mirror of
https://github.com/ipfs/kubo.git
synced 2025-05-21 00:47:22 +08:00
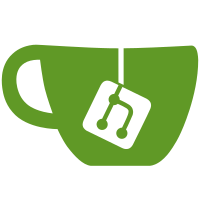
* add deprecation warning when tracer plugins are loaded * add response format attribute to span in gateway handler * add note about tracing's experimental status in godoc * add nil check for TTL when adding name span attrs * add basic sharness test for integration with otel collector * add nil check in UnixFSAPI.processLink * test: sharness check all json objs for swarm span * add env var docs to docs/environment-variables.md * chore: pin the otel collector version * add tracing spans per response type (#8841) * docs: tracing with jaeger-ui Co-authored-by: Marcin Rataj <lidel@lidel.org>
48 lines
1.6 KiB
Go
48 lines
1.6 KiB
Go
package corehttp
|
|
|
|
import (
|
|
"bytes"
|
|
"io/ioutil"
|
|
"net/http"
|
|
"time"
|
|
|
|
"github.com/ipfs/go-ipfs/tracing"
|
|
ipath "github.com/ipfs/interface-go-ipfs-core/path"
|
|
"go.opentelemetry.io/otel/attribute"
|
|
"go.opentelemetry.io/otel/trace"
|
|
)
|
|
|
|
// serveRawBlock returns bytes behind a raw block
|
|
func (i *gatewayHandler) serveRawBlock(w http.ResponseWriter, r *http.Request, resolvedPath ipath.Resolved, contentPath ipath.Path, begin time.Time) {
|
|
ctx, span := tracing.Span(r.Context(), "Gateway", "ServeRawBlock", trace.WithAttributes(attribute.String("path", resolvedPath.String())))
|
|
defer span.End()
|
|
blockCid := resolvedPath.Cid()
|
|
blockReader, err := i.api.Block().Get(ctx, resolvedPath)
|
|
if err != nil {
|
|
webError(w, "ipfs block get "+blockCid.String(), err, http.StatusInternalServerError)
|
|
return
|
|
}
|
|
block, err := ioutil.ReadAll(blockReader)
|
|
if err != nil {
|
|
webError(w, "ipfs block get "+blockCid.String(), err, http.StatusInternalServerError)
|
|
return
|
|
}
|
|
content := bytes.NewReader(block)
|
|
|
|
// Set Content-Disposition
|
|
name := blockCid.String() + ".bin"
|
|
setContentDispositionHeader(w, name, "attachment")
|
|
|
|
// Set remaining headers
|
|
modtime := addCacheControlHeaders(w, r, contentPath, blockCid)
|
|
w.Header().Set("Content-Type", "application/vnd.ipld.raw")
|
|
w.Header().Set("X-Content-Type-Options", "nosniff") // no funny business in the browsers :^)
|
|
|
|
// Done: http.ServeContent will take care of
|
|
// If-None-Match+Etag, Content-Length and range requests
|
|
http.ServeContent(w, r, name, modtime, content)
|
|
|
|
// Update metrics
|
|
i.rawBlockGetMetric.WithLabelValues(contentPath.Namespace()).Observe(time.Since(begin).Seconds())
|
|
}
|