mirror of
https://github.com/ipfs/kubo.git
synced 2025-05-19 07:56:01 +08:00
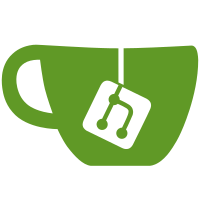
Also change existing 'Node' type to 'ProtoNode' and use that most everywhere for now. As we move forward with the integration we will try and use the Node interface in more places that we're currently using ProtoNode. License: MIT Signed-off-by: Jeromy <why@ipfs.io>
94 lines
2.1 KiB
Go
94 lines
2.1 KiB
Go
package coreunix
|
|
|
|
import (
|
|
"bytes"
|
|
"io/ioutil"
|
|
"testing"
|
|
|
|
bstore "github.com/ipfs/go-ipfs/blocks/blockstore"
|
|
bserv "github.com/ipfs/go-ipfs/blockservice"
|
|
core "github.com/ipfs/go-ipfs/core"
|
|
offline "github.com/ipfs/go-ipfs/exchange/offline"
|
|
importer "github.com/ipfs/go-ipfs/importer"
|
|
chunk "github.com/ipfs/go-ipfs/importer/chunk"
|
|
merkledag "github.com/ipfs/go-ipfs/merkledag"
|
|
ft "github.com/ipfs/go-ipfs/unixfs"
|
|
uio "github.com/ipfs/go-ipfs/unixfs/io"
|
|
|
|
context "context"
|
|
cid "gx/ipfs/QmXUuRadqDq5BuFWzVU6VuKaSjTcNm1gNCtLvvP1TJCW4z/go-cid"
|
|
u "gx/ipfs/Qmb912gdngC1UWwTkhuW8knyRbcWeu5kqkxBpveLmW8bSr/go-ipfs-util"
|
|
ds "gx/ipfs/QmbzuUusHqaLLoNTDEVLcSF6vZDHZDLPC7p4bztRvvkXxU/go-datastore"
|
|
dssync "gx/ipfs/QmbzuUusHqaLLoNTDEVLcSF6vZDHZDLPC7p4bztRvvkXxU/go-datastore/sync"
|
|
)
|
|
|
|
func getDagserv(t *testing.T) merkledag.DAGService {
|
|
db := dssync.MutexWrap(ds.NewMapDatastore())
|
|
bs := bstore.NewBlockstore(db)
|
|
blockserv := bserv.New(bs, offline.Exchange(bs))
|
|
return merkledag.NewDAGService(blockserv)
|
|
}
|
|
|
|
func TestMetadata(t *testing.T) {
|
|
ctx := context.Background()
|
|
// Make some random node
|
|
ds := getDagserv(t)
|
|
data := make([]byte, 1000)
|
|
u.NewTimeSeededRand().Read(data)
|
|
r := bytes.NewReader(data)
|
|
nd, err := importer.BuildDagFromReader(ds, chunk.DefaultSplitter(r))
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
c := nd.Cid()
|
|
|
|
m := new(ft.Metadata)
|
|
m.MimeType = "THIS IS A TEST"
|
|
|
|
// Such effort, many compromise
|
|
ipfsnode := &core.IpfsNode{DAG: ds}
|
|
|
|
mdk, err := AddMetadataTo(ipfsnode, c.String(), m)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
rec, err := Metadata(ipfsnode, mdk)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if rec.MimeType != m.MimeType {
|
|
t.Fatalf("something went wrong in conversion: '%s' != '%s'", rec.MimeType, m.MimeType)
|
|
}
|
|
|
|
cdk, err := cid.Decode(mdk)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
retnode, err := ds.Get(ctx, cdk)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
rtnpb, ok := retnode.(*merkledag.ProtoNode)
|
|
if !ok {
|
|
t.Fatal("expected protobuf node")
|
|
}
|
|
|
|
ndr, err := uio.NewDagReader(ctx, rtnpb, ds)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
out, err := ioutil.ReadAll(ndr)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
if !bytes.Equal(out, data) {
|
|
t.Fatal("read incorrect data")
|
|
}
|
|
}
|