mirror of
https://github.com/filecoin-project/lotus.git
synced 2025-05-17 15:20:37 +08:00
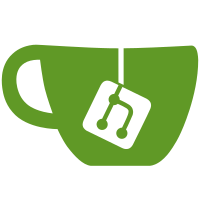
* feat(f3): allow specifying full static manifests Signed-off-by: Jakub Sztandera <oss@kubuxu.com> * fix interop ManifestServerID Signed-off-by: Jakub Sztandera <oss@kubuxu.com> * cleanup F3BoostrapEpoch Signed-off-by: Jakub Sztandera <oss@kubuxu.com> * fix butterfly Signed-off-by: Jakub Sztandera <oss@kubuxu.com> * Rip out dynamic manifest handling Signed-off-by: Jakub Sztandera <oss@kubuxu.com> * Fix itests Signed-off-by: Jakub Sztandera <oss@kubuxu.com> * fix lint Signed-off-by: Jakub Sztandera <oss@kubuxu.com> * Disable non-relevant linter Signed-off-by: Jakub Sztandera <oss@kubuxu.com> * fix tests Signed-off-by: Jakub Sztandera <oss@kubuxu.com> * Address review Signed-off-by: Jakub Sztandera <oss@kubuxu.com> * add CHANGELOG Signed-off-by: Jakub Sztandera <oss@kubuxu.com> * Update build/buildconstants/f3manifest_butterfly.json Co-authored-by: Masih H. Derkani <m@derkani.org> * Update build/buildconstants/f3manifest_interop.json Co-authored-by: Masih H. Derkani <m@derkani.org> * Update build/buildconstants/f3manifest_2k.json Co-authored-by: Masih H. Derkani <m@derkani.org> --------- Signed-off-by: Jakub Sztandera <oss@kubuxu.com> Co-authored-by: Masih H. Derkani <m@derkani.org>
88 lines
2.2 KiB
Go
88 lines
2.2 KiB
Go
package buildconstants
|
|
|
|
import (
|
|
"encoding/json"
|
|
"math/big"
|
|
"os"
|
|
"time"
|
|
|
|
"github.com/ipfs/go-cid"
|
|
logging "github.com/ipfs/go-log/v2"
|
|
"github.com/libp2p/go-libp2p/core/peer"
|
|
|
|
"github.com/filecoin-project/go-address"
|
|
"github.com/filecoin-project/go-f3/manifest"
|
|
"github.com/filecoin-project/go-state-types/abi"
|
|
|
|
"github.com/filecoin-project/lotus/chain/actors/policy"
|
|
)
|
|
|
|
// moved from now-defunct build/paramfetch.go
|
|
var log = logging.Logger("build/buildtypes")
|
|
|
|
func SetAddressNetwork(n address.Network) {
|
|
address.CurrentNetwork = n
|
|
}
|
|
|
|
func MustParseAddress(addr string) address.Address {
|
|
ret, err := address.NewFromString(addr)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
|
|
return ret
|
|
}
|
|
|
|
func IsNearUpgrade(epoch, upgradeEpoch abi.ChainEpoch) bool {
|
|
if upgradeEpoch < 0 {
|
|
return false
|
|
}
|
|
return epoch > upgradeEpoch-policy.ChainFinality && epoch < upgradeEpoch+policy.ChainFinality
|
|
}
|
|
|
|
func MustParseID(id string) peer.ID {
|
|
p, err := peer.Decode(id)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
return p
|
|
}
|
|
|
|
func wholeFIL(whole uint64) *big.Int {
|
|
bigWhole := big.NewInt(int64(whole))
|
|
return bigWhole.Mul(bigWhole, big.NewInt(int64(FilecoinPrecision)))
|
|
}
|
|
|
|
func F3Manifest() *manifest.Manifest {
|
|
if F3ManifestBytes == nil {
|
|
return nil
|
|
}
|
|
var manif manifest.Manifest
|
|
|
|
if err := json.Unmarshal(F3ManifestBytes, &manif); err != nil {
|
|
log.Panicf("failed to unmarshal F3 manifest: %s", err)
|
|
}
|
|
if err := manif.Validate(); err != nil {
|
|
log.Panicf("invalid F3 manifest: %s", err)
|
|
}
|
|
|
|
if ptCid := os.Getenv("F3_INITIAL_POWERTABLE_CID"); ptCid != "" {
|
|
if k, err := cid.Parse(ptCid); err != nil {
|
|
log.Errorf("failed to parse F3_INITIAL_POWERTABLE_CID %q: %s", ptCid, err)
|
|
} else if manif.InitialPowerTable.Defined() && k != manif.InitialPowerTable {
|
|
log.Errorf("ignoring F3_INITIAL_POWERTABLE_CID as lotus has a hard-coded initial F3 power table")
|
|
} else {
|
|
manif.InitialPowerTable = k
|
|
}
|
|
}
|
|
if !manif.InitialPowerTable.Defined() {
|
|
log.Warn("initial power table is not specified, it will be populated automatically assuming this is testing network")
|
|
}
|
|
|
|
// EC Period sanity check
|
|
if manif.EC.Period != time.Duration(BlockDelaySecs)*time.Second {
|
|
log.Panicf("static manifest EC period is %v, expected %v", manif.EC.Period, time.Duration(BlockDelaySecs)*time.Second)
|
|
}
|
|
return &manif
|
|
}
|