mirror of
https://github.com/facebook/lexical.git
synced 2025-05-21 00:57:23 +08:00
Add controller shared context (#852)
This commit is contained in:
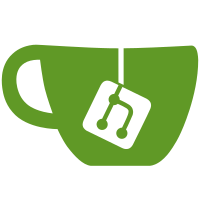
committed by
acywatson
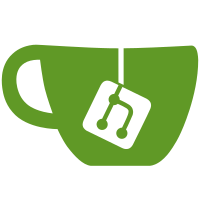
parent
e2a3787e06
commit
ca7cb2b4d7
@ -22,6 +22,8 @@ export type PlaygroundContext = {
|
|||||||
triggerListeners(type: 'readonly' | 'clear', value: ListenerValue): void,
|
triggerListeners(type: 'readonly' | 'clear', value: ListenerValue): void,
|
||||||
};
|
};
|
||||||
|
|
||||||
|
export type PlaygroundSharedContext = {};
|
||||||
|
|
||||||
const config = {
|
const config = {
|
||||||
theme: {
|
theme: {
|
||||||
paragraph: 'editor-paragraph',
|
paragraph: 'editor-paragraph',
|
||||||
@ -90,7 +92,17 @@ function createPlaygroundContext(): PlaygroundContext {
|
|||||||
};
|
};
|
||||||
}
|
}
|
||||||
|
|
||||||
const PlaygroundController: Controller<PlaygroundContext> =
|
function createPlaygroundSharedContext(): PlaygroundSharedContext {
|
||||||
createController<PlaygroundContext>(createPlaygroundContext, config);
|
return {};
|
||||||
|
}
|
||||||
|
|
||||||
|
const PlaygroundController: Controller<
|
||||||
|
PlaygroundContext,
|
||||||
|
PlaygroundSharedContext,
|
||||||
|
> = createController<PlaygroundContext, PlaygroundSharedContext>(
|
||||||
|
createPlaygroundContext,
|
||||||
|
createPlaygroundSharedContext,
|
||||||
|
config,
|
||||||
|
);
|
||||||
|
|
||||||
export default PlaygroundController;
|
export default PlaygroundController;
|
||||||
|
@ -10,56 +10,60 @@
|
|||||||
import type {OutlineEditor, EditorState, EditorThemeClasses} from 'outline';
|
import type {OutlineEditor, EditorState, EditorThemeClasses} from 'outline';
|
||||||
|
|
||||||
import * as React from 'react';
|
import * as React from 'react';
|
||||||
import {createContext, useContext, useMemo} from 'react';
|
import {createContext as createReactContext, useContext, useMemo} from 'react';
|
||||||
import {createEditor} from 'outline';
|
import {createEditor} from 'outline';
|
||||||
import invariant from 'shared/invariant';
|
import invariant from 'shared/invariant';
|
||||||
|
|
||||||
type ControllerContext<Context> = null | [OutlineEditor, Context];
|
type ControllerContext<Context, SharedContext> =
|
||||||
|
| null
|
||||||
|
| [OutlineEditor, Context, null | SharedContext];
|
||||||
|
|
||||||
export type Controller<Context> = {
|
export type Controller<Context, SharedContext> = {
|
||||||
({children: React$Node}): React$Node,
|
({children: React$Node}): React$Node,
|
||||||
__context: React$Context<ControllerContext<Context>>,
|
__context: React$Context<ControllerContext<Context, SharedContext>>,
|
||||||
};
|
};
|
||||||
|
|
||||||
export function createController<Context>(
|
export function createController<Context, SharedContext>(
|
||||||
createControllerContext: () => Context,
|
createContext: () => Context,
|
||||||
|
createSharedContext: () => SharedContext,
|
||||||
editorConfig?: {
|
editorConfig?: {
|
||||||
initialEditorState?: EditorState,
|
initialEditorState?: EditorState,
|
||||||
theme?: EditorThemeClasses,
|
theme?: EditorThemeClasses,
|
||||||
},
|
},
|
||||||
): Controller<Context> {
|
): Controller<Context, SharedContext> {
|
||||||
const ControlledContext = createContext<ControllerContext<Context>>(null);
|
const ControllerContextInternal =
|
||||||
|
createReactContext<ControllerContext<Context, SharedContext>>(null);
|
||||||
|
|
||||||
function ControllerComponent({children}) {
|
function ControllerComponent({children}) {
|
||||||
const existingContext = useContext(ControlledContext);
|
const existingController = useContext(ControllerContextInternal);
|
||||||
if (existingContext !== null) {
|
|
||||||
invariant(
|
|
||||||
false,
|
|
||||||
'OutlineController\'s of the same type cannot be nested',
|
|
||||||
);
|
|
||||||
}
|
|
||||||
const controllerContext = useMemo(() => {
|
const controllerContext = useMemo(() => {
|
||||||
const context: Context = createControllerContext();
|
const context: Context = createContext();
|
||||||
const editor = createEditor<Context>({
|
const editor = createEditor<Context>({
|
||||||
...editorConfig,
|
...editorConfig,
|
||||||
context,
|
context,
|
||||||
});
|
});
|
||||||
return [editor, context];
|
let sharedContextValue: SharedContext | null = null;
|
||||||
}, []);
|
// Create shared context
|
||||||
|
if (existingController === null) {
|
||||||
|
sharedContextValue = createSharedContext();
|
||||||
|
}
|
||||||
|
return [editor, context, sharedContextValue];
|
||||||
|
}, [existingController]);
|
||||||
|
|
||||||
return (
|
return (
|
||||||
<ControlledContext.Provider value={controllerContext}>
|
<ControllerContextInternal.Provider value={controllerContext}>
|
||||||
{children}
|
{children}
|
||||||
</ControlledContext.Provider>
|
</ControllerContextInternal.Provider>
|
||||||
);
|
);
|
||||||
}
|
}
|
||||||
ControllerComponent.__context = ControlledContext;
|
ControllerComponent.__context = ControllerContextInternal;
|
||||||
|
|
||||||
return ControllerComponent;
|
return ControllerComponent;
|
||||||
}
|
}
|
||||||
|
|
||||||
export function useController<Context>(
|
export function useController<Context, SharedContext>(
|
||||||
controller: Controller<Context>,
|
controller: Controller<Context, SharedContext>,
|
||||||
): [OutlineEditor, Context] {
|
): [OutlineEditor, Context, null | SharedContext] {
|
||||||
const context = useContext(controller.__context);
|
const context = useContext(controller.__context);
|
||||||
if (context === null) {
|
if (context === null) {
|
||||||
invariant(
|
invariant(
|
||||||
|
@ -43,5 +43,6 @@
|
|||||||
"41": "createNode: node does not exist in nodeMap",
|
"41": "createNode: node does not exist in nodeMap",
|
||||||
"42": "reconcileNode: prevNode or nextNode does not exist in nodeMap",
|
"42": "reconcileNode: prevNode or nextNode does not exist in nodeMap",
|
||||||
"43": "reconcileNode: parentDOM is null",
|
"43": "reconcileNode: parentDOM is null",
|
||||||
"44": "Reconciliation: could not find DOM element for node key \"${key}\""
|
"44": "Reconciliation: could not find DOM element for node key \"${key}\"",
|
||||||
|
"45": "OutlineCotext.useOutlineContext: cannot find matching OutlineContext"
|
||||||
}
|
}
|
||||||
|
Reference in New Issue
Block a user