .
```
-call `self.pause()` when you want to pause the playback and wait for an input to continue (check the keybindings).
+> *Note:* the `-e` flag allows you to edit the files, and observe the changes directly when using Manim Slides
+
+## Usage
+
+Using Manim Slides is a two-step process:
+1. Render animations using `Slide` (resp. `ThreeDSlide`) as a base class instead of `Scene` (resp. `ThreeDScene`), and add calls to `self.pause()` everytime you want to create a new slide.
+2. Run `manim-slides` on rendered animations and display them like a *Power Point* presentation.
+
+### Basic Example
+
Wrap a series of animations between `self.start_loop()` and `self.stop_loop()` when you want to loop them (until input to continue):
+
```python
+# example.py
+
from manim import *
# or: from manimlib import *
from manim_slides import Slide
@@ -40,105 +75,103 @@ class Example(Slide):
dot = Dot()
self.play(GrowFromCenter(circle))
- self.pause()
+ self.pause() # Waits user to press continue to go to the next slide
- self.start_loop()
+ self.start_loop() # Start loop
self.play(MoveAlongPath(dot, circle), run_time=2, rate_func=linear)
- self.end_loop()
+ self.end_loop() # This will loop until user inputs a key
self.play(dot.animate.move_to(ORIGIN))
- self.pause()
+ self.pause() # Waits user to press continue to go to the next slide
- self.wait()
+ self.wait() # The presentation directly exits after last animation
```
-You **must** end your `Slide` with a `self.play(...)` or a `self.wait(..)`.
+You **must** end your `Slide` with a `self.play(...)` or a `self.wait(...)`.
+
+First, render the animation files:
+
+```bash
+manim example.py
+# or
+manimgl example.py
+```
To start the presentation using `Scene1`, `Scene2` and so on simply run:
-```
-manim-slides Scene1 Scene2...
+
+```bash
+manim-slides [OPTIONS] Scene1 Scene2...
```
-## Keybindings
-
-Default keybindings to control the presentation:
-
-| Keybinding | Action |
-|:-----------:|:------------------------:|
-| Right Arrow | Continue/Next Slide |
-| Left Arrow | Previous Slide |
-| R | Re-Animate Current Slide |
-| V | Reverse Current Slide |
-| Spacebar | Play/Pause |
-| Q | Quit |
-
-
-You can run the **configuration wizard** with:
+Or in this example:
+```bash
+manim-slides Example
```
+
+## Key Bindings
+
+The default key bindings to control the presentation are:
+
+| Keybinding | Action | Icon |
+|:-----------:|:------------------------:|:----:|
+| Right Arrow | Continue/Next Slide | |
+| Left Arrow | Previous Slide | |
+| R | Replay Current Slide | |
+| V | Reverse Current Slide | |
+| Spacebar | Play/Pause | |
+| Q | Quit | |
+
+You can run the **configuration wizard** to change those key bindings:
+
+```bash
manim-slides wizard
```
-Alternatively you can specify different keybindings creating a file named `.manim-slides.json` with the keys: `QUIT` `CONTINUE` `BACK` `REVERSE` `REWIND` and `PLAY_PAUSE`.
+Alternatively you can specify different key bindings creating a file named `.manim-slides.json` with the keys: `QUIT` `CONTINUE` `BACK` `REVERSE` `REWIND` and `PLAY_PAUSE`.
A default file can be created with:
-```
+
+```bash
manim-slides init
```
> **_NOTE:_** `manim-slides` uses `cv2.waitKeyEx()` to wait for keypresses, and directly registers the key code.
-## Run Example
+## Other Examples
-Clone this repository:
-```
-git clone https://github.com/jeertmans/manim-slides.git
-cd manim-slides
-```
-
-Install `manim` and `manim-slides`:
-```
-pip install manim manim-slides
-# or
-pip install manimgl manim-slides
-```
-
-Render the example scene:
-```
-manim -qh example.py Example
-# or
-manimgl --hd example.py Example
-```
-
-Run the presentation
-```
-manim-slides Example
-```
+Other examples are available in the `example.py` file, if you downloaded the git repository.
Below is a small recording of me playing with the slides back and forth.
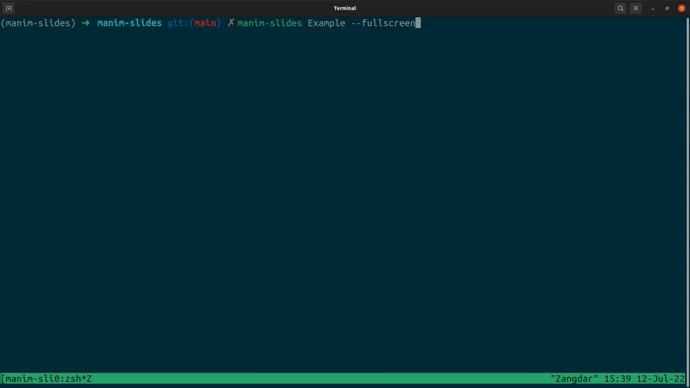
-## Comparison with original `manim-presentation`
+## Features and Comparison with original manim-presentation
-Here are a few things that I implemented (or that I'm planning to implement) on top of the original work:
+Below is a non-exhaustive list of features:
-- [x] Allowing multiple keys to control one action (useful when you use a laser pointer)
-- [x] More robust config files checking
-- [x] Dependencies are installed with the package
-- [x] Only one cli (to rule them all)
-- [x] User can easily generate dummy config file
-- [x] Config file path can be manually set
-- [x] Play animation in reverse [#9](https://github.com/galatolofederico/manim-presentation/issues/9)
-- [x] Handle 3D scenes out of the box
-- [x] Support for both `manim` and `manimgl` modules
-- [ ] Generate docs online
-- [x] Fix the quality problem on Windows platforms with `fullscreen` flag
+| Feature | `manim-slides` | `manim-presentation` |
+|:--------|:--------------:|:--------------------:|
+| Support for Manim | :heavy_check_mark: | :heavy_check_mark: |
+| Support for ManimGL | :heavy_check_mark: | :heavy_multiplication_x: |
+| Configurable key bindings | :heavy_check_mark: | :heavy_check_mark: |
+| Configurable paths | :heavy_check_mark: | :heavy_multiplication_x: |
+| Play / Pause slides | :heavy_check_mark: | :heavy_check_mark: |
+| Next / Previous slide | :heavy_check_mark: | :heavy_check_mark: |
+| Replay slide | :heavy_check_mark: | :heavy_check_mark: |
+| Reverse slide | :heavy_check_mark: | :heavy_multiplication_x: |
+| Multiple key per actions | :heavy_check_mark: | :heavy_multiplication_x: |
+| One command line tool | :heavy_check_mark: | :heavy_multiplication_x: |
+| Robust config file parsing | :heavy_check_mark: | :heavy_multiplication_x: |
+| Support for 3D Scenes | :heavy_check_mark: | :heavy_multiplication_x: |
+| Documented code | WIP | :heavy_multiplication_x: |
+| Tested on Unix, macOS, and Windows | :heavy_check_mark: | :heavy_multiplication_x: |
-## Contributions and license
-The code is released as Free Software under the [GNU/GPLv3](https://choosealicense.com/licenses/gpl-3.0/) license. Copying, adapting and republishing it is not only consent but also encouraged.
+## Contributing
+
+Contributions are more than welcome!
[pypi-version-badge]: https://img.shields.io/pypi/v/manim-slides?label=manim-slides
[pypi-version-url]: https://pypi.org/project/manim-slides/