mirror of
https://github.com/filecoin-project/lotus.git
synced 2025-05-21 00:58:27 +08:00
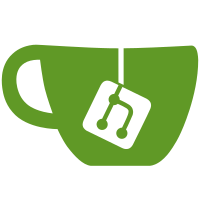
Introduce the first API for Lotus v2, focusing on `ChainGetTipSet` within the `Chain` group. Define `TipSetSelector` for advanced tipset retrieval options, and create a compact JSON-RPC call format. Gracefully accommodate both EC and F3 finalized tipsets based on node configuration, where: * EC finalized tipset is returned when F3 is turned off, has no finalized tipset or F3 isn't ready. * F3 finalized is returned otherwise. Support three categories of selectors under `TipSetSelector`: * By tag: either "latest" or "finalized." * By height: epoch, plus optional fallback to previous non-null tipset. * By tipset key. The selection falls back to tag "latest" if the user specifies no selection criterion. The JSON-RPC format is designed to use JSON Object as the parameters passed to the RPC call to remain compact, and extensible.
106 lines
3.2 KiB
Go
106 lines
3.2 KiB
Go
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
|
|
"golang.org/x/sync/errgroup"
|
|
|
|
"github.com/filecoin-project/lotus/api/docgen"
|
|
docgen_openrpc "github.com/filecoin-project/lotus/api/docgen-openrpc"
|
|
)
|
|
|
|
func main() {
|
|
var lets errgroup.Group
|
|
lets.SetLimit(1) // TODO: Investigate why this can't run in parallel.
|
|
lets.Go(generateApiFull)
|
|
lets.Go(generateApiV0Methods)
|
|
lets.Go(generateApiV2Methods)
|
|
lets.Go(generateStorage)
|
|
lets.Go(generateWorker)
|
|
lets.Go(generateOpenRpcGateway)
|
|
if err := lets.Wait(); err != nil {
|
|
fmt.Println("Error:", err)
|
|
os.Exit(1)
|
|
}
|
|
fmt.Println("All documentations generated successfully.")
|
|
}
|
|
|
|
func generateWorker() error {
|
|
if ainfo, err := docgen.ParseApiASTInfo("api/api_worker.go", "Worker", "api", "./api"); err != nil {
|
|
return err
|
|
} else if err := generateMarkdown("documentation/en/api-v0-methods-worker.md", "Worker", "api", ainfo); err != nil {
|
|
return err
|
|
} else {
|
|
return generateOpenRpc("build/openrpc/worker.json", "Worker", "api", ainfo)
|
|
}
|
|
}
|
|
|
|
func generateStorage() error {
|
|
if ainfo, err := docgen.ParseApiASTInfo("api/api_storage.go", "StorageMiner", "api", "./api"); err != nil {
|
|
return err
|
|
} else if err := generateMarkdown("documentation/en/api-v0-methods-miner.md", "StorageMiner", "api", ainfo); err != nil {
|
|
return err
|
|
} else {
|
|
return generateOpenRpc("build/openrpc/miner.json", "StorageMiner", "api", ainfo)
|
|
}
|
|
}
|
|
|
|
func generateApiV0Methods() error {
|
|
if ainfo, err := docgen.ParseApiASTInfo("api/v0api/full.go", "FullNode", "v0api", "./api/v0api"); err != nil {
|
|
return err
|
|
} else {
|
|
return generateMarkdown("documentation/en/api-v0-methods.md", "FullNode", "v0api", ainfo)
|
|
}
|
|
}
|
|
|
|
func generateApiV2Methods() error {
|
|
if ainfo, err := docgen.ParseApiASTInfo("api/v2api/full.go", "FullNode", "v2api", "./api/v2api"); err != nil {
|
|
return err
|
|
} else if err := generateMarkdown("documentation/en/api-v2-unstable-methods.md", "FullNode", "v2api", ainfo); err != nil {
|
|
return err
|
|
} else {
|
|
return generateOpenRpc("build/openrpc/v2/full.json", "FullNode", "v2api", ainfo)
|
|
}
|
|
}
|
|
|
|
func generateApiFull() error {
|
|
if ainfo, err := docgen.ParseApiASTInfo("api/api_full.go", "FullNode", "api", "./api"); err != nil {
|
|
return err
|
|
} else if err := generateMarkdown("documentation/en/api-v1-unstable-methods.md", "FullNode", "api", ainfo); err != nil {
|
|
return err
|
|
} else {
|
|
return generateOpenRpc("build/openrpc/full.json", "FullNode", "api", ainfo)
|
|
}
|
|
}
|
|
|
|
func generateOpenRpcGateway() error {
|
|
if ainfo, err := docgen.ParseApiASTInfo("api/api_gateway.go", "Gateway", "api", "./api"); err != nil {
|
|
return err
|
|
} else {
|
|
return generateOpenRpc("build/openrpc/gateway.json", "Gateway", "api", ainfo)
|
|
}
|
|
}
|
|
|
|
func generateMarkdown(path, iface, pkg string, info docgen.ApiASTInfo) error {
|
|
out, err := os.OpenFile(path, os.O_WRONLY|os.O_CREATE|os.O_TRUNC, 0644)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
defer func() {
|
|
_ = out.Close()
|
|
}()
|
|
return docgen.Generate(out, iface, pkg, info)
|
|
}
|
|
|
|
func generateOpenRpc(path, iface, pkg string, info docgen.ApiASTInfo) error {
|
|
out, err := os.OpenFile(path, os.O_WRONLY|os.O_CREATE|os.O_TRUNC, 0644)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
defer func() {
|
|
_ = out.Close()
|
|
}()
|
|
return docgen_openrpc.Generate(out, iface, pkg, info, false)
|
|
}
|